Numpy Part #4: Numpy in practice / Numpy Parte 4: Numpy en práctica - Coding Basics #33
Putting Numpy into practice
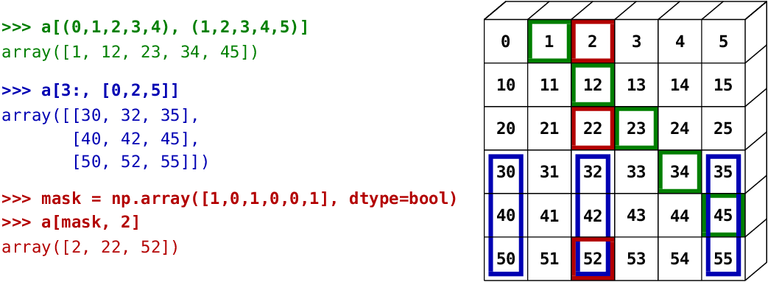
Shoutout to Towards Data Science
In this article you will find:
- Introduction
- Example 1
- Example 2
- Example 3
Continuing with numPy, where so far we have seen how to create and manage arrays as well as use the random module to create random values. However, we have not seen much of its application in practice.
This is why in the next post we will see some examples where we will use the random module and its methods/functions to create the applications we want. From trivia to database systems, you'll see the potential of random.
Without more to say,
Example 1
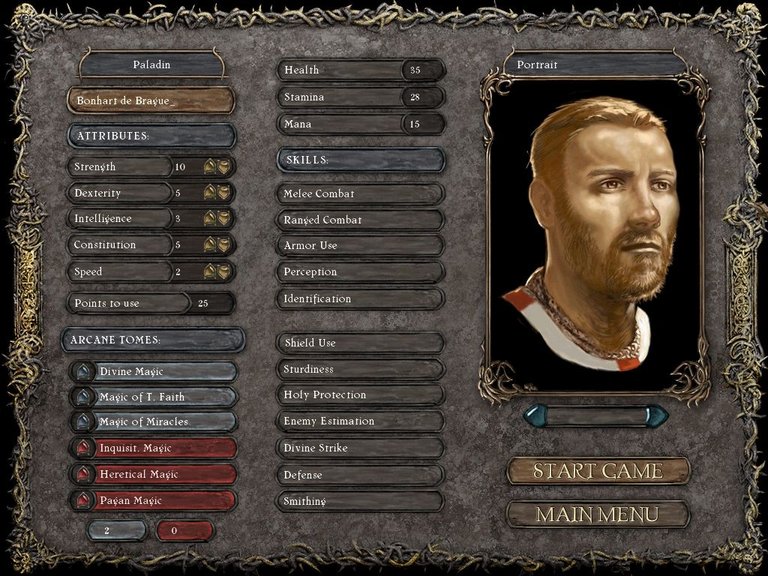
[Shoutout to ByGoneBytes](https://bygonebytes.wordpress.com/2015/07/14/indie-spotlight-inquisitor-pc-the-child-of-bauldurs-gate-and-diablo-gets-brutal /)
For this first example, we will have something simple. Remember those character creation menus where you can spend longer creating a character than playing the game? You can do that or just place it randomly and have the algorithm generate a character for you.
That is precisely what we will do here, where according to a series of attributes that we will place within arrays, we will be able to deliver random characters to the user.
So, the only thing you have to keep in mind:
- We must use random.choice to be able to place the characteristics at random within a range that we can control (That is, that the eye color is a color that we tell it, etc...)
Thus, we begin using this method to generate the values, which will be:
- Gender.
- Eye color.
- Skin color.
- Hair color.
- Height.
