Return Type In JavaScript Function
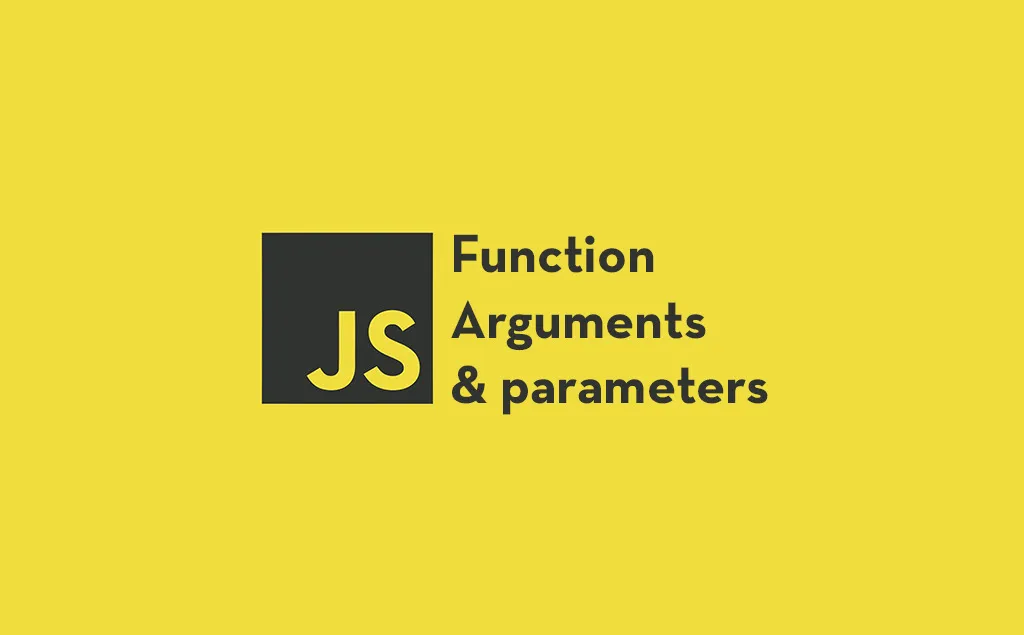
The keyword return is used to return the value as a result of function execution to the calling function. Tpically the value is returned at the end of the function. As soon as the control reaches the point where return statement is used, the functions stops its operation and any code after that will be ignored. We will see a simple example of function that will return the reverse the string passed to the function parameter. For this we will use JavaScript built-in function: split()
that will split the string into the array of individual characters, reverse()
that will reverse the array of characters that we just got from previous steps and then finally use join()
to join all these individual characters into a single string.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Function</title>
<script>
function findReverse(a)
{
var splitted_string = a.split('');
var reverse_character = splitted_string.reverse();
var reverse_string = reverse_character.join('');
return reverse_string;
}
var result = findReverse("TPIRCSAVAJ");
document.write("<h1>" + "The reverse string is: " + result + "</h1>")
</script>
</head>
<body>
</body>
</html>
When you use a return statement, you should store the return value of the calling function in some variable (in above case it is the variable result). If you run the code and see the output, you can see it as below: