Function In JavaScript
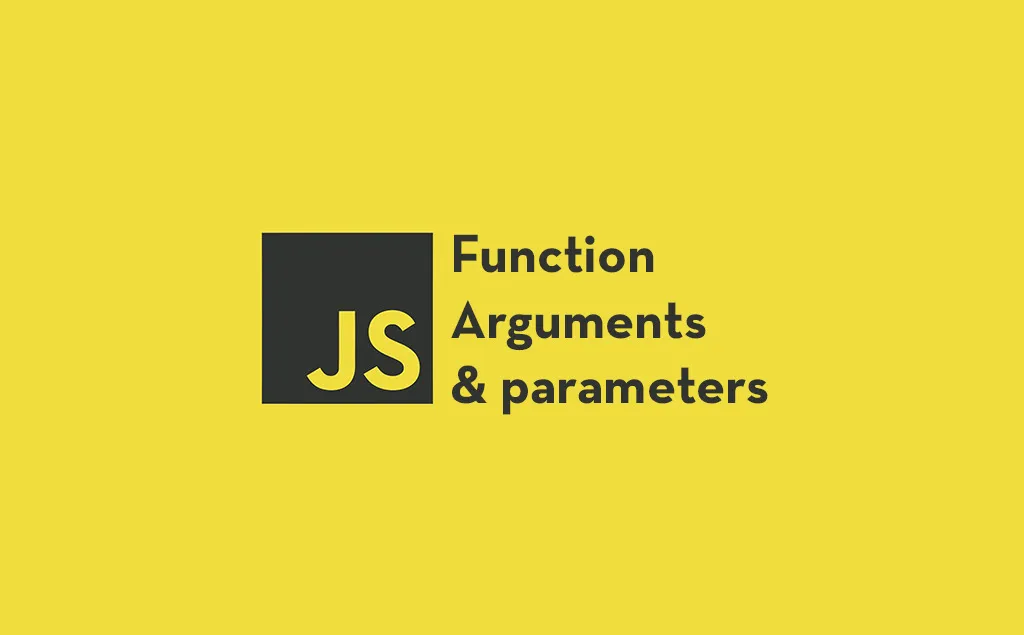
Functions are key concepts in every programming language which is nothing but just a block that consists of a piece of code that can be reused in multiple places across your program by calling it. It is used to avoid code duplication and makes your code clear, concise and effective. For example: window.alert()
, document.write()
-these all are built-in functions in JavaScript that you can use manytimes in your programs.
Function can have parameters or no parameters to perform its intended task. The basic syntax of JavaScript function is:
function func_name()
{
//Function Body
}
func_name()
The function is defined using function keyword followed by the function name. There are certain conventions while you are writing function name. Its good to write the name starting with lowercase followed by uppercase like: findSum or the one separated with underscore like: find_sum. The name should also be descriptive not just random word so that other programmer also know actually what
the function does especially when the project is very big. If there are multiple function parameters, they are written inside the parenthesis separated by comma.
There are many advanced stuffs you can do with JavaScript functions but I am just writing about its basic details so that you can grasp understanding of it at very core level. We will be doing more complex programming as we progress (as I am also learnign right now 😁😃). Lets first see a simple program that has no any parameters. We will just print a simple message on the screen.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Function</title>
<script>
function showMessage()
{
document.write("<h1>This is function with no parameter.</h1>")
}
showMessage();
</script>
</head>
<body>
</body>
</html>
You need to call function in order to actually run the function, which I did in the above code just after the end of curly braces. We see the following output on the browser:
Now we will see a program that has a function with one parameter defined in the function name. We will pass string as an argument to that function and prints its upper case.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Function</title>
<script>
function upperCase(a)
{
document.write("<h1>" + "The word in uppercase is " + a.toUpperCase() + "</h1>");
}
upperCase("hello");
</script>
</head>
<body>
</body>
</html>
Here "a" is function parameter and "hello" is function argument. You can see the following output in the browser screen: