NumPy Part 2: More Array Operations / NumPy Parte 2: Más operaciones con Arreglos - Coding Basics #32
More NumPy
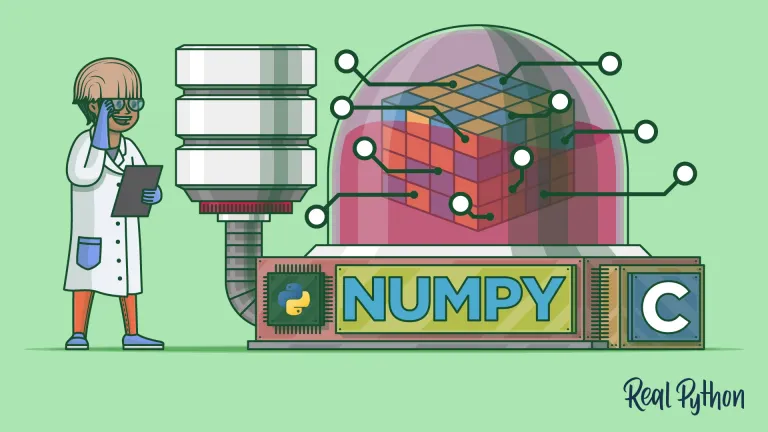
Shoutout to Real Python
In this article you will find:
- Introduction
- Reshape
- Concatenate
- Separate Arrays
- Sort
In the last edition of Coding Basics we introduced one of the most used libraries in Python, NumPy. In addition to hinting at their potential, we were able to create arrays and perform some basic operations with them.
However, we skipped over a few of these, which are equally important to someone who may need them for their project. This is why in the next article we will see more operations with arrays in NumPy, so that we do not leave any stone unturned regarding the power of numPy.
Reshape
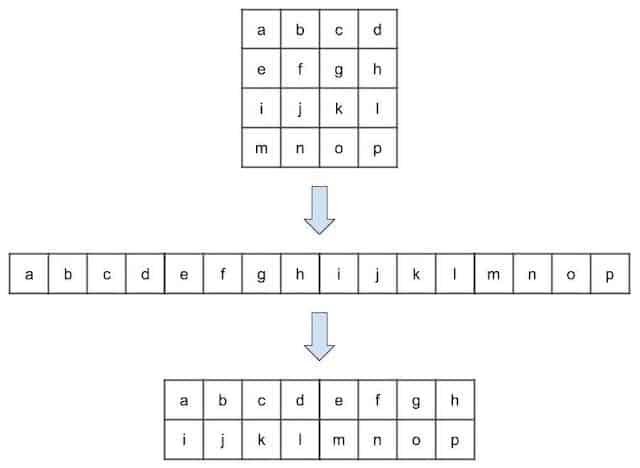
Shoutout to Sparrow Computing
If we go back to the previous article, we know that with Shape we could know the shape of an array, its number of dimensions and the number of elements in each one. For example, if we have a two-dimensional array:
import numpy
newarray = numpy.array([[1,2,3],[4,5,6]])
print(newarray.shape)
>>>
(23)
Thus, we know that in these two dimensions, the first (the list that contains the other list) has 2 elements, while the second (the lists that are inside the first list) has 3 elements.
Now, if we wanted to change the dimensions of an array, for example:
import numpy
newarray = numpy.array([1,2,3,4,5,6,7,8,9,10,11,12])
We just have to use reshape, as long as it fits the elements of each dimension. In this case, if we want two dimensions, one with 6 elements and another with 2:
newarray2 = newarray.reshape(6,2)
print(newarray2)
>>>
[[ 1 2]
[ 3. 4]
[5 6]
[7 8]
[9 10]
[11 12]]
This can be carried out for any number of dimensions. We only have to verify that we are within the range and that a number of parameters equal to the dimensions are placed. If, for example, we wanted to put something out of range in newarray, we would get the following:
newarray2 = newarray.reshape(6,3)
print(newarray2)
>>>
Traceback (most recent call last):
File "C:\Users\rogelio\Desktop\ProyectosPython\exampleforpy.py", line 5, in <module>
newarray2 = newarray.reshape(6,3)
ValueError: cannot reshape array of size 12 into shape (6,3)
We get a valueerror since it is impossible to transform this array from 12 elements into one of 6x3 = 18 elements, we would be missing 6 elements to carry out this.
Concatenate and Stack
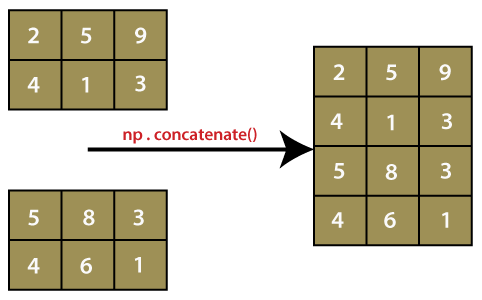
Shoutout to Javatpoint
We already know what we should do when we want to resize an array in NumPy. However, we can also perform a broader range of functions equaling and even surpassing Python's own arrangements in some respects.
If you want to join two numpy arrays, you just have to resort to the concatenate method, which allows you to join the contents of both arrays into one, with the dimensions that we specify, for example:
import numpy
array1 = numpy.array([1,2,3])
array2 = numpy.array([4,5,6])
newarray = numpy.concatenate(array1,array2)
print(newarray)
>>> [1 2 3 4 5 6]
However, as we can see, the content of both arrays were merged into a single-dimensional one. This is because if we want to change the dimensions in which the elements of the arrays are joined, we must add an additional parameter called axis, referring to the axis of the dimension in which it is located (e.g. x,y for two dimensions) .
Thus, in the first example the default value of 0 was taken, which indicates a single axis or dimension. If we want to join two two-dimensional arrays:
import numpy
array1 = numpy.array([1,2,3], [4,5,6])
array2 = numpy.array([7,8,9], [10,11,12])
newarray = numpy.concatenate(array1,array2, axis=1)
print(newarray)
>>>
[[1 2 3 7 8 9]
[4 5 6 10 11 12]]
We must always take into account the dimensions of the arrangements to determine the maximum number of dimensions of the resulting arrangement. If we used an axis of 2 in this case, we would have the following error:
Traceback (most recent call last):
File "./prog.py", line 7, in <module>
File "<__array_function__ internals>", line 5, in concatenate
numpy.AxisError: axis 2 is out of bounds for array of dimension 2
Likewise, if we want to join two arrays and automatically create a new dimension to store them, we use the stack command:
import numpy
arr1 = numpy.array([1, 2, 3])
arr2 = numpy.array([4, 5, 6])
arr = numpy.stack((arr1, arr2))
print(arr)
>>>
[[1 2 3]
[4 5 6]]
Note: If in this case we set axis=1, we would have three arrays as elements of our two-dimensional array, joining the elements in the specific positions of each one:
import numpy
arr1 = numpy.array([1, 2, 3])
arr2 = numpy.array([4, 5, 6])
arr = numpy.stack((arr1, arr2, axis=1))
print(arr)
>>>
[[1 4]
[2 5]
[3 6]]
Separate arrays
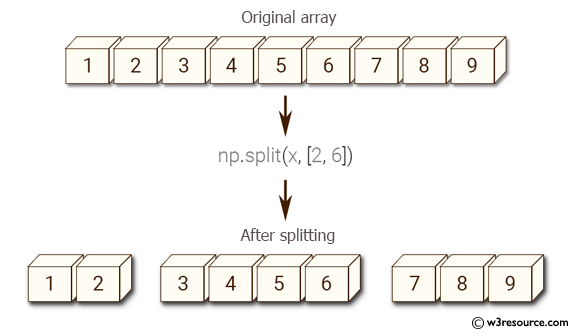
Shoutout to w3resource
The opposite of the operations to join arrays, with the use of a method called array_split we can separate an array into multiple arrays. The only thing we must enter as parameters is the name of the array and the number of arrays into which we want to separate, thus obtaining a list with the specified number of arrays:
import numpy
arr = numpy.array([1, 2, 3, 4, 5, 6, 7, 8])
newarr = numpy.array_split(arr, 4)
print(newarr)
>>> [array([1, 2]), array([3, 4]), array([5, 6]), array([7, 8])]
So, just like any list, we can access these arrays by simply indicating the index of each one.