Thus, among the values we will have:
import numpy
Gender = numpy.array(['Man', 'Woman'])
EyeColor = numpy.array(['Black','Brown','Green','Blue','Yellow','Grey'])
SkinColor = numpy.array(['Black','White','Yellow','Purple','Green'])
HairColor = numpy.array(['Black,','Brown','White','Grey','Blonde','Red'])
Height = numpy.array(['Tall', 'Average Height', 'Small'])
And now, just create a function that we will use whenever we want to create a character. We remember that we created it within a function so that we do not have to write all the code again if we want to do this operation.
import numpy
Gender = numpy.array(['Man', 'Woman'])
EyeColor = numpy.array(['Black','Brown','Green','Blue','Yellow','Grey'])
SkinColor = numpy.array(['Black','White','Yellow','Purple','Green'])
HairColor = numpy.array(['Black,','Brown','White','Grey','Blonde','Red'])
Height = numpy.array(['Tall', 'Average Height', 'Small'])
def generate_character(Gender,EyeColor,SkinColor,HairColor,Height):
sex = numpy.random.choice(Gender)
eye = numpy.random.choice(EyeColor)
skin = numpy.random.choice(SkinColor)
hair = numpy.random.choice(HairColor)
length = numpy.random.choice(Height)
print(f'Your character is a {sex} with {skin} skin, {eye} eyes, {hair} hair and {length} stature')
generate_character(Gender,EyeColor,SkinColor,HairColor,Height)
Here, we can see that we will use the previously created arrays as parameters, and then, we will store the random values in variables by using random.choice.
Finally, we print the result at the end of the function and execute it. Thus, when running the program:
>>> Your character is a Woman with Green skin, Gray eyes, Blonde hair and Average Height height
Example 2
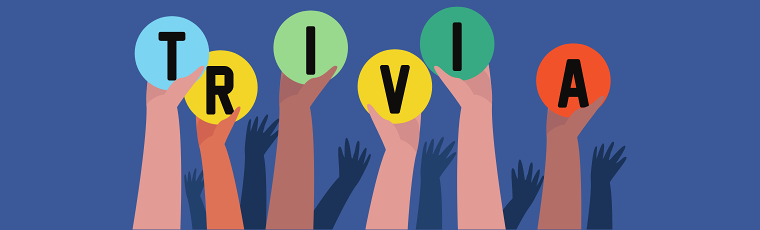
Shoutout to East Lansing Public Library
Now, we return to the trivia exercises. Where we are asked to create a small game with a series of questions that will have the following condition: If you answer correctly, you will automatically have another question and if you answer wrong, the game is over.
However, what will give numpy its charm will be the fact that we will use two arrays to do this, one with the questions and one with the answers:
import numpy
Questions = numpy.array(['What is the Capital of Greece?', 'What\'s the name of James Bond\'s boss?', 'Who won the 2010 world cup?', 'What\'s the most sold Toyota car?'])
Answers = ['Athens','M','Spain','Corolla']
In this case, only questions will be an array with numpy because it is to this that we will apply random.choice to obtain the question by using, then searching for the respective index in the Answers array.
Now, we must create a function that shows us some of the questions in questions and shows it to us in the form of a string, and then takes the index and searches for it in answers. For this, we do the following:
import numpy
Questions = numpy.array(['What is the Capital of Greece?', 'What\'s the name of James Bond\'s boss?', 'Who won the 2010 world cup?', 'What\'s the most sold Toyota car?'])
Answers = ['Athens','M','Spain','Corolla']
def question_answer(Questions, Answers):
array = numpy.random.choice(Questions)
print(str(array))
index = Questions.tolist().index(str(array))
As we can see, what we need to obtain the index is to convert the numpy array to a list with the tolist() method and then use another list method to search for indexes according to a value: index(), where we place as a parameter the string value of random.choice.
Now that we have the index, we need to do two things: Give the user the option to respond and verify if the information they enter is correct. For this, we create a variable with input() to give us a response.
Then, we create a conditional to check if the answer is equal to the answer value in the same position we put in the question (The correct answer), and if true, we give a 'Correct!' and we ask the next question. If not, we finish the program:
import numpy
Questions = numpy.array(['What is the Capital of Greece?', 'What\'s the name of James Bond\'s boss?', 'Who won the 2010 world cup?', 'What\'s the most sold Toyota car?'])
Answers = ['Athens','M','Spain','Corolla']
def question_answer(Questions, Answers):
array = numpy.random.choice(Questions)
print(str(array))
index = Questions.tolist().index(str(array))
to_answ = str(input(''))
if to_answ == str(Answers[index]) or to_answ == str(Answers[index]).lower():
print('Correct!')
question_answer(Questions, Answers)
else:
print('Wrong!')
question_answer(Questions, Answers)
And if we execute:
What's the name of James Bond's boss?
M
Correct!
What's the most sold Toyota car?
Corolla
Correct!
What is the Capital of Greece?
Rome
Wrong!
Example 3
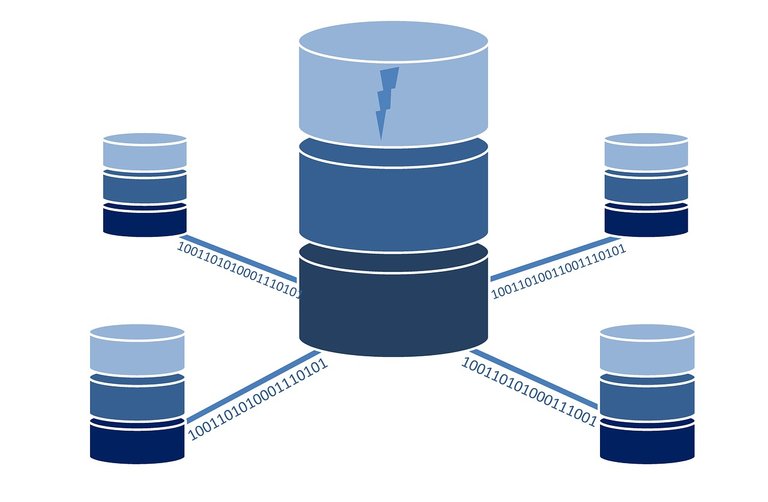
Shoutout to Needpix.com
Finally, we will simulate a database, where we add the name, age and nationality of as many people as the user wants.
To make this possible, we will use a method specific to lists and arrays in Python, which can be used in the same way with the numpy library. This is append:
Thus, the first thing we must do is ask the user for their information. Before this, we create the first array, a two-dimensional array, where each one-dimensional array within it will contain the users' data.
import numpy
total_list = numpy.array([['Name','Age','Country']],ndmin=2)
Then we can create a function where we use this array as a parameter and through variables with input, we enter the variables in two-dimensional arrays with only one element that we will add to our total_list:
def add_person(total_list):
name = str(input('Insert your name'))
age = int(input('Insert your age'))
country = str(input('Where are you from?'))
person_id = numpy.array([[name,age,country]])
Then, we need to add this new array inside total_list. We do this with append, taking as parameters:
- The array to which we will add the new elements (In this case total_list).
- The arrangement with the elements that we will add (For us person_id).
- The axis on which they will be added. Since we want to add an array of one dimension (Which is inside the second dimension), we place the axis 0. Thus, we will have:
total_list = numpy.append(total_list, person_id, axis=0)
Then, we will only have to ask the user if they want to add a new ID. For this, we create a variable with input for yes/no and depending on what we get we repeat the function or show the total of all users. Finally, we call the function with total_list as a parameter:
import numpy
total_list = numpy.array([['Name','Age','Country']],ndmin=2)
def add_person(total_list):
name = str(input('Insert your name'))
age = int(input('Insert your age'))
country = str(input('Where are you from?'))
person_id = numpy.array([[name,age,country]])
total_list = numpy.append(total_list, person_id, axis=0)
repeat = str(input('Do you want to add another person?'))
