Python & Hive: How start easy
I love Hive and Python :)
I love it when machines work instead of me ...
I don't like it when I miss something...
And of course, I absolutely love free.
So this post is about making my hive experiences free and helping me.
The first thing that prevents you from learning Python is the presence of a server on which it is installed and ready to work around the clock.
But the problem is solved with the help of replit.com registration in which is free and is performed in a couple of clicks
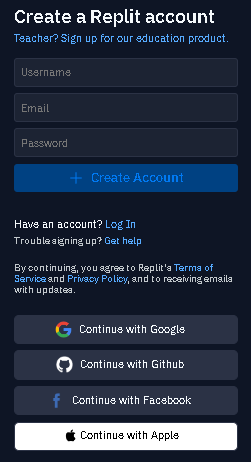
Undoubtedly, those who are more attentive will be surprised to say - "What's the point if there is no Always On Repls mode in the free plan"
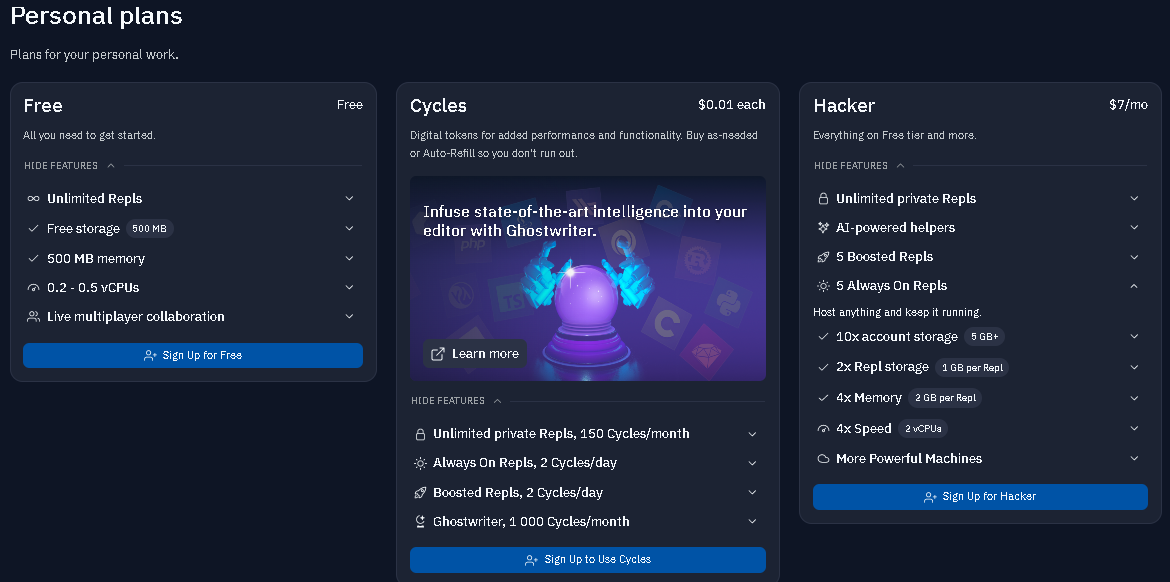
And they will be right. If we close the browser, after 10-30 minutes our script will stop. But if "someone" periodically opens a link leading to the page of our web server, the scripts will continue to work indefinitely.
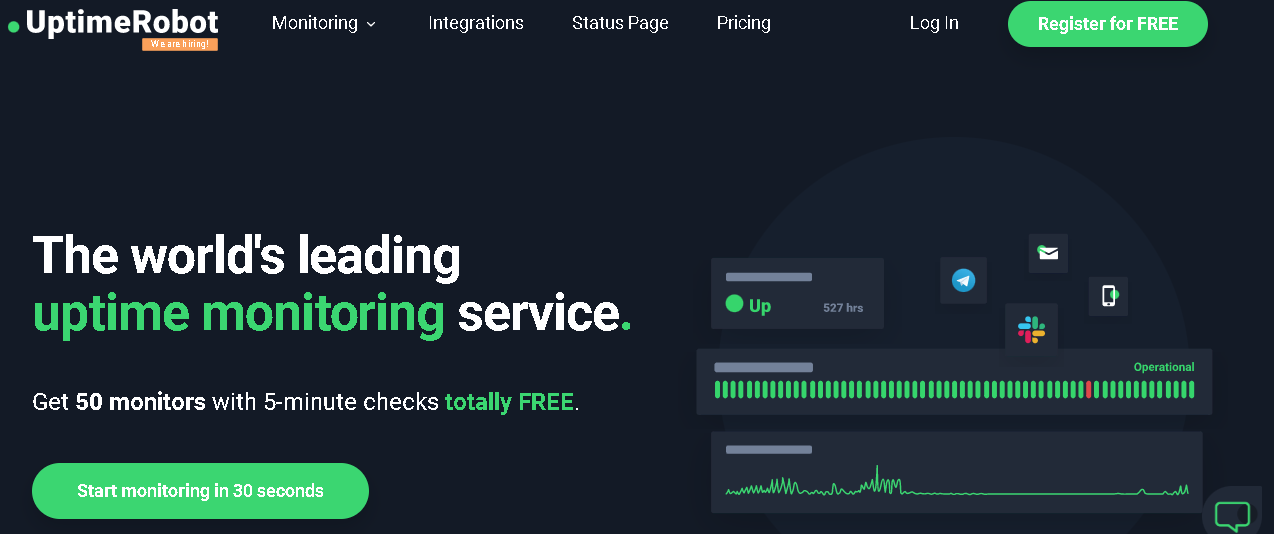
For example, the uptimerobot.com service will open it every five minutes for free.
Disclamation: Don't forget, this is a public service, so be safe.
I prefer to receive telegram notifications. (But if anyone is interested in how to make my plan on Discord, I will make a separate post) so I will describe how to make a simple telegram bot.
To do this, you need to follow a few simple steps.
Contact the bot https://t.me/BotFather
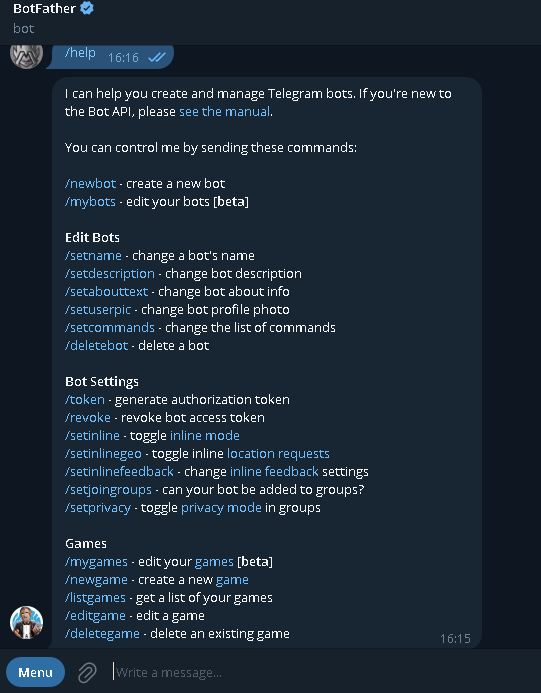
Give the command to create a new bot
/newbot
And we answer questions
Choose a name for the bot (I specified NameMyBotForTestPyHive)
And the username, which for bots must end with _bot (I specified NameMyBotForTestPyHive_bot)
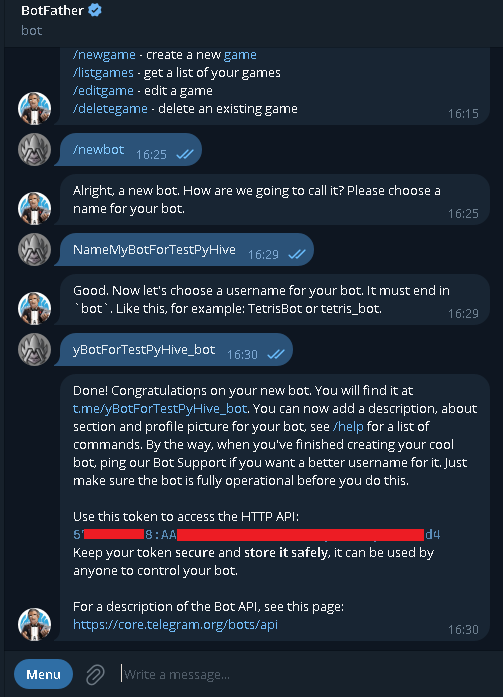
As a result, we received a token to access the HTTP API
It makes sense that I removed it. Anyone who knows the token can control your bot.
For convenience, I created a channel in which my bot will report the events I need
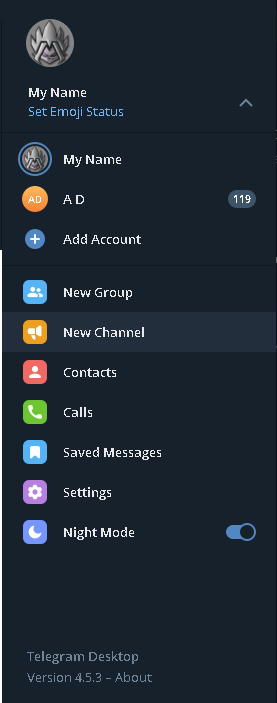
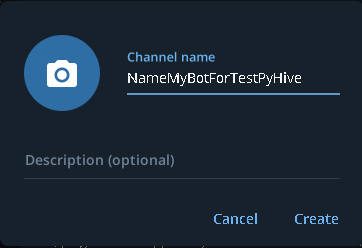
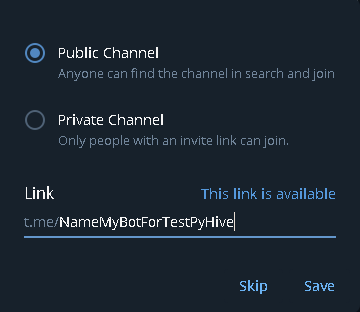
Public or private channel - it's your choice. It does not affect work in any way.
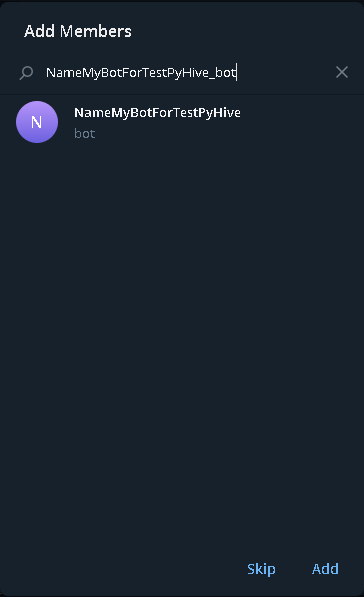
And immediately add our bot to the channel.
