datetime Module / Módulo datetime - Coding Basics #17
Can you tell me the time?
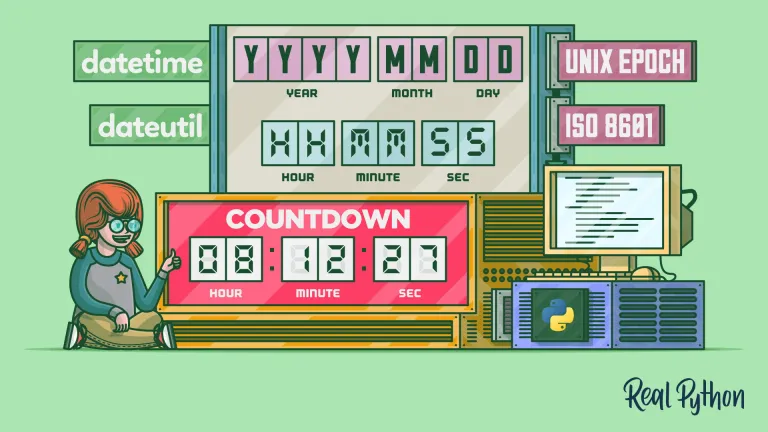
Shoutout to Real Python
In this article you'll find:
- Introduction
- What is the Date Module?
- What does datetime look like in code?
- Portraying Dates as Strings
- Creating objects of the class Date
- A little example
Now that we know the modules, we can begin to know the true face of Python and the endless number of built-in modules within this programming language.
The first of these is a relatively simple but very important one when we want to represent the values of our variables in the form of dates: Dates.
So, if you want to see how this module works and how you can apply it to give a more technical detail to your projects, keep on reading.
What is the Date Module?
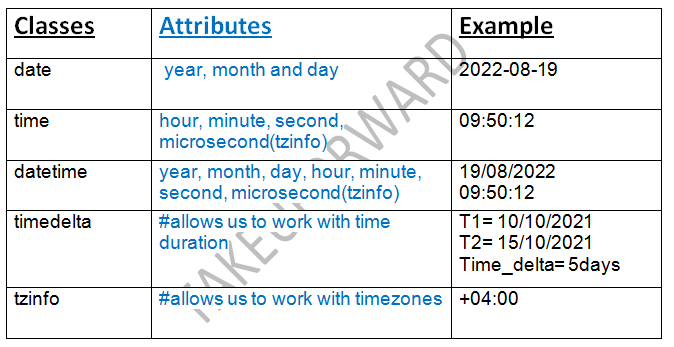
Shoutout to takeUforward
ate is an internal module within Python that is used when you want to represent values in terms of date. If we wanted to represent date values in Python without importing any library, we cannot use a specific DataType.
For this we would have to apply a manual and tedious process, which can even get complicated if we want to observe different dates.
That is why, in order to avoid this problem, we use the Datetime module, which allows us to work with objects of the datetime class to view any date in a proper format.
Now that we know this, how does the use of Datetime look like in code?
What does datetime look like in code?
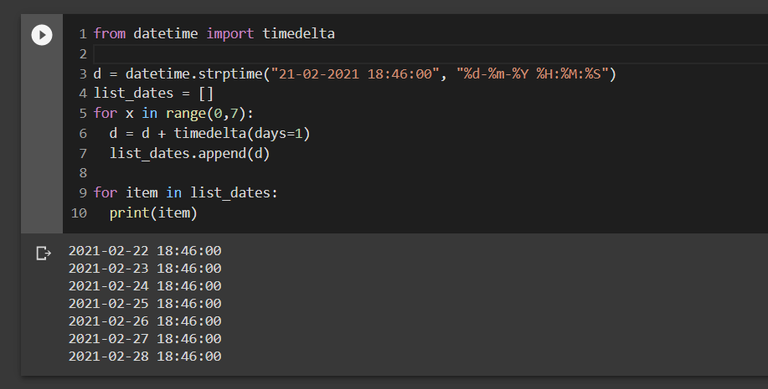
Shoutout to freeCodeCamp
Being a module, the first action will be to insert the import instruction:
import datetime
Now, everything will depend on the method we want to execute. If, for example, we want to see the current date, we only have to use now:
x = datetime.datetime.now()
print(x)
>>>
2023-08-29 08:13:38.666779
We don't necessarily have to see the whole date, if we want, we can use other methods of the datetime module to see specific parts of it. For example, if we want to see only the year, we will use year:
print(x.year)
>>> 2023
If we want to see the month or the day, we use month and day.