if repeat == 'yes' or repeat == 'Yes':
add_person(total_list)
else:
print(total_list)
add_person(total_list)
And when you run the program:
>>>
Insert your nameJohn
Insert your age28
Where are you from?Us
Do you want to add another person?Yes
Insert your nameMarc
Insert your age29
Where are you from?France
Do you want to add another person?Yes
Insert your nameGunther
Insert your age30
Where are you from?Germany
Do you want to add another person?Yes
Insert your nameJames
Insert your age30
Where are you from?England
Do you want to add another person?No
[['Name' 'Age' 'Country']
['John' '28' 'Us']
['Marc' '29' 'France']
['Gunther' '30' 'Germany']
['James' '30' 'England']]
As you can see, the use of arrays and the random module with numpy gives us a new range of possibilities that we can take advantage of to create countless programs. If these simple examples are like this, imagine what you can do with this module.
If you think the programs could be designed better or want to add elements like try/except to avoid errors or a higher degree of complexity, go ahead. I am proud of everyone who has reached this point.
We'll see you in the next edition of Coding Basics, continuing with NumPy.
Poniendo Numpy en práctica
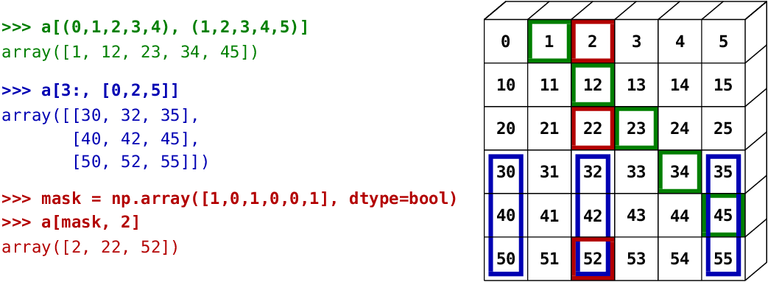
Shoutout to Towards Data Science
En este artículo encontrarás:
- Introducción
- Ejemplo 1
- Ejemplo 2
- Ejemplo 3
Continuando con numPy, donde hasta ahora hemos visto como crear y manejar arreglos así como usar el módulo random para crear valores al azar. Sin embargo, no hemos visto mucho su aplicación en la práctica.
Es por esto, que en el siguiente post veremos algunos ejemplos donde haremos uso del módulo random y sus métodos/funciones para crear las aplicaciones que deseemos. Desde trivias hasta sistemas de bases de datos, podrás ver el potencial de random.
Sin más que decir,
Ejemplo 1
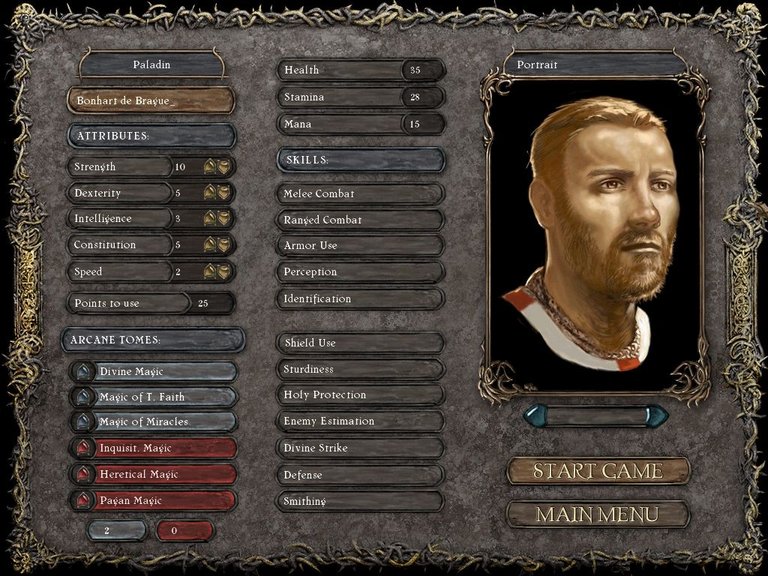
Shoutout to ByGoneBytes
Para este primer ejemplo, tendremos algo sencillo. ¿Recuerdas los menús de creación de personaje donde puedes durar más tiempo creando un personaje que jugando el juego? Puedes hacer eso o simplemente colocarlo al azar y que el algoritmo genere un caracter para ti.