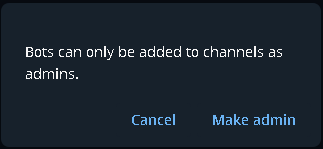
Make him an admin
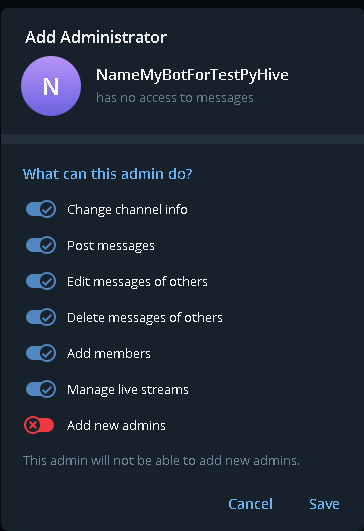
In general, it is enough for us that the bot has the right to send messages to the channel, but I left everything by default
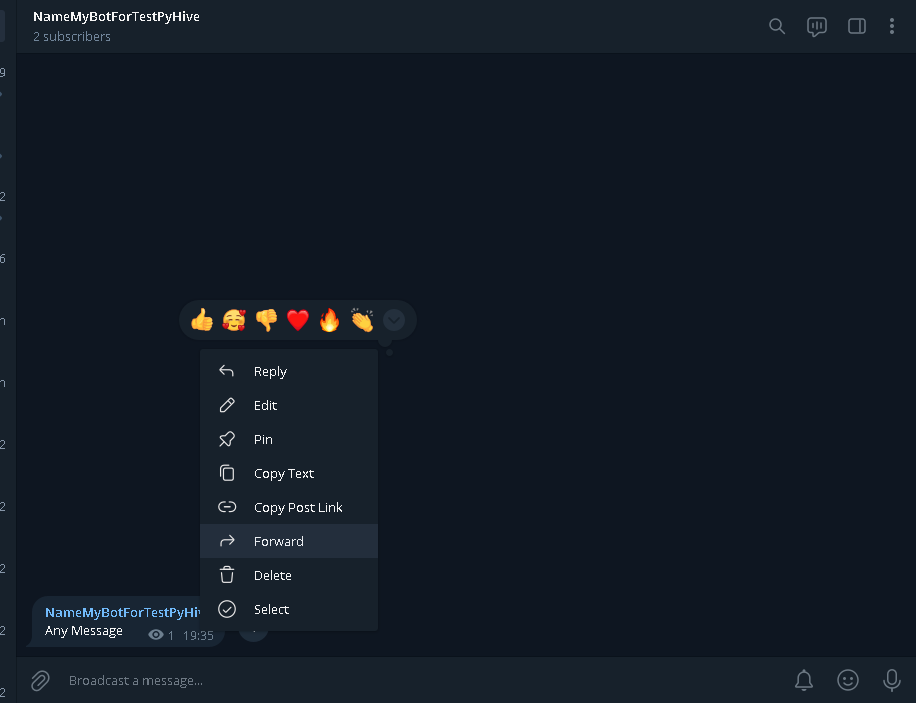
We need to find out the channel id to which the bot will send messages.
To do this, we will write any message in the channel and send it to the bot https://t.me/getidsbot
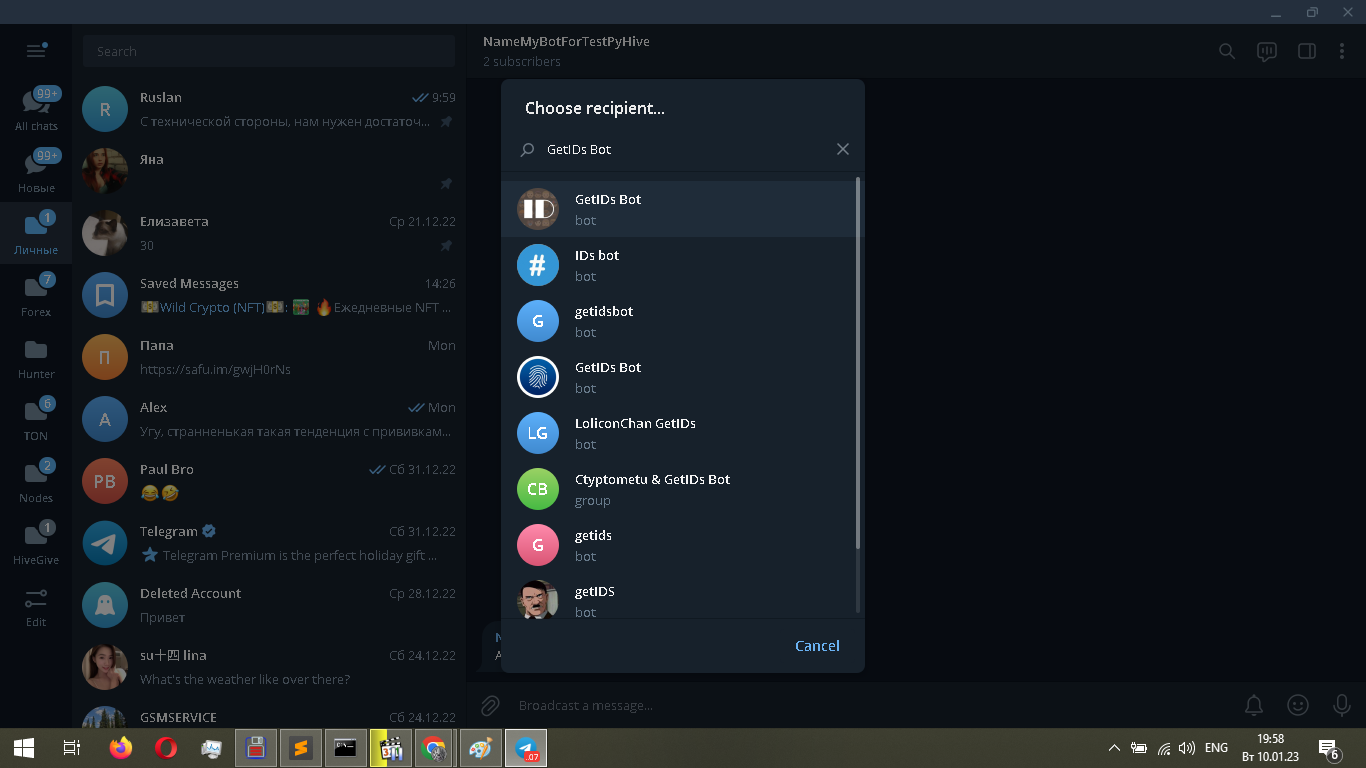
In response, we will receive a message with information about us, about the channel and about the message.