print(newarr[0])
>>>
[1 2]
This procedure will be repeated for arrangements of any dimension. If, for example, we work with a two-dimensional array:
import numpy
arr = numpy.array([1, 2], [3, 4], [5, 6], [7, 8], [9,10], [11,12], [13,14], [15 ,16]])
newarr = numpy.array_split(arr, 4)
print(newarr)
>>>
[array([[1, 2],
[3, 4]]), array([[5, 6],
[7, 8]]), array([[ 9, 10],
[11, 12]]), array([[ 13, 14],[15, 16]]), ]
Sort
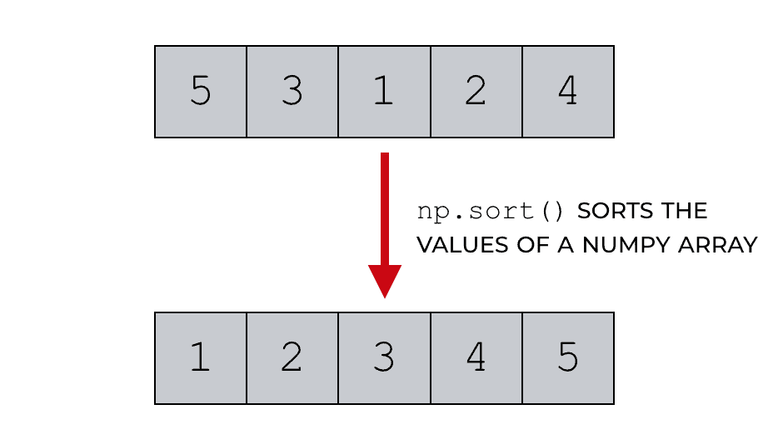
Shoutout to Sharp Sight
As we know when working with arrays, in some cases we will need to organize them according to an incremental order, whether alphabetical, numerical or even Boolean. To do this, we use the sort function, which is also no stranger to numpy.
If we want to sort an array of 1 dimension from smallest to largest:
import numpy
arr = numpy.array([7,9,6,10])
print(numpy.sort(arr))
>>> [6 7 9 10]
If used with strings, it will only sort them alphabetically:
import numpy
arr = numpy.array(['cross', 'atom', 'deacon'])
print(numpy.sort(arr))
>>> ['atom', 'cross', 'deacon']
Now, if we use sort with arrays of larger dimensions, what it will do is continue ordering the elements within the arrays that make up the larger array:
import numpy
arr = numpy.array([[5,8,6], [5, 0, 1], [2,1,3]])
print(numpy.sort(arr))
>>>
[[5 6 8]
[0 1 5]
[1 2 3]]
As we can see, the range of operations that we can perform with arrays in numpy is really extensive, matching that of common arrays in Python. However, the potential of this beautiful bookstore does not stop there.
This is why in the next edition of Coding Basics, we will see some of the applications that numpy can have to make our lives better in endless fields, all so that you can expand the tools at your disposal when tackling different projects. That being said, I wish you the best in your Python learning journey.