Eso es precisamente lo que haremos aquí, donde acuerdo a una serie de atributos que colocaremos dentro de arreglos, podremos entregar personajes al azar para el usuario.
Así, lo único que hay que tener en cuenta:
- Debemos de usar random.choice para poder colocar las características al azar dentro de un rango que podamos controlar (Es decir, que el color de ojos sea un color que le digamos, etc...)
Así, comenzamos usando este método para ir generando los valores, que serán:
- Género.
- Color de ojos.
- Color de piel.
- Color de Cabello.
- Altura.
Así, entre los valores tendremos:
import numpy
Gender = numpy.array(['Man', 'Woman'])
EyeColor = numpy.array(['Black','Brown','Green','Blue','Yellow','Grey'])
SkinColor = numpy.array(['Black','White','Yellow','Purple','Green'])
HairColor = numpy.array(['Black,','Brown','White','Grey','Blonde','Red'])
Height = numpy.array(['Tall', 'Average Height', 'Small'])
Y ahora, solo basta crear una función que usaremos siempre que queramos crear un personaje. Recordamos que la creamos dentro de una función para que no tengamos que escribir todo el código de nuevo si queremos hacer esta operación.
import numpy
Gender = numpy.array(['Man', 'Woman'])
EyeColor = numpy.array(['Black','Brown','Green','Blue','Yellow','Grey'])
SkinColor = numpy.array(['Black','White','Yellow','Purple','Green'])
HairColor = numpy.array(['Black,','Brown','White','Grey','Blonde','Red'])
Height = numpy.array(['Tall', 'Average Height', 'Small'])
def generate_character(Gender,EyeColor,SkinColor,HairColor,Height):
sex = numpy.random.choice(Gender)
eye = numpy.random.choice(EyeColor)
skin = numpy.random.choice(SkinColor)
hair = numpy.random.choice(HairColor)
length = numpy.random.choice(Height)
print(f'Your character is a {sex} with {skin} skin, {eye} eyes, {hair} hair and {length} stature')
generate_character(Gender,EyeColor,SkinColor,HairColor,Height)
Aquí, podemos ver que usaremos como parámetros los arreglos creados anteriormente, y luego, almacenaremos los valores aleatorios en variables por medio del uso de random.choice.
Finalmente, imprimimos el resultado al final de la función y la ejecutamos. Así, al correr el programa:
>>> Your character is a Woman with Green skin, Grey eyes, Blonde hair and Average Height stature
Ejemplo 2
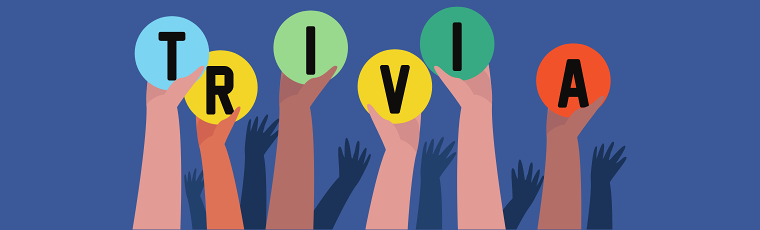
Shoutout to East Lansing Public Library
Ahora, volvemos a los ejercicios de trivia. Donde se nos pide crear un pequeño juego con una serie de preguntas que tendrán la siguiente condición: Si respondes correctamente, automáticamente tendrás otra pregunta y si respondes mal, se acaba el juego.
Sin embargo, lo que dará el encanto de numpy será el hecho de que usaremos dos arreglos para llevar esto a cabo, uno con las preguntas y otro con las respuestas:
import numpy
Questions = numpy.array(['What is the Capital of Greece?', 'What\'s the name of James Bond\'s boss?', 'Who won the 2010 world cup?', 'What\'s the most sold Toyota car?'])
Answers = ['Athens','M','Spain','Corolla']
En este caso, solo questions será un array con numpy debido a que es a este a quien aplicaremos random.choice para obtener la pregunta al usar, luego buscando el índice respectivo en el arreglo de Answers.
Ahora, debemos de crear una función que nos muestre alguna de las preguntas en questions y nos la muestre en forma de string, para luego tomar el índice y buscarlo en answers. Para esto, hacemos lo siguiente:
import numpy
Questions = numpy.array(['What is the Capital of Greece?', 'What\'s the name of James Bond\'s boss?', 'Who won the 2010 world cup?', 'What\'s the most sold Toyota car?'])
Answers = ['Athens','M','Spain','Corolla']
def question_answer(Questions, Answers):
array = numpy.random.choice(Questions)
print(str(array))
index = Questions.tolist().index(str(array))
Como podemos ver, lo que debemos para obtener el índice es convertir el arreglo de numpy a una lista con el método tolist() y luego usar otro método propio de las listas para buscar índices de acuerdo a un valor: index(), donde colocamos como parámetro el valor en string de random.choice.
Ahora que tenemos el índice, nos falta hacer dos cosas: Dar al usuario la opción de responder y verificar si la información que introduce es correcta. Para esto, creamos una variable con input() para que nos de una respuesta.
Luego, creamos un condicional para verificar si la respuesta es igual al valor de la respuesta en la misma posición que colocamos en la pregunta (La respuesta correcta), y si se cumple, damos un mensaje de '¡Correcto!' y rodamos la siguiente pregunta. En caso de que no, acabamos el programa:
import numpy
Questions = numpy.array(['What is the Capital of Greece?', 'What\'s the name of James Bond\'s boss?', 'Who won the 2010 world cup?', 'What\'s the most sold Toyota car?'])
Answers = ['Athens','M','Spain','Corolla']
def question_answer(Questions, Answers):
array = numpy.random.choice(Questions)
print(str(array))
index = Questions.tolist().index(str(array))
to_answ = str(input(''))
if to_answ == str(Answers[index]) or to_answ == str(Answers[index]).lower():
print('Correct!')
question_answer(Questions, Answers)
else:
print('Wrong!')
question_answer(Questions, Answers)
Y si ejecutamos:
What's the name of James Bond's boss?
M
Correct!
What's the most sold Toyota car?
Corolla
Correct!
What is the Capital of Greece?
Rome
Wrong!
Ejemplo 3
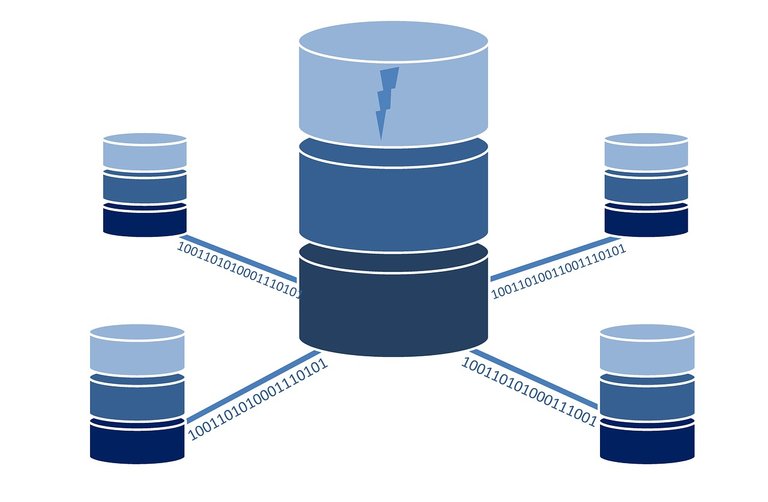
Shoutout to Needpix.com
Finalmente, haremos la simulación de una base de datos, donde agregamos el nombre, la edad y la nacionalidad de cuantas personas quiera el usuario.
