JavaScript Objects: Second Part
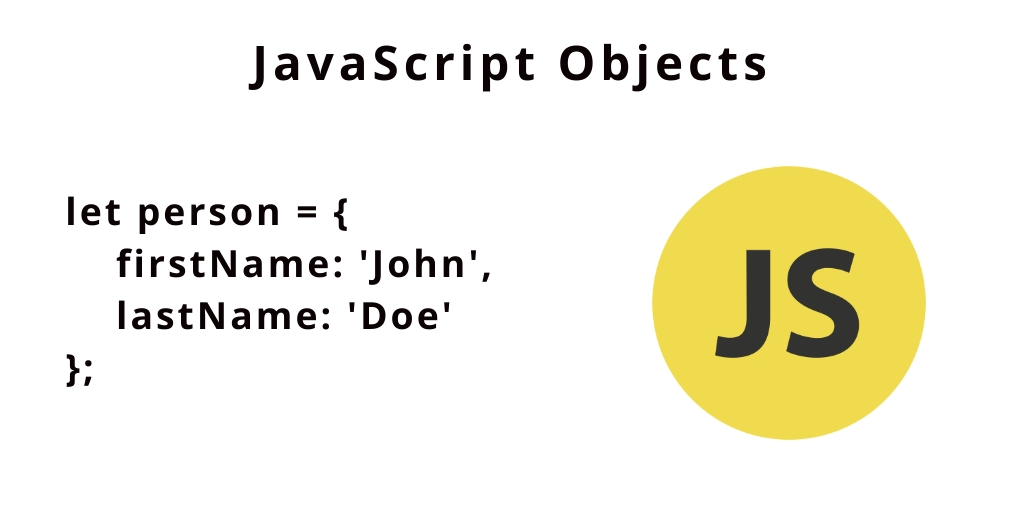
In my previous post which can be found here, I discuss about the basic concepts behind JavaScript objects and how to create an object by using a literal way. In this post, I am gonna discuss about the new way you can use to create objects in JavaScript. If you have seen the last post, you can notice that creating object through a literal way is not very desirable when creating a large number of objects. It will take more space and makes your code long and not so attractive. So, we will be using a concept of object constructor to create our object. And this is done with the help of our constructor function which is a template for creating an object.
<!DOCTYPE html>
<html lang="en">
<head>
<title>JS Object</title>
<script>
function Bike(brand, model, price, mileage, speed)
{
this.brand = brand;
this.model = model;
this.price = price;
this.mileage = mileage;
this.speed = speed;
this.run = function()
{
return "The bike is now running.";
}
}
var bike1 = new Bike("Apache", "RTR 200", 35000, "40 km/l", "127 km/hr");
var bike2 = new Bike("Bajaj", "Pulsar 200 NS", 380000, "42 km/l", "130 km/hr");
console.log(bike1.mileage);
console.log(bike2.run())
</script>
</head>
<body>
</body>
</html>
In the above code function Bike() is our constructor function. You can see the same output as you see for the previous one:
Another method of creating an object in JS is through the use of Object.create(). The first argument to the function is the prototype for the object. In the simplest term, it means an existing object which a new object can use as a prototype. A simplest example is:
<script>
var Bike1 = {
Brand: "Apache",
Model: "RTR 200",
Price: 350000,
Mileage: "40 km/l",
Speed: "127 km/hr",
run: function()
{
return "The bike is now running.";
}
}
var Bike2 = Object.create(Bike1);
Bike2.Brand = "Bajaj";
Bike2.Model = "Pulsar 200 NS";
Bike2.Price = 380000;
Bike2.Mileage = "42 km/l";
Bike2.Speed = "130 km/hr";
Bike2.run = function(){return "The bike is now running.";}
console.log(Bike2.run());
</script>
You will get the output that the bike is running in the console.