Más NumPy
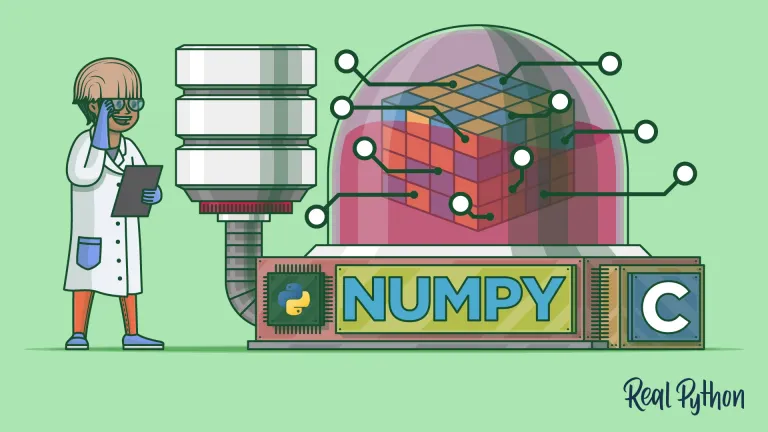
Shoutout to Real Python
En este artículo encontrarás:
- Introducción
- Reshape
- Concatenate
- Separar Arreglos
- Sort
En la edición pasada de Coding Basics hicimos una introducción a una de las librerías más utilizadas en Python, NumPy. Además de hacer alusión a su potencial, pudimos crear arreglos y realizar algunas operaciones básicas con estos.
Sin embargo, nos saltamos algunas de estas, las cuales son de igual importancia para alguien que puede necesitarlas para su proyecto. Es por esto, que en el siguiente artículo veremos más operaciones con arreglos en NumPy, eso para que no dejemos ninguna piedra sin levantar en cuanto al poder de numPy.
Reshape
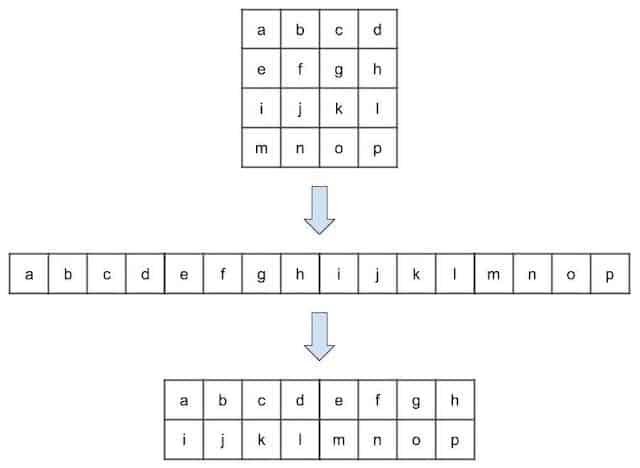
Shoutout to Sparrow Computing
Si volvemos al artículo anterior, sabemos que con Shape podíamos conocer la forma de un arreglo, su número de dimensiones y la cantidad de elementos en cada una. Por ejemplo, si tenemos un arreglo de dos dimensiones:
import numpy
newarray = numpy.array([[1,2,3],[4,5,6]])
print(newarray.shape)
>>>
(2, 3)
Así, sabemos que en estas dos dimensiones, la primera (la lista que contiene a la otra lista), tiene 2 elementos, mientras que la segunda (Las listas que están dentro de la primera lista) tiene 3 elementos.
Ahora bien, si quisieramos cambiar las dimensiones de un arreglo, por ejemplo:
import numpy
newarray = numpy.array([1,2,3,4,5,6,7,8,9,10,11,12])
Solo tenemos que usar reshape, siempre y cuando se ajuste a los elementos de cada dimensión. En este caso, si queremos dos dimensiones, una de 6 elementos y otra de 2:
newarray2 = newarray.reshape(6,2)
print(newarray2)
>>>
[[ 1 2]
[ 3 4]
[ 5 6]
[ 7 8]
[ 9 10]
[11 12]]
Esto puede llevarse a cabo para cualquier número de dimensiones. Solo debemos verificar que estemos dentro del rango y que se coloque un número de parámetros igual al de dimensiones. Si por ejemplo, quisieramos colocar algo fuera de rango en newarray, obtendremos lo siguiente:
newarray2 = newarray.reshape(6,3)
print(newarray2)
>>>
Traceback (most recent call last):
File "C:\Users\rogelio\Desktop\ProyectosPython\exampleforpy.py", line 5, in <module>
newarray2 = newarray.reshape(6,3)
ValueError: cannot reshape array of size 12 into shape (6,3)
Nos aparece un valueerror ya que es imposible transformar este arreglo, de 12 elementos en uno de 6x3 = 18 elementos, nos faltarían 6 elementos para llevar a cabo esto.