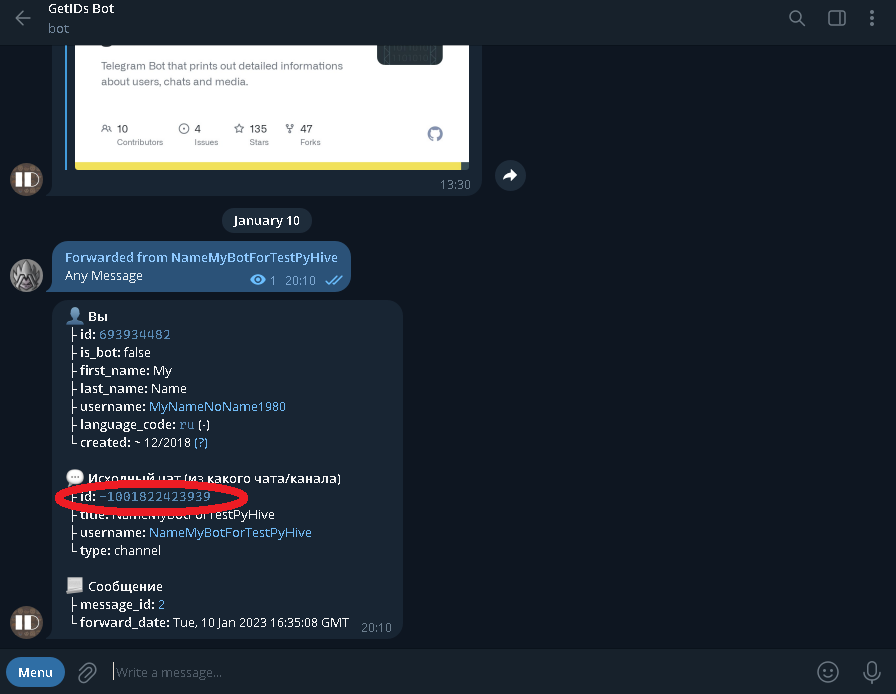
IMPORTANT The channel id starts with a minus sign and it is important
Before working with Telegram’s API, you need to get your own API ID and hash:
- Login to your Telegram account with the phone number of the developer account to use.
- Click under API Development tools.
- A Create new application window will appear. Fill in your application details. There is no need to enter any URL, and only the first two fields (App title and Short name) can currently be changed later.
- Click on Create application at the end. Remember that your API hash is secret and Telegram won’t let you revoke it. Don’t post it anywhere!
