Try, Except: Examples in practice / Try, Except: Ejemplos en práctica - Coding Basics #24
Putting Error Handling to the Test
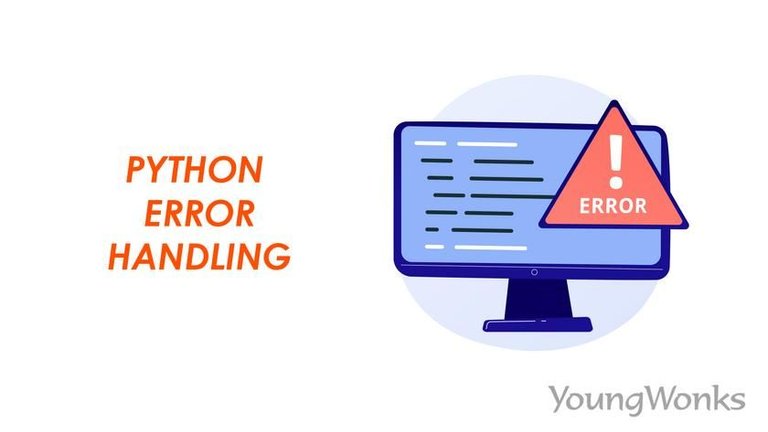
Shoutout to YoungWonks
In this article you'll find:
- Introduction
- Example 1
- Example 2
- Example 3
- Example 4
In the last chapter of Coding Basics, we talked about the Try Except block, a set of instructions that allows us to check our code for errors and do something about them if they occur.
However, we only got a cursory glimpse of the theory behind it. For this reason, in this post we will see some examples of the Try Except application, not only to understand it in greater depth, but also so that you can apply it to your own projects.
Having said that,
Example 1
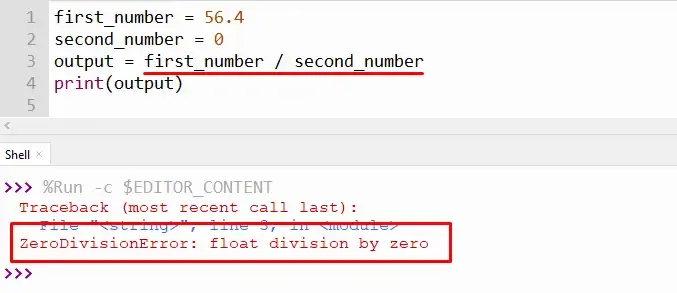
Shoutout to Its Linux FOSS
In this first example, we'll start with the easiest level of difficulty, where we'll create a program that allows us to divide two numbers. Although it may seem very innocent, there are certain errors that can put you in danger. These are:
- Dividing other values than numbers.
- Dividing a number by 0.
If we divide with values that are not numeric, we will have the following as a result:
Traceback (most recent call last):
File "C:\Yourfiledirectory", line n, in <module>
TypeError: unsupported operand type(s) for /: 'str' and 'str'
And if we divide by zero, we will get the following error:
Traceback (most recent call last):
File "C:\Yourfiledirectory", line n, in <module>
ZeroDivisionError: division by zero
Thus, we must make sure that this does not happen. In case we get a TypeError, we'll tell the user that they can only use numbers and if we get a ZeroDivision Error, we'll tell them that it can't be divided by zero.
How do we do this?
Very easy. If we remember from the previous chapter, the try except statements will be our choice. We just have to place the division operation inside the try to verify that everything goes well. In case there is a specific error, we will except give a message. Thus, knowing that dividing by zero we will have a ZeroDivisionError and that with values that are not numbers we will have a ValueError:
try:
number1 = int(input('Please enter a number for your numerator'))
number2 = int(input('Please enter a number for your denominator'))
result = number1/number2
print(result)
except ZeroDivisionError:
print('You can\'t divide by Zero')
except ValueError:
print('Only numbers are allowed')
If we execute with accepted values:
>>> Please enter a number for your numerator5
Please enter a number for your denominator5
1.0
If we divide by zero:
>>> Please enter a number for your numerator 5
Please enter a number for your denominator 0
You can't divide by Zero
And if we split two strings:
>>> Please enter a number for your numerator hello
Only numbers are allowed
Example 2
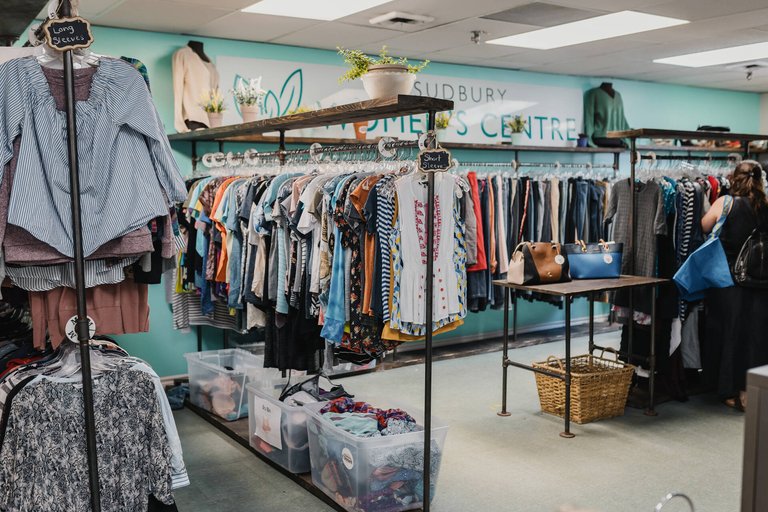
Shoutout to Sudbury Women's Centre
In this case, we will do an exercise with the MockUp of a Boutique page. Here we will display a text string that introduces us to Paul's Boutique. Then, we will be shown a series of Items. These are:
- A coat.
- A jacket.
- A suit.
- Some designer shoes.
- A leather belt.
All these items will be saved in a list with the corresponding names. Now, what corresponds is to give the user the option to select one of these. In this case, we will ask for the Item number as shown on the screen.
items = ['Coat', 'Jacket', 'Suit', 'Designer Shoes', 'Leather Belt']
As long as the user puts in a numeric value and it's within the list's index range, everything will be fine. However, if you place a value greater than the number of elements in the list, an IndexError will jump to which we will place 'The item is not in the list'.
Thus, applying a Try Except the program would look like this:
try:
items = ['Coat', 'Jacket', 'Suit', 'Designer Shoes', 'Leather Belt']
print('Welcome to Paul\'s Boutique. Select the Item you want')
print('************************************************ ********')
print('''
1 | Coat
2 | Jacket
3 | suit
4 | Designer Shoes
5 | Leather Belt''')
answer = int(input())
print(f'You have selected the {items[answer-1]}')
except IndexError:
print('The Item is not on the list')
except:
print('Use numbers to select an article')
We can note that the int will guarantee that the input type must always be an integer type, which will mean that in case of placing a non-integer value, we will have a ValueError or TypeError.
Also, we notice that when displaying the value, we use an fstring. The particularity here is given by the use of answer-1 when looking for the index of the object in the items list. This is because when counting in lists, we start with the number 0 (Which in this case would be Coat), with which, by subtracting one from the number we place, we would be in the correct position.
Finally, as we indicated, if the IndexError occurs, with the except we indicate that the Item is not in the list and in case of any other exception, we ask the user to use numbers.
Example 3
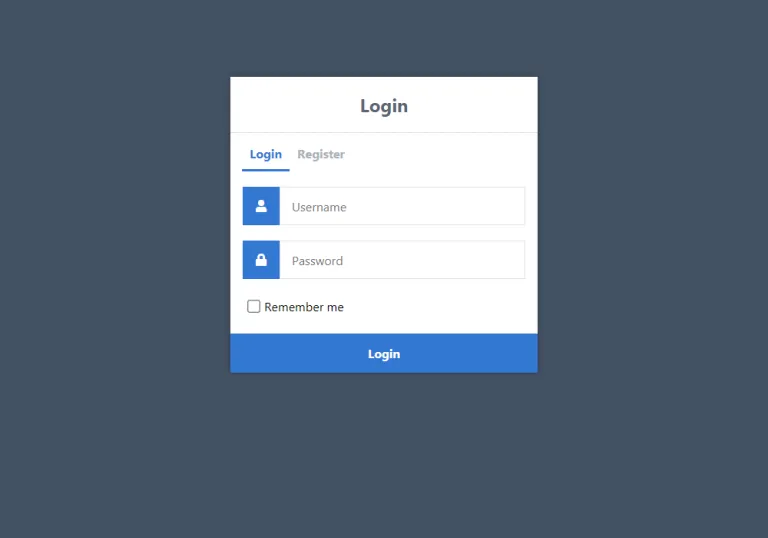
Shoutout to CodeShack
Now, we will simulate the login interface of a banking company. For this, we will ask the user for his username and password. Although it would be much more efficient to use dictionaries for these operations, in this case we will use two lists: one for usernames and one for passwords.
So how do we go about verifying that the user is on the list, has the correct password?
For this, in addition to the try except, we will use two instructions that will be of great help: assert and index.
index is a method of the list class, which allows us to identify the specific index of an element in the list by introducing its value as a parameter. For example:
list1 = ['hello','hi','welcome']
element1 = list1.index('hi')
print(element1)
>>> 1
Which will be correct, since 'hi', is the value of index one in the list.
For assert, think of this as a conditional, where if the condition the assert is trying to test for is true, it returns true and continues with the program. In case it is not fulfilled, this will immediately return an error, specifically an AssertionError. For example:
a = 1
b = 2
assert a + b == 3
print('a + b =', (a + b))
>>> a + b = 3
And if this condition is not met:
a = 1
b = 2
assert a + b == 2
print('a + b =', (a + b))
>>> a + b = 3
Taking this into account, we can go on to design our program. First, we must create a list with username and password values that we want. Then, we must request the user the information to verify if they are in the system:
users = ['John23', 'Francis35', 'Damian50']
passwords = ['momloveyou', 'money$$35', '452686']
print('Welcome to Piggy Bank. Here you can see the balance of your account')
print('Please insert your username:')
user = str(input())
print('Please insert your password:')
password = str(input())
Then, it's time to perform the verification logic. For this, we will use try, except, assert and index. So:
try:
indexuser = users.index(user)
indexpassword = passwords.index(password)
assert indexuser == indexpassword
except AssertionError:
print('user and password don\'t match ')
except:
print('User or Password not recognized')
else:
print('User and Password Checked, You can enter')
Here, we can see that two variables are created to identify the indices. indexuser uses the index statement to identify where the user is in the list, just like index password.
Then, with assert we verify that both indices are in the same position, that is, that the user has his password and not someone else's. In case this is not fulfilled, the except code is executed with AssertionError, which tells us that the password does not belong to the user.
In the event that other errors occur, such as a ValueError, in case of entering a user that is not on the list, it will tell us that neither the user nor the password are recognized.
Then, with the else, which is executed only if the try was successful, it will tell us that the username and password were verified, which is why the bank can be entered.
Example 4
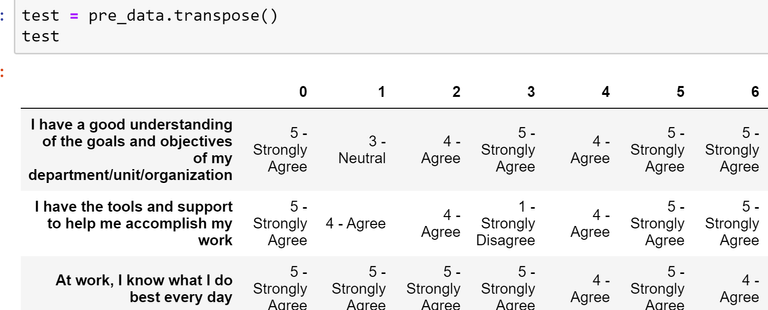
Shoutout to Amanda Wishnie in Stack Overflow
Now, we will create a survey, where we will ask the user a couple of questions that he must answer exclusively with a yes or no. These questions are:
- Are you from the United States?
- Do you like chewing gum?
The only condition for everything to work will be that the answers can only be yes/no. However, in this case we will not use try except, but simply use the raise statement. Remember that raise allows us to place any error message we want when a condition is met or not met.
For this, what we will do is create a conditional, where if the condition that we write yes or no as an answer is met, the program continues to run normally, saving our answer in a list. Otherwise, we use the raise to show us the 'Only use yes or no' error.
Thus, if we proceed to the first part of the program, we create the list and two variables that will be our responses, as well as the introduction to the program:
answers = []
answer1 = '';
answer2 = '';
print(''' In this survey, we'll present you a series of questions
you have to answer with Yes/No, any other answer will raise an error''')
Next, we create the questions and the inputs for their answers inside the variables:
print('''Question 1: \n
Are you from the US?''')
answer1 = str(input())
print('''Question 2: \n
Do you like bubble gum?''')
answer2 = str(input())
Now, we would be left with logic, where we will verify with the conditional if the answer is yes or no. If we were to create a conditional for each question, we would be using more lines of code with identical operations, which is not good practice.
Whenever a procedure is constantly repeated in a program, it's best to create a function for it. What we will do:
def ifyesno(answer):
if answer == 'yes' or answer == 'no':
answers.append(answer)
else:
raise ValueError('The information should be either yes or no')
ifyesno takes answer as a parameter (which can be any of the two variables as we indicate when calling it) and will ask if the text is yes or no. If it is, it uses append to add to the list and if it is not, it simply shows us a ValueError that tells us that they should only be yes or no.
Once this function is defined, we only have to place it at the end of each question with the appropriate variable so that it can be easily executed:
print('''Question 1: \n
Are you from the US?''')
answer1 = str(input())
ifyesno(answer1)
print('''Question 2: \n
Do you like bubble gum?''')
answer2 = str(input())
ifyesno(answer2)
And finally, in case the instruction is executed successfully:
print('Thank you for your time. Your answers were:')
for i in answers:
print(i)
With which it will show us the result of each question, going through the list of answers with the for loop. So, when running:
>>>
In this survey, we'll present you a series of questions
you have to answer with Yes/No, any other answer will raise an error
****************************
Question 1:
Are you from the US?
forks
Question 2:
Do you like bubble gum?
No
Thank you for your time. Your answers were:
forks
No
If a 'yes' value or a 'no' value is not placed
In this survey, we'll present you a series of questions
you have to answer with Yes/No, any other answer will raise an error
****************************
Question 1:
Are you from the US?
hkfgj
Traceback (most recent call last):
File "C:\Users\rogelio\Desktop\PythonProjects\exampleforpy.py", line 79, in <module>
ifyesno(answer1)
File "C:\Users\rogelio\Desktop\PythonProjects\exampleforpy.py", line 70, in ifyesno
raise ValueError('The information should be either yes or no')
ValueError: The information should be either yes or no
Bug handling is something every programmer deals with on a day-to-day basis, getting to the point where on certain days, more than half of your programming day will be resolving bugs. This is why the handling of instructions for errors becomes critical.
After these examples and the theory in the previous chapter, you will have an expert understanding of Error Handling, being able to apply it to your projects to create program opportunities and add greater reliability to them.
This is why I encourage you to continue on your path and be proud of the progress made to date.
Buckle up, because the most interesting parts will come soon.
Poniendo el Error Handling a Prueba
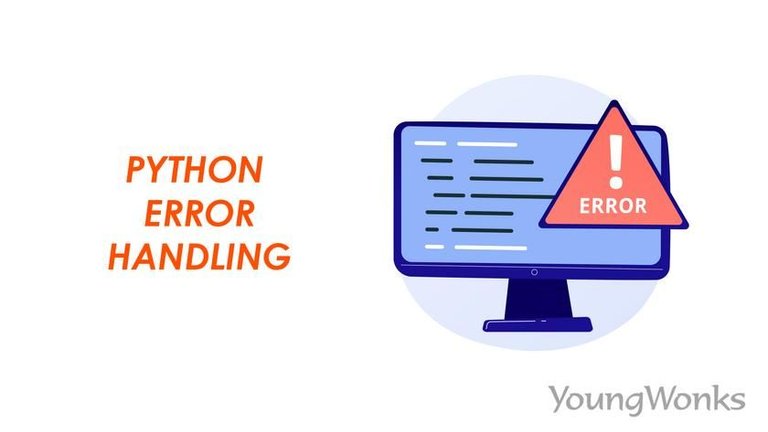
Shoutout to YoungWonks
En este artículo encontrarás
- Introducción
- Ejemplo 1
- Ejemplo 2
- Ejemplo 3
- Ejemplo 4
En el capítulo pasado de Coding Basics, hablamos sobre el bloque Try Except, un conjunto de instrucciones que nos permite verificar nuestro código en busqueda de errores y hacer algo al respecto si estos ocurren.
Sin embargo, solo vimos de manera superficial la teoría detrás de esto. Es por esto, que en este post veremos algunos ejemplos de la aplicación de Try Except, no solo para entender a mayor profundidad, sino para que puedes aplicarlo a tus propios proyectos.
Dicho esto,
Ejemplo 1
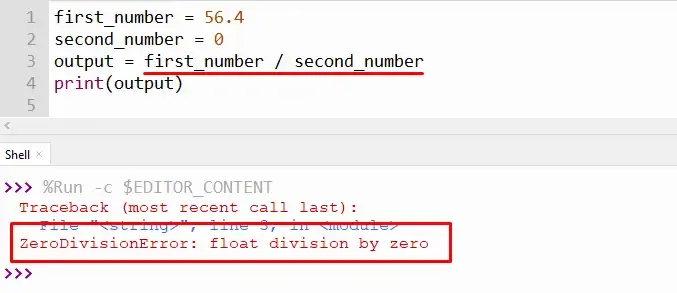
Shoutout to Its Linux FOSS
En este primer ejemplo, comenzaremos con el nivel más sencillo de dificultad, donde crearemos un programa que nos permita dividir dos números. Aunque pueda parecer muy inocente, existen ciertos errores que lo pueden poner en peligro. Estos son:
- El dividir otros valores que no sean números.
- El dividir un número entre 0.
Si dividimos con valores que no son numéricos, tendremos lo siguiente como resultado:
Traceback (most recent call last):
File "C:\Yourfiledirectory", line n, in <module>
TypeError: unsupported operand type(s) for /: 'str' and 'str'
Y Si dividimos entre cero, obtendremos el siguiente error:
Traceback (most recent call last):
File "C:\Yourfiledirectory", line n, in <module>
ZeroDivisionError: division by zero
Así, debemos de cerciorarnos de que esto no ocurra. En caso de que tengamos un TypeError, le avisaremos al usuario que solo pueden usar números y si nos ZeroDivision Error, les diremos que no se puede dividir por cero.
¿Cómo hacemos esto?
Muy sencillo. Si recordamos el capítulo anterior, las instrucciones try except serán nuestra elección. Solo debemos colocar la operación de división dentro del try para verificar que todo vaya bien. En caso de que haya un error específico, con except daremos un mensaje. Así, sabiendo que dividiendo entre cero tendremos un ZeroDivisionError y que con valores que no sean números tendremos un ValueError:
try:
number1 = int(input('Please enter a number for your numerator'))
number2 = int(input('Please enter a number for your denominator'))
result = number1/number2
print(result)
except ZeroDivisionError:
print('You can\'t divide by Zero')
except ValueError:
print('Only numbers are allowed')
Si ejecutamos con valores aceptados:
>>> Please enter a number for your numerator5
Please enter a number for your denominator5
1.0
Si dividimos entre cero:
>>> Please enter a number for your numerator 5
Please enter a number for your denominator 0
You can't divide by Zero
Y si dividimos dos string:
>>> Please enter a number for your numerator hello
Only numbers are allowed
Ejemplo 2
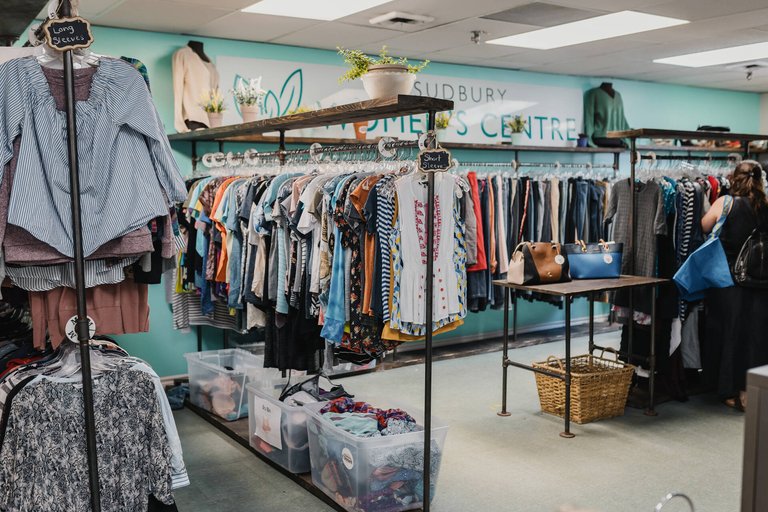
Shoutout to Sudbury Women's Centre
En este caso, haremos un ejercicio con el MockUp de una página de Boutique. Aquí mostraremos una cadena de texto que nos introduzca a Paul's Boutique. Luego, se nos mostrará una serie de Items. Estos son:
- Un abrigo.
- Una chaqueta.
- Un traje.
- Unos zapatos de diseñador.
- Un cinturón de cuero.
Todos estos items serán guardados en una lista con los nombres correspondientes. Ahora, lo que corresponde es dar al usuario la opción de seleccionar uno de estos. En este caso, les pediremos el número del Item según lo mostrado en la pantalla.
items = ['Coat', 'Jacket', 'Suit', 'Designer Shoes', 'Leather Belt']
Mientras que el usuario coloque un valor numérico y que esté dentro del rango de índices de la lista, todo estará bien. Sin embargo, si coloca un valor mayor a la cantidad de elementos en la lista, saltará un IndexError al que colocaremos 'El item no está en la lista'.
Así, aplicando un Try Except el programa se vería de la siguiente manera:
try:
items = ['Coat', 'Jacket', 'Suit', 'Designer Shoes', 'Leather Belt']
print('Welcome to Paul\'s Boutique. Select the Item you want')
print('*********************************************************')
print('''
1 | Coat
2 | Jacket
3 | Suit
4 | Designer Shoes
5 | Leather Belt''')
answer = int(input())
print(f'You have selected the {items[answer-1]}')
except IndexError:
print('The Item is not on the list')
except:
print('Use numbers to select an article')
Podemos notar que el int, garantizará que el tipo de input deba ser siempre de tipo entero, lo que hará que en caso de colocar un valor no entero, tengamos un ValueError o TypeError.
Además, notamos que al mostrar el valor, usamos un fstring. La particularidad aquí se da por el uso de answer-1 a la hora de buscar el índice del objeto en la lista items. Esto se debe a que al contar en listas, se empieza por el número 0 (Que en este caso sería Coat), con lo que, al restar uno al número que coloquemos, estaríamos en la posición correcta.
Finalmente, como indicamos, si ocurre el IndexError, con el except indicamos que el Item no está en la lista y en caso de cualquier otra excepción, le pedimos al usuario que use números.
Ejemplo 3
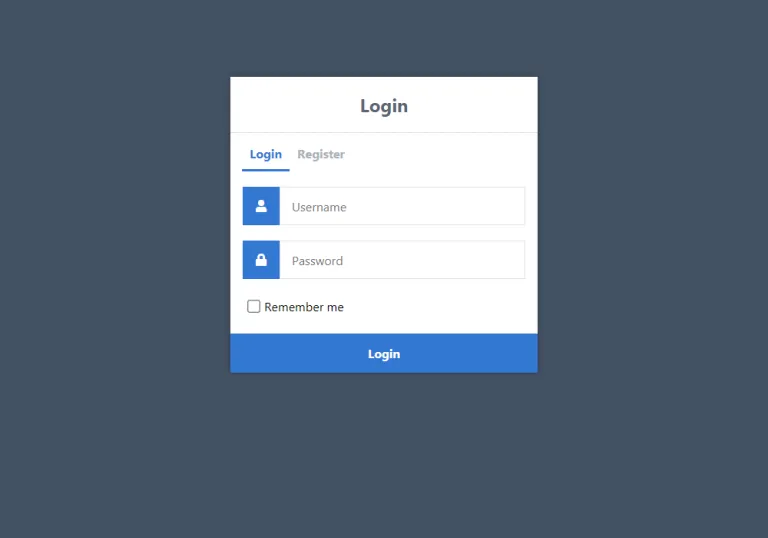
Shoutout to CodeShack
Ahora, simularemos la interfaz de login de una empresa bancaria. Para esto, le pediremos al usuario su nombre de usuario y la contraseña. Aunque sería mucho más eficiente el uso de diccionarios para estas operaciones, en este caso usaremos dos listas: una para los nombres de usuario y otra para las contraseñas.