Concatenate y Stack
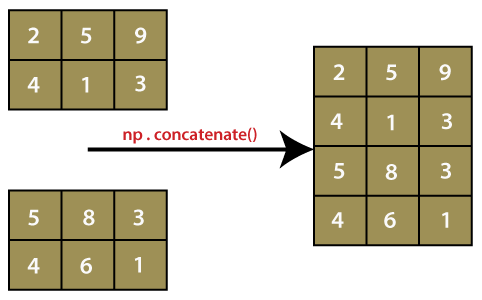
Shoutout to Javatpoint
Ya sabemos que debemos de hacer cuando queremos redimensionar un arreglo en NumPy. Sin embargo, también podemos llevar a cabo un rango más amplio de funciones igualando e incluso superando en algunos aspectos a los arreglos propios de Python.
Si quieres unir dos arreglos de numpy, solo debes de recurrir al método concatenate, el cual te permite unir el contenido de ambos arrays en uno, con las dimensiones que nosotros especifiquemos, por ejemplo:
import numpy
array1 = numpy.array([1,2,3])
array2 = numpy.array([4,5,6])
newarray = numpy.concatenate(array1,array2)
print(newarray)
>>> [1 2 3 4 5 6]
Sin embargo, como podemos ver, el contenido de ambos arreglos se unieron en uno de una sola dimensión. Esto se debe a que si queremos cambiar las dimensiones en que se unen los elementos de los arreglos, debemos de agregar un parámetro adicional llamado axis, haciendo referencia al eje de la dimensión en que se encuentra (ej: x,y para dos dimensiones).
Así, en el primer ejemplo se tomó el valor por defecto de 0, que nos indica un solo eje o dimensión. Si queremos unir dos arreglos de dos dimensiones:
import numpy
array1 = numpy.array([1,2,3], [4,5,6])
array2 = numpy.array([7,8,9], [10,11,12])
newarray = numpy.concatenate(array1,array2, axis=1)
print(newarray)
>>>
[[1 2 3 7 8 9]
[4 5 6 10 11 12]]
Siempre debemos de tener en cuenta las dimensiones de los arreglos para determinar la cantidad máxima de dimensiones del arreglo resultante. Si usaramos un axis de 2 en este caso, tendríamos el siguiente error:
Traceback (most recent call last):
File "./prog.py", line 7, in <module>
File "<__array_function__ internals>", line 5, in concatenate
numpy.AxisError: axis 2 is out of bounds for array of dimension 2
De igual forma, si queremos unir dos arreglos y crear automáticamente una nueva dimensión para almacenarlos, usamos el comando stack:
import numpy
arr1 = numpy.array([1, 2, 3])
arr2 = numpy.array([4, 5, 6])
arr = numpy.stack((arr1, arr2))
print(arr)
>>>
[[1 2 3]
[4 5 6]]
Nota: Si en este caso colocaramos el axis=1, tendríamos tres arreglos como elementos de nuestro arreglo de dos dimensiones, uniendo los elementos en las posiciones específicas de cada uno:
import numpy
arr1 = numpy.array([1, 2, 3])
arr2 = numpy.array([4, 5, 6])
arr = numpy.stack((arr1, arr2, axis=1))
print(arr)
>>>
[[1 4]
[2 5]
[3 6]]
Separar arreglos
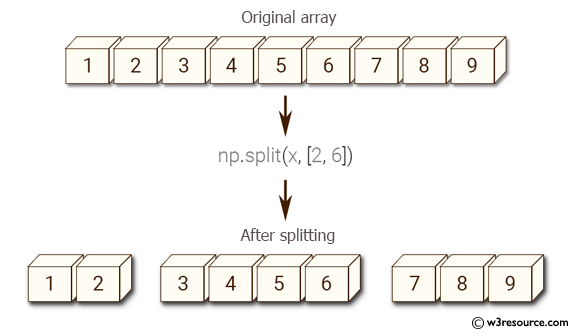
Shoutout to w3resource
Lo opuesto a las operaciones para unir arreglos, con el uso de un método llamado array_split podremos separar un arreglo en múltiples arreglos. Lo único que debemos de ingresar como parámetros son el nombre del arreglo y la cantidad de arreglos en que se desea separar, obteniendo así una lista con la cantidad de arreglos especificada:
import numpy
arr = numpy.array([1, 2, 3, 4, 5, 6, 7, 8])
newarr = numpy.array_split(arr, 4)
print(newarr)
>>> [array([1, 2]), array([3, 4]), array([5, 6]), array([7, 8])]
Entonces, al igual que en cualquier lista, podemos acceder a estos arreglos con solo indicar el índice de cada uno.