Note
This API ID and hash is the one used by your application, not your phone number. You can use this API ID and hash with any phone number or even for bot accounts.
(c) Telethon Docs
Setup phase completed
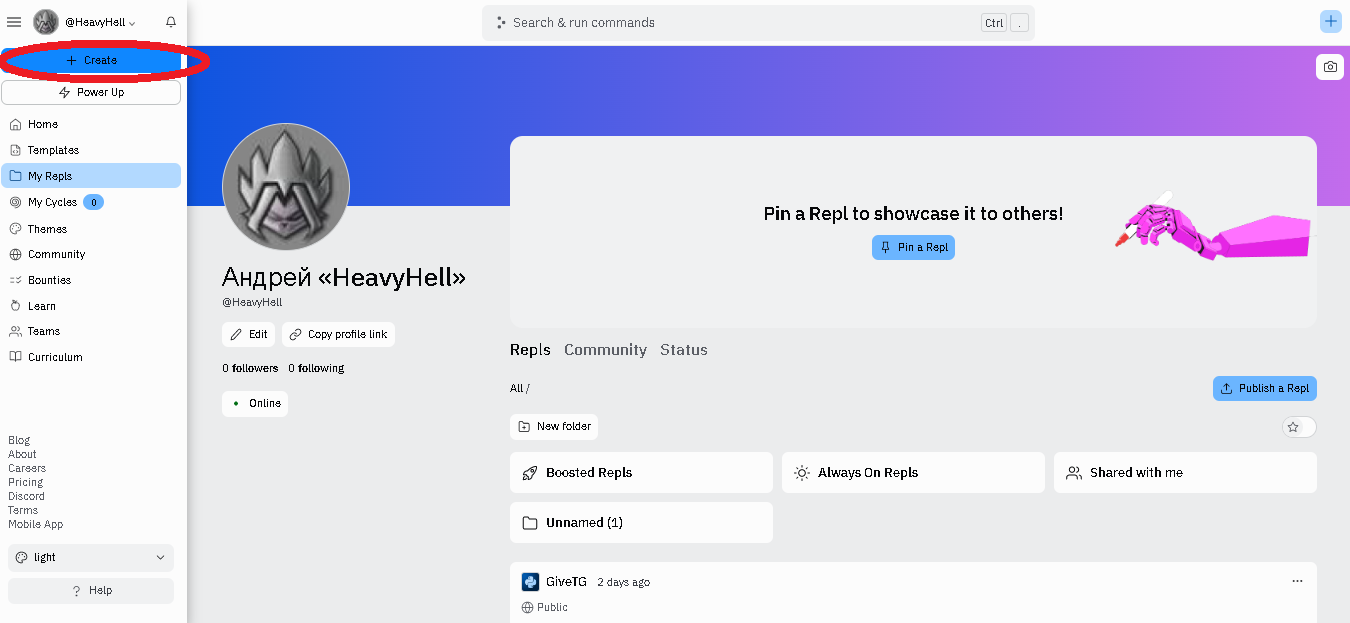
And select the Python template (although in some cases it is more convenient to use Python with Turtle or Python Discord Bot with pre-installed libraries)
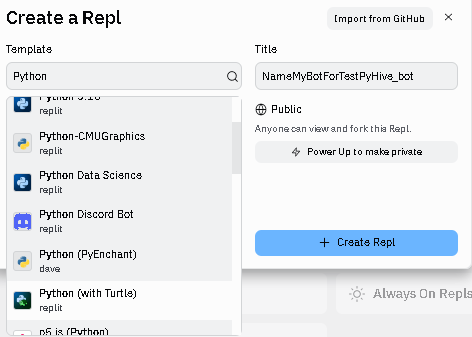
Enter the name of our replit and click create
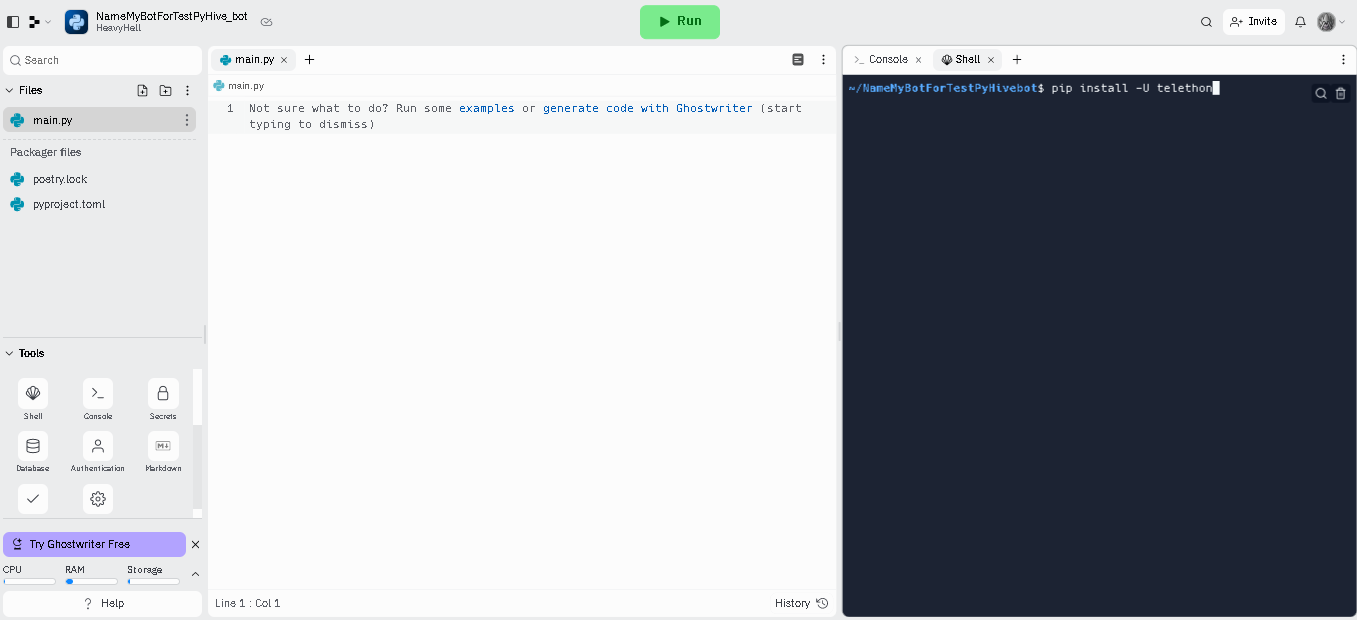
In the console (shell tab), install the necessary libraries.