Para hacer esto posible, usaremos un método propio de las listas y arreglos en Python, que se puede usar de igual manera con la librería de numpy. Este es append:
Así, lo primero que debemos de hacer es preguntarle sus datos al usuario. Antes de esto, creamos el primer arreglo, uno de dos dimensiones, donde cada arreglo de una dimensión dentro de este contendrá los datos de los usuarios.
import numpy
total_list = numpy.array([['Name','Age','Country']],ndmin=2)
Entonces podremos crear una función donde usemos este arreglo como parámetro y por medio de variables con input, vayamos ingresando los variables en arreglos de dos dimensiones con solo un elemento que agregaremos a nuestra total_list:
def add_person(total_list):
name = str(input('Insert your name'))
age = int(input('Insert your age'))
country = str(input('Where are you from?'))
person_id = numpy.array([[name,age,country]])
Luego, nos falta añadir este nuevo arreglo dentro de total_list. Esto lo hacemos con append, tomando como parámetros:
- El arreglo al que agregaremos los nuevos elementos (En este caso total_list).
- El arreglo con los elementos que agregaremos (Para nosotros person_id).
- El eje en el que se agregarán. Ya que queremos agregar un arreglo de una dimensión (Que está dentro del de segunda dimension), colocamos el eje 0. Así, tendremos:
total_list = numpy.append(total_list, person_id, axis=0)
Entonces, solo nos quedará preguntar al usuario si quiere agregar una nueva id. Para esto, creamos una variable con input para yes/no y dependiendo de lo que obtengamos repetimos la función o mostramos el total de todos los usuarios. Finalmente, llamamos a la función con total_list como parámetro:
import numpy
total_list = numpy.array([['Name','Age','Country']],ndmin=2)
def add_person(total_list):
name = str(input('Insert your name'))
age = int(input('Insert your age'))
country = str(input('Where are you from?'))
person_id = numpy.array([[name,age,country]])
total_list = numpy.append(total_list, person_id, axis=0)
repeat = str(input('Do you want to add another person?'))
if repeat == 'yes' or repeat == 'Yes':
add_person(total_list)
else:
print(total_list)
add_person(total_list)
Y al ejecutar el programa:
>>>
Insert your nameJohn
Insert your age28
Where are you from?Us
Do you want to add another person?Yes
Insert your nameMarc
Insert your age29
Where are you from?France
Do you want to add another person?Yes
Insert your nameGunther
Insert your age30
Where are you from?Germany
Do you want to add another person?Yes
Insert your nameJames
Insert your age30
Where are you from?England
Do you want to add another person?No
[['Name' 'Age' 'Country']
['John' '28' 'Us']
['Marc' '29' 'France']
['Gunther' '30' 'Germany']
['James' '30' 'England']]
Como podrás ver, el uso de arreglos y el módulo random con numpy nos brinda un nuevo rango de posibilidades que podemos aprovechar para crear infinidad de programas. Si así son estos ejemplos sencillos, imagina lo que puedes hacer con este módulo.
Si crees que los programas podrían diseñarse de mejor manera o quieres agregar elementos como try/except para evitar errores o un grado mayor de complejidad, adelante. Me siento orgulloso por todos los que han llegado hasta este punto.
Nos veremos en la siguiente edición de Coding Basics, continuando con NumPy.
Thanks for sharing your coding tutorials!
Reminds me of my time as a student, trying to understand coding :p
Great article!
Thanks!
They say the best way to prove you understand something is to share It with others. I hope you could have gotten value from It.
Have a blessed day!
It's definitely true! Understanding (or thinking you do so) is step 1 but explaining it to others is the next step! Especially doing so in easy words is a great achievement!
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.