Entonces, ¿Cómo haremos para verificar que el usuario se encuentre en la lista, tenga la contraseña adecuada?
Para esto, además del try except, haremos uso de dos instrucciones que nos serán de gran ayuda: assert e index.
index es un método propio de la clase list, el cual nos permite identificar el índice específico de un elemento en la lista con introducirle su valor como parámetro. Por ejemplo:
list1 = ['hello','hi','welcome']
element1 = list1.index('hi')
print(element1)
>>> 1
Lo cual será correcto, ya que 'hi', es el valor del índice uno en la lista.
Para assert, piensa en este como un condicional, donde si la condición que el assert trata de verificar se cumple, nos devuelve un true y continua con el programa. En caso de que no se cumpla, este nos devolverá de inmediato un error, específicamente un AssertionError. Por ejemplo:
a = 1
b = 2
assert a + b == 3
print('a + b =', (a + b))
>>> a + b = 3
Y en caso de que no se cumpliera esta condición:
a = 1
b = 2
assert a + b == 2
print('a + b =', (a + b))
>>> a + b = 3
Tomando esto en cuenta, podemos pasar a diseñar nuestro programa. Primero, debemos de crear una lista con valores de usuario y contraseña que deseemos. Luego, debemos de solicitar al usuario la información para verificar si se encuentran en el sistema:
users = ['John23', 'Francis35', 'Damian50']
passwords = ['momloveyou', 'money$$35', '452686']
print('Welcome to Piggy Bank. Here you can see the balance of your account')
print('Please insert your username:')
user = str(input())
print('Please insert your password:')
password = str(input())
Luego, toca realizar la lógica de verificación. Para esto, usaremos try, except, assert e index. Así:
try:
indexuser = users.index(user)
indexpassword = passwords.index(password)
assert indexuser == indexpassword
except AssertionError:
print('user and password don\'t match ')
except:
print('User or Password not recognized')
else:
print('User and Password Checked, You can enter')
Aquí, podemos ver que se crean dos variables para identificar los índices. indexuser usa la instrucción index para identificar en que posición de la lista se encuentra el usuario, al igual que index password.
Luego, con assert verificamos que ambos índices se encuentren en la misma posición, es decir, que el usuario tenga su contraseña y no la de otra persona. En caso de que no se cumpla esto, se ejecuta el código del except con AssertionError, el cual nos indica que la contraseña no pertenece al usuario.
En caso de que se produzcan otros errores, como puede ser un ValueError, en caso de introducir un usuario que no se encuentre en la lista, nos dirá que ni el usuario ni la contraseña son reconocidos.
Luego, con el else, que se ejecuta solo si el try fue exitoso, nos dirá que el usuario y la contraseña fueron verificados, razón por la cual se puede entrar al banco.
Ejemplo 4
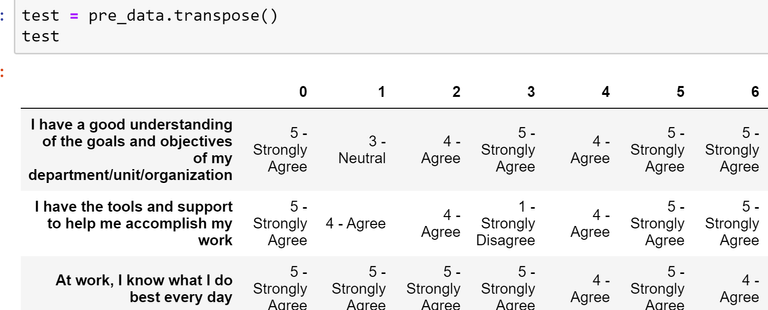
Shoutout to Amanda Wishnie in Stack Overflow
Ahora, crearemos una encuesta, donde le haremos un par de preguntas al usuario que debe de responder exclusivamente con un si o no. Estas preguntas son:
- ¿Eres de los Estados Unidos?