telethon - for working with telegrams
beem - for working with the hive
pip install -U telethon
pip install -U beem
I prefer to keep the settings in a separate file, so I create a config.py file
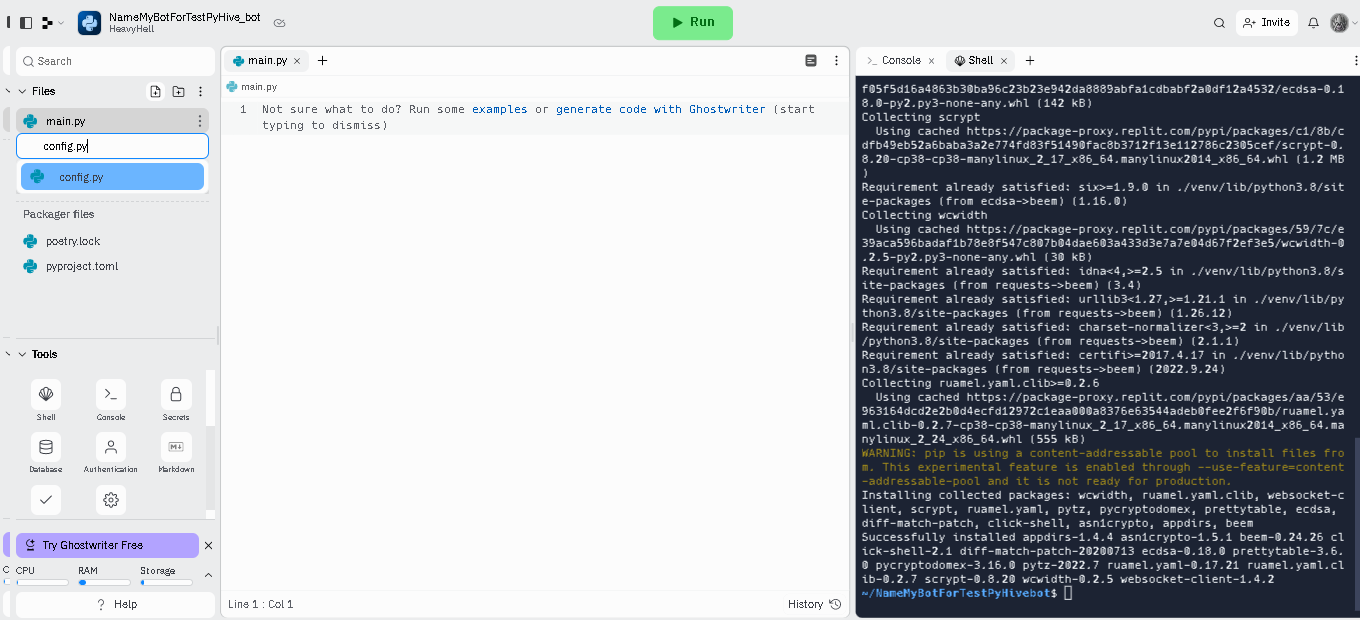
Since we use a public service and anyone can view the source code, we cannot enter our APIs directly in the code. But of course there is a way out. We will use environment variables.
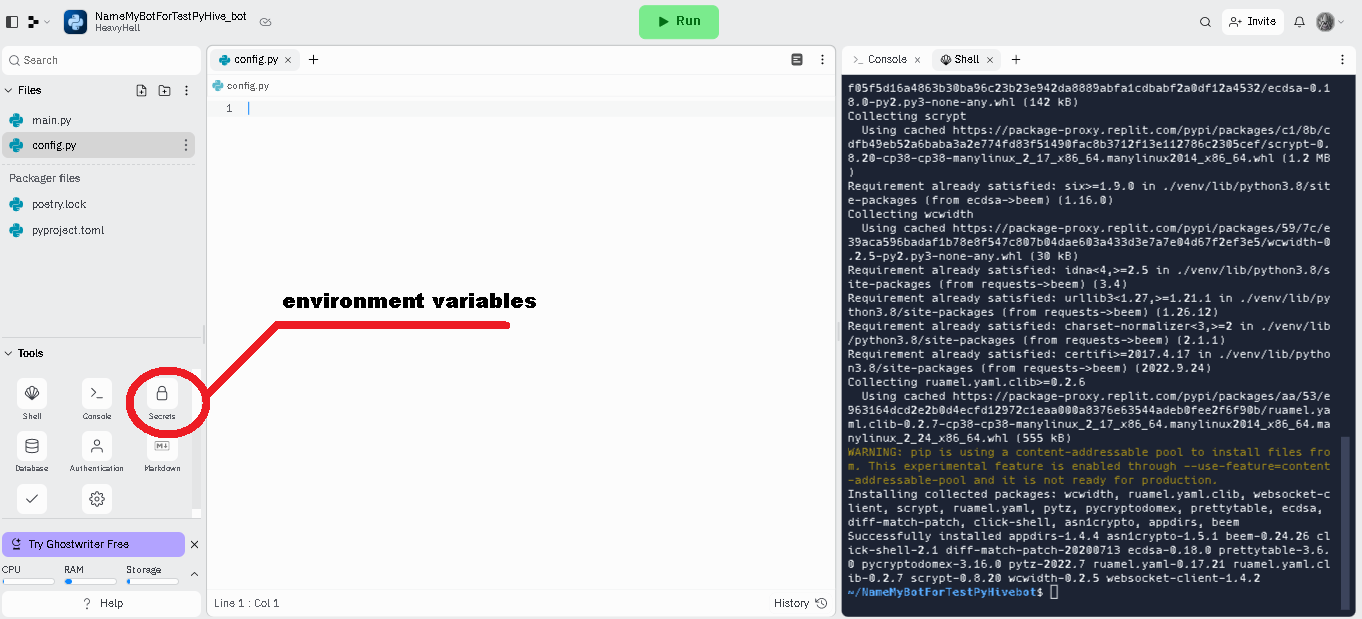
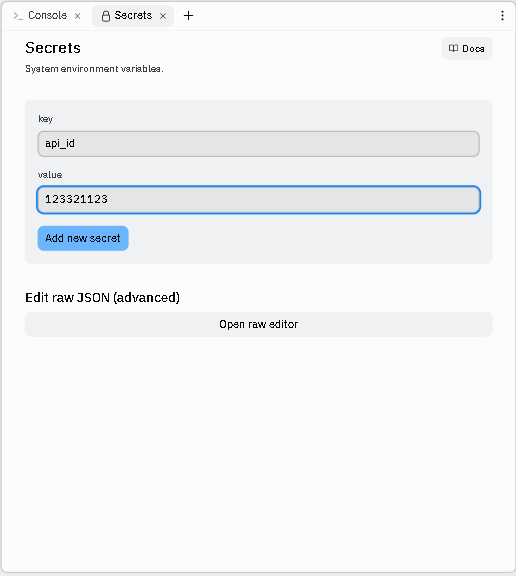
In raw editor it looks something like this.