print(newarr[0])
>>>
[1 2]
Este procedimiento se repetirá para arreglos de cualquier dimensión. Si por ejemplo, trabajamos con un arreglo de dos dimensiones:
import numpy
arr = numpy.array([1, 2], [3, 4], [5, 6], [7, 8], [9,10], [11,12], [13,14], [15,16]])
newarr = numpy.array_split(arr, 4)
print(newarr)
>>>
[array([[1, 2],
[3, 4]]), array([[5, 6],
[7, 8]]), array([[ 9, 10],
[11, 12]]), array([[ 13, 14],[15, 16]]), ]
Sort
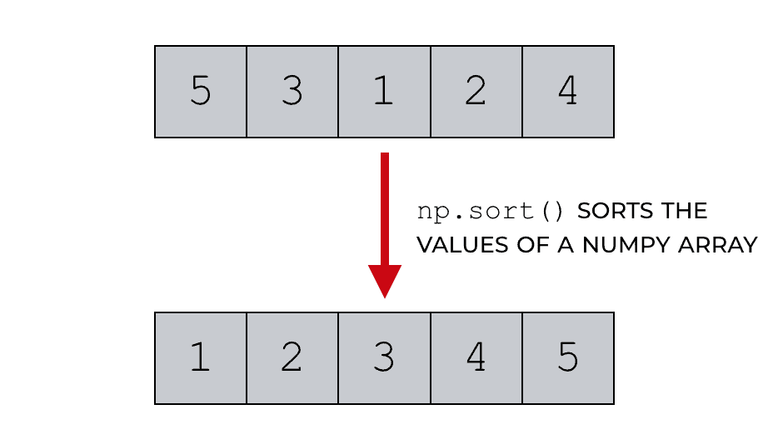
Shoutout to Sharp Sight
Como sabemos al trabajar con arreglos, en algunos casos tendremos la necesidad de organizarlos de acuerdo a un orden incremental, ya sea alfabético, numérico o incluso booleano. Para llevar a cabo esto, hacemos uso de la función sort, que tampoco es extraña a numpy.
Si queremos sortear un arreglo de 1 dimensión de menor a mayor:
import numpy
arr = numpy.array([7,9,6,10])
print(numpy.sort(arr))
>>> [6 7 9 10]
Si se usa con strings, solo las ordenará alfabéticamente:
import numpy
arr = numpy.array(['cross', 'atom', 'deacon'])
print(numpy.sort(arr))
>>> ['atom', 'cross', 'deacon']
Ahora, si usamos sort con arreglos de dimensiones mayores, lo que hará es seguir ordenando los elementos dentro de los arreglos que conforman al arreglo mayor:
import numpy
arr = numpy.array([[5,8,6], [5, 0, 1], [2,1,3]])
print(numpy.sort(arr))
>>>
[[5 6 8]
[0 1 5]
[1 2 3]]
Como podemos ver, el rango de operaciones que podemos realizar con arreglos en numpy es realmente extenso, equiparándose al de los arreglos comunes en Python. Sin embargo, el potencial de esta hermosa librería no se detiene en esto.
Es por esto, que en la siguiente edición de Coding Basics, veremos algunas de las aplicaciones que puede tener numpy para hacernos la vida mejor en un sinfin de campos, todo para que puedas ampliar las herramientas a tu disposición al combatir distintos proyectos. Dicho esto, te deseo lo mejor en tu viaje de aprendizaje de Python.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.