- ¿Te gusta la goma de mascar?
La única condición para que funcione todo será que las respuestas solo pueden ser si/no. Sin embargo, en este caso no usaremos try except, sino que usaremos simplemente la instrucción raise. Recordamos que raise nos permite colocar cualquier mensaje de error que deseemos al cumplirse o no cumplirse una condición.
Para esto lo que haremos es crear un condicional, donde si se cumple la condición de que escribimos si o no como respuesta, se sigue ejecutando el programa normalmente, guardando nuestra respuesta en una lista. En caso contrario, usamos el raise para que nos muestre el error de 'Solo usar si o no'.
Así, si procedemos a la primera parte del programa, creamos la lista y dos variables que serán nuestras respuestas, así como la introducción al programa:
answers = []
answer1 = '';
answer2 = '';
print(''' In this survey, we'll present you a series of question
you have to answer with Yes/No, any other answer will raise an error''')
Luego, creamos las preguntas y los inputs para sus respuestas dentro de las variables:
print('''Question 1: \n
Are you from the US?''')
answer1 = str(input())
print('''Question 2: \n
Do you like bubble gum?''')
answer2 = str(input())
Ahora, nos quedaría la lógica, donde verificaremos con el condicional si se cumple que la respuesta es un si o no. Si crearamos un condicional para cada pregunta, estaríamos usando más líneas de código con operaciones idénticas, cosa que no es buena práctica.
Siempre que un procedimiento se repita de manera constante en un programa, lo mejor será crear una función para eso. Cosa que haremos:
def ifyesno(answer):
if answer == 'yes' or answer == 'no':
answers.append(answer)
else:
raise ValueError('The information should be either yes or no')
ifyesno toma como parámetro answer (Que puede ser cualquiera de las dos variables según se lo indiquemos al llamarla) y preguntará si el texto es si o no. En caso de que si lo sea, usa append para añadir a la lista y en caso de que no, simplemente nos muestra un ValueError que nos indica que solo deben ser si o no.
Una vez definida esta función, solo nos queda colocarla al final de cada pregunta con la variable adecuada para que se ejecute facilmente:
print('''Question 1: \n
Are you from the US?''')
answer1 = str(input())
ifyesno(answer1)
print('''Question 2: \n
Do you like bubble gum?''')
answer2 = str(input())
ifyesno(answer2)
Y finalmente, en caso de que la instrucción se ejecute de manera satisfactoria:
print('Thank you for your time. Your answers were:')
for i in answers:
print(i)
Con lo que nos mostrará el resultado de cada pregunta, recorriendo la lista de respuestas con el ciclo for. Así, al ejecutar:
>>>
In this survey, we'll present you a series of question
you have to answer with Yes/No, any other answer will raise an error
**************************
Question 1:
Are you from the US?
yes
Question 2:
Do you like bubble gum?
no
Thank you for your time. Your answers were:
yes
no
Si no se coloca un valor 'yes' o un valor 'no'
In this survey, we'll present you a series of question
you have to answer with Yes/No, any other answer will raise an error
**************************
Question 1:
Are you from the US?
hkfgj
Traceback (most recent call last):
File "C:\Users\rogelio\Desktop\ProyectosPython\exampleforpy.py", line 79, in <module>
ifyesno(answer1)
File "C:\Users\rogelio\Desktop\ProyectosPython\exampleforpy.py", line 70, in ifyesno
raise ValueError('The information should be either yes or no')
ValueError: The information should be either yes or no
El manejo de errores es algo con lo que cada programador lidia en el día a día, llegando al punto en el que en ciertos días, más de la mitad de tu jornada de programación será resolver errores. Es por esto que se hace crítico el manejo de instrucciones para errores.
Tras estos ejemplos y la teoría en el capítulo anterior, tendrás un entendimiento experto del Error Handling, pudiendo aplicarlo a tus proyectos para crear oportunidades de programas y añadir mayor fiabilidad a estos.
Es por esto, que te animo a continuar tu camino y enorgullecerte del progreso realizado hasta hoy.
Abrochate el cinturón, porque ya vendrán las partes más interesantes.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.