Difference Between var, let And const Keyword In JavaScript
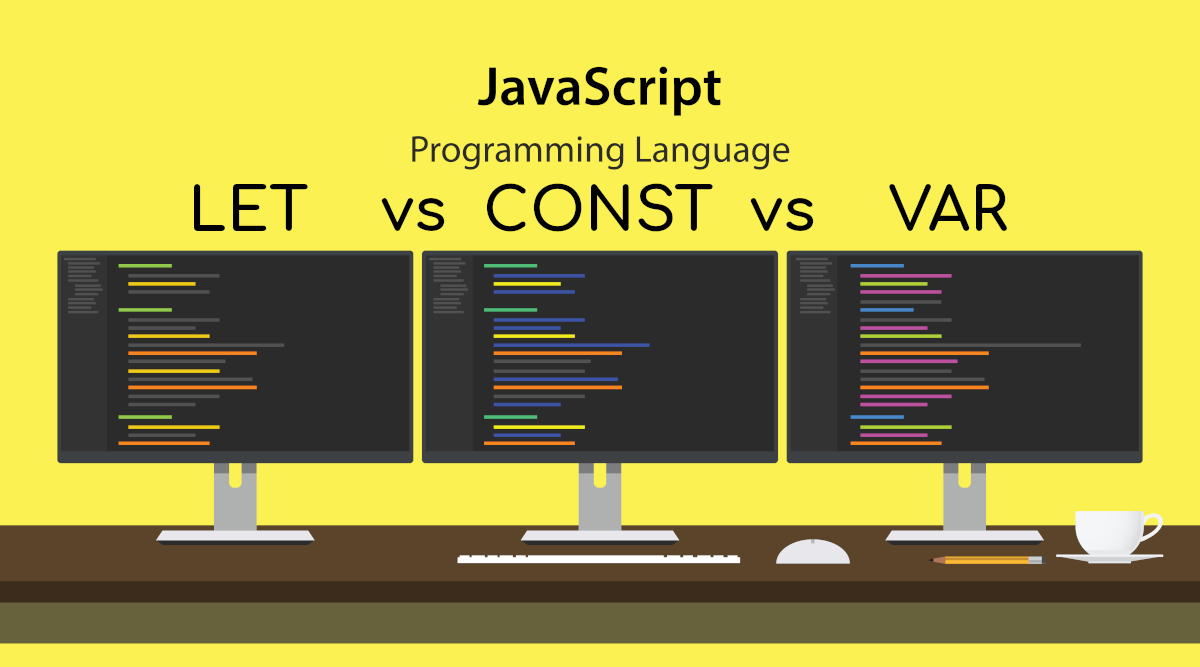
JavaScript Es6 has introduced two keywords: let and const for declaring your variable. Before these keywords, var was used for variable declaration. In this post, we will see what are the basic differences between these three ways of variable declaration. The main difference between these three keywords is based on a common concept known as scope i.e. where the variable will be avaialable.
var is function scoped whereas let and const are block scoped. That means to say that the variable declared with var is avaialable anywhere inside the function where it is defined. And the same is true for the variables declared using let and const. They are available only inside the block where they are defined. Consider the following code:
<script>
function demo()
{
var name1 = "John";
console.log(name1);
}
demo();
// It will print John in the console.
console.log(name1);
// Uncaught ReferenceError: name1 is not defined
</script>
In line number 9 of this code i.e. console.log(name);
, we are trying to access the name variable outside the function demo. Since, name is declared with var keyword, its scope is only limited inside the function demo and not outside it. Here's the output on console:
Now, lets see about let and const.
<script>
if(true)
{
let name = "John";
const surname = "Murray";
}
console.log(name);
// Uncaught ReferenceError: name is not defined
console.log(surname);
// Uncaught ReferenceError: surname is not defined
</script>
Here name and surname are available only inside the if-block. So if you want to get the output then you have to move that console statement inside the if-block and you can see the output in console without any error.
Another major difference between var and let/const is that variable declared using var keyword can be redeclared again with different value whereas the same can't be applied for let/const. Consider the following code:
<script>
var name1 = "hello";
console.log(name1);
var name1= "Bruce";
console.log(name1);
</script>
You can see the output as below in your console.
Now if you replace that var keyword with let or const then you will get the syntax error in your console. The corresponding code with let keyword is:
<script>
let name1 = "hello";
console.log(name1);
let name1= "Bruce";
console.log(name1);
</script>
The output in the console is:
The same is true if you replace that let keyword with const keyword.
Now we have seen a major difference between var, let and const. It looks like const/let is pretty different from var in the similar way. But what are the difference between let and const? Well, the only major difference between these two keyword is that variable declared with let can be updated but not redeclared (as we saw above: SyntaxError) whereas the variable declared using const can neither be redeclared nor be updated since const is used to imply constant value i.e. can't change forever once declared. Lets see with the example and code for let keyword.
<script>
let name1 = "Edward";
console.log(name1);
name1 = "Oscar";
console.log(name1);
</script>
We can see the updated value gets printed in the console as below:
Now lets change the above code by replacing the let keyword with const as below:
<script>
const name1 = "Edward";
console.log(name1);
name1 = "Oscar";
console.log(name1);
</script>
We can see the Edward gets printed since there is no error upto the second line. The third line throws an error since we are trying to update the value. The error is as following in the console:
I hope this makes clear about the difference between var and let/const. If there's something that I had left out please comment down below. I am enthusiastic to learn more.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.