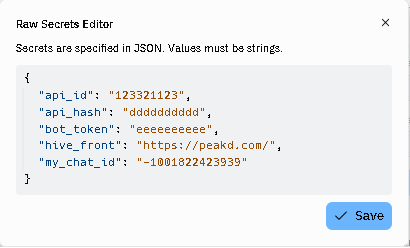
That is, we set five environment variables
Create another background.py file in the project. Flask server will be launched in it, which will receive requests from the monitoring service and be used to maintain the script on ReplIt.
from flask import Flask
from flask import request
from threading import Thread
import time
import requests
app = Flask('')
@app.route('/')
def home():
return "I'm alive"
def run():
app.run(host='0.0.0.0', port=80)
def keep_alive():
t = Thread(target=run)
t.start()
This will be our page, which uptimerobot.com will check every five minutes.
In main.py we add our bot
# -*- coding: utf-8 -*-
from config import *
from beem import Hive
from beem.blockchain import Blockchain
from beem.nodelist import NodeList
from telethon.sync import TelegramClient
from telethon.sessions import MemorySession
from telethon.errors.rpcerrorlist import PeerFloodError, SessionPasswordNeededError
from background import keep_alive
nodelist = NodeList()
nodelist.update_nodes()
nodes = nodelist.get_hive_nodes()
hive = Hive(node=nodes)
print(hive.is_hive)
blockchain = Blockchain()
start_num = blockchain.get_current_block_num()
// checklist - A list of words that we are looking for in post titles (case is not important in check_pattern_func, we still translate it to lower)
checklist = {'Giveaway','giveaway','GIVEAWAY'}
// We store the session in memory (by default, a file is created, which is not suitable for us due to the publicity of the service)
bot = TelegramClient(MemorySession(), api_id, api_hash)
bot.start(bot_token=bot_token)
// Function to send a message to a channel
def send_message_func(text):
bot.send_message(entity=my_chat_id, parse_mode='html', link_preview=False, message=text)
// Function to search for the occurrence of a word in a string
def check_pattern_func(text,key_words):
words = text.lower().split()
for word in words:
for key in key_words:
if key in word:
return True
return False
send_message_func('Start from ' + str(start_num) + ' block' )
// Starting the Flask Server
keep_alive()
// Listen to the blockchain. When we hear a comment appear
for op in blockchain.stream(opNames=['comment']):
// Check if it's a reply to someone's comment or if it's a new post
if op['parent_author'] == '':
// If this is a new post, check if the word from the checklist is in the title
if check_pattern_func(op['title'],checklist):
// Form a link to the post
mess = hive_front+"@"+op['author']+"/"+op['permlink']
// Send it to the channel
send_message_func(mess)
Running our script
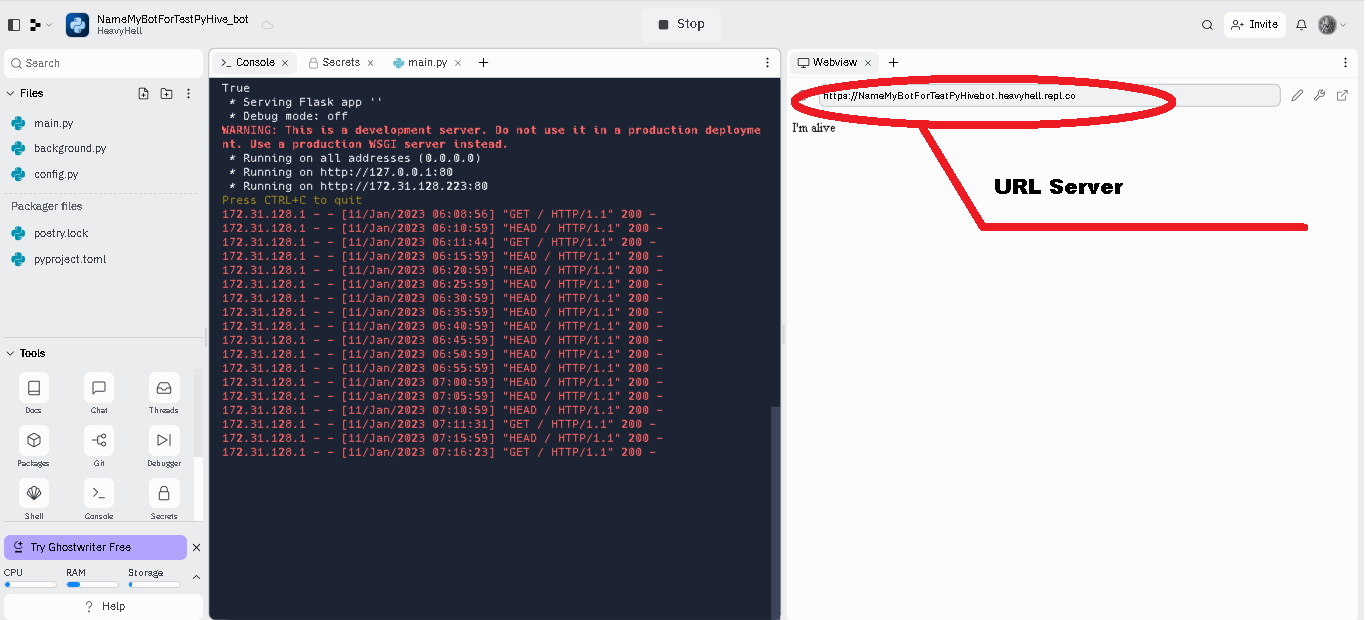
Copy the link to our server to add it to the monitoring service uptimerobot.com
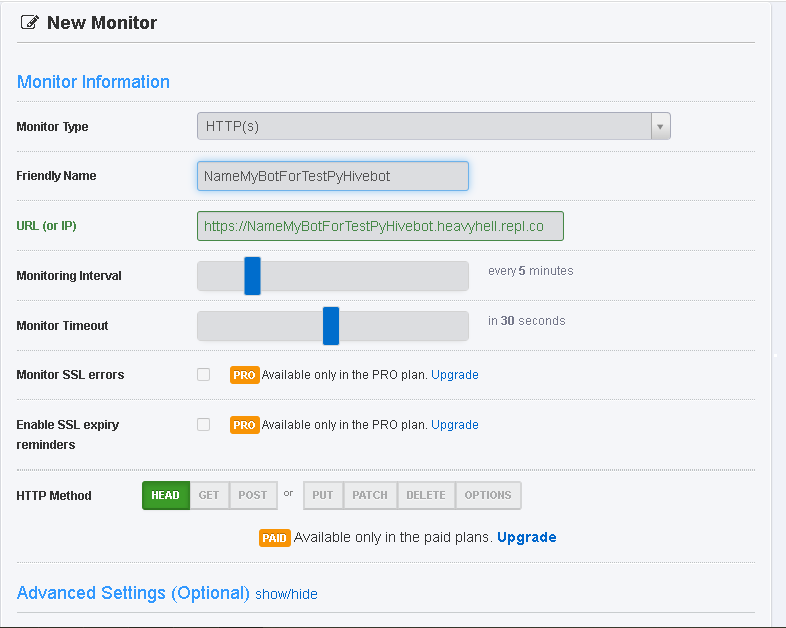
That's all done!!! We will not miss any post containing the word Giveaway in the title.
In reality, we have over a hundred people in our hive doing a Giveaway. Therefore, you can modify the script a little.
For example add another list.
checklistgivers = {'aftersound','apprentice001','rtonline'}
Where to list those in whose Giveaway you want to participate. After that, in the bot, replace the lines
