Android App Development | Lecture#21 | Hive Learners
𝓖𝓻𝓮𝓮𝓽𝓲𝓷𝓰𝓼
Hello, dear Hive Learners, I hope you all are well. Today is our 21st lecture on Android App Development. We will start developing a Sign-in and Sign-up page. Today We will design the Signup page with the Material Design.
GitHub Link
Use this GitHub project to clone into your directory. It will constantly get updated in the following lecture so you will never miss the latest code. Happy Coding!.
What Should I Learn
- Add Material Design implementation in Gradle file
- Use the Material design to create a sign-up page design.
Assignment
- Create a design for the Signup page
Procedure
First, we need to add the Material Design library to the Gradle file. Use this implementation. After adding the library click on the sync button. Make sure that you have a reliable internet connection. After the Gradle successful build, we can use the Material Designs in our XML file.
implementation 'com.google.android.material:material:1.0.0'
On the sign-up page, we are going to add 4 edittext fields (name, email, password, confirm password) We will use 3 buttons (signup, signing). Due to Material design, we can style the whole page easily. I have created this design. You can make your design. Here is the code for the main_activity.xml
file for this design.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="8dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginBottom="5dp"
android:text="CREATE ACCOUNT"
android:textSize="26sp"
android:textStyle="bold" />
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter Your Name"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:hint="Enter Your Email"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="horizontal">
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:layout_marginEnd="2dp"
android:layout_weight=".5"
android:hint="Enter Password"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="2dp"
android:layout_marginTop="5dp"
android:layout_weight=".5"
android:hint="Confirm Password"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
</LinearLayout>
<com.google.android.material.button.MaterialButton
style="@style/Widget.AppCompat.Button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="10dp"
android:backgroundTint="#2987B1"
android:text="Sign up"
android:textColor="@color/white"
app:cornerRadius="4dp" />
</LinearLayout>
</LinearLayout>
We also need to set the IDs for all the widgets to use in the java file. I have set the IDs (signup_name_et, signup_email_et, signup_pass_et, signup_confirm_pass_et, signup_btn)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:padding="8dp">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginBottom="5dp"
android:text="CREATE ACCOUNT"
android:textSize="26sp"
android:textStyle="bold" />
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter Your Name"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:id="@+id/signup_name_et"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:hint="Enter Your Email"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:id="@+id/signup_email_et"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:orientation="horizontal">
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:layout_marginEnd="2dp"
android:layout_weight=".5"
android:hint="Enter Password"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:id="@+id/signup_pass_et"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="2dp"
android:layout_marginTop="5dp"
android:layout_weight=".5"
android:hint="Confirm Password"
app:boxStrokeColor="@color/black"
app:boxStrokeWidth="1dp">
<EditText
android:id="@+id/signup_confirm_pass_et"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</com.google.android.material.textfield.TextInputLayout>
</LinearLayout>
<com.google.android.material.button.MaterialButton
android:id="@+id/signup_btn"
style="@style/Widget.AppCompat.Button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="10dp"
android:backgroundTint="#2987B1"
android:text="Sign up"
android:textColor="@color/white"
app:cornerRadius="4dp" />
</LinearLayout>
</LinearLayout>
We also need a Sign-in button if a user already has an account then the user clicks on sign-in or Already has an account button. I am using a borderless button here.
<com.google.android.material.button.MaterialButton
android:id="@+id/already_act_btn"
style="@style/Widget.AppCompat.Button.Borderless"
android:layout_width="wrap_content"
android:layout_height="35dp"
android:layout_gravity="center"
android:text="Already Have an Account"
android:textColor="@color/black"
android:textSize="14sp"
app:backgroundTint="@null"
android:background="@null"/>

Thank You
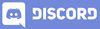
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
That is very important for an app !! I am interesting to see the next steps.
!1UP
Click this banner to join "The Cartel" discord server to know more.
You have received a 1UP from @gwajnberg!
@stem-curator
And they will bring !PIZZA 🍕.
Learn more about our delegation service to earn daily rewards. Join the Cartel on Discord.
Yay! 🤗
Your content has been boosted with Ecency Points, by @faisalamin.
Use Ecency daily to boost your growth on platform!
Support Ecency
Vote for new Proposal
Delegate HP and earn more