// If this is a new post, check if the word from the checklist is in the title
if check_pattern_func(op['title'],checklist):
// Form a link to the post
mess = hive_front+"@"+op['author']+"/"+op['permlink']
// Send it to the channel
send_message_func(mess)
replaced by
// If this is a new post, check if the word from the checklist is in the title
if check_pattern_func(op['title'],checklist):
// If a new post was made by an author from the checklistgivers list
if check_pattern_func(op['author'],checklistgivers):
// Form a link to the post
mess = hive_front+"@"+op['author']+"/"+op['permlink']
// Send it to the channel
send_message_func(mess)
Then we will receive notifications only about them.
There are an incredible number of options for why to listen to the entire blockchain
- Listen to receive notifications in case of transfer of coins from YOUR accounts (as from a bank about suspicious transfers)
- Listen for notifications of significant token price changes
- Listen for notifications of transfers of significant amounts of tokens
- Organize auto-staking (if you put the key in the environment variables, you can use the script Splinterlands SPS Auto-Staker v.1.2 by @slobberchops )
Posted with STEMGeeks
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
You have done an impressive job!
I will try to make time to test this method.
Thank you very much, this is very interesting!
!BBH
@alinalazareva! Your Content Is Awesome so I just sent 1 $BBH (Bitcoin Backed Hive) to your account on behalf of @contagio. (4/5)
Hello @alinalazareva nice to meet you.
Barb 😊
!BBH
!CTP
!ALIVE
@adcreatordesign, sorry! You need more $ALIVE to use this command.
The minimum requirement is 1000.0 ALIVE staked.
More $ALIVE is available from Hive-Engine or Tribaldex
That is an amazing job
!1UP
You have received a 1UP from @gwajnberg!
@leo-curator, @ctp-curator, @stem-curator, @vyb-curator, @pob-curator
And they will bring !PIZZA 🍕.
Learn more about our delegation service to earn daily rewards. Join the Cartel on Discord.
I gifted $PIZZA slices here:
@curation-cartel(18/20) tipped @alinalazareva (x1)
Send $PIZZA tips in Discord via tip.cc!
Dear @alinalazareva,
Our previous proposal expired end of December and the Hivebuzz project is not funded anymore. May we ask you to review and support our new proposal (https://peakd.com/me/proposals/248)?
Thank you for your help!
I've been wanting to set up a way to auto-stake tokens in my accounts. This could very well be the knowledge I was looking for. Thank you very much. I'll have to find some time to get it all configured and see if I can make it work the way I want.
It's not as difficult as it seems.
To do this, you need to at least fill up
Thanks for sharing all that work you did. One of those posts that I will keep for future reference.
!ALIVE
!CTP
Posted via
Veews
@alinalazareva! You Are Alive so I just staked 0.1 $ALIVE to your account on behalf of @lisamgentile1961. (3/10)
The tip has been paid for by the We Are Alive Tribe through the earnings on @alive.chat, feel free to swing by our daily chat any time you want.

Good Morning and Thank you, @youarealive! Enjoy your day.😀