print(x.month)
print(x.day)
>>> 8
>>> 29
Portraying Dates as Strings
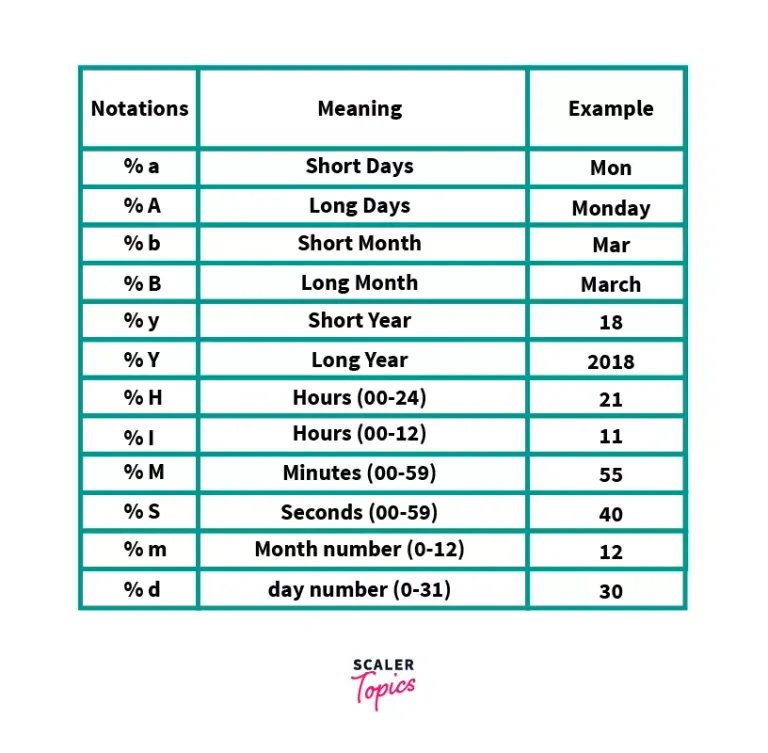
Shoutout to Scaler
So far, the use of Dates seems perfect, but what if we want to see the date in terms of days of the week or see the name of the month?
For this we use another method, this one is called strftime, which will allow to give a specific format to the date. For this, we only have to enter a parameter, which according to the value it has, will allow us to see the dates in terms of:
- %A: Days of the week
- %B: Name of the month
- %H: Hours from 0 to 23
- %I: Hours from 0 to 12
- %p: AM/PM
- %M: Minute from 0 to 59
- %S: Seconds from 0 to 59
- %Z: Time zone.
So, trying some of these:
import datetime
x = datetime.datetime.now()
print(x.strftime("%B"))
>>> August
print(x.strftime("%H"))
>>> 8
print(x.strftime(%M))
>>> 32
Creating Date class objects
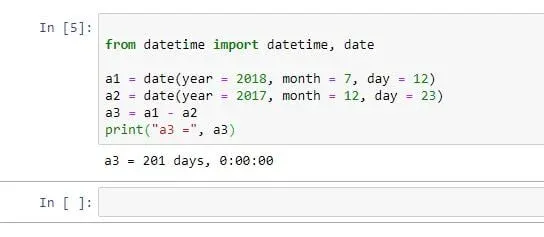
Shoutout to Technicalblog.in
Until now we have only worked with objects that show us the present date. However, if we would like to use a date that we can choose, we will only have to create an object of the Date class.
To do this, just use the constructor of the datetime class and enter the year, month and day as parameters. Looking at an example:
import datetime
date = datetime.datetime(2023, 8, 23)
print(date)
>>> 2023-08-23 00:00:00
So we will have an object with a date value specified by us.
A little example
To finish explaining the applications of datetime, we can use a cycle to determine the holidays within a month. In this case, if we want to determine the holidays in December.
The first thing we would have to do is to create the object that will have the date of December 1st, 2023:
import datetime
x = datetime.datetime(2023,12,1)
Then, by means of the use of a for loop and the range instruction, we go through the days of the month of December to finally verify that, if the month is 12 and the date is the indicated one (25 and 31), it is a holiday:
for i in range(1,32):
x = x.replace(day=i)
if x.month == 12 and x.day == 25:
print("It's Christmas")
if x.month == 12 and x.day == 31:
print("It's the day before New Year")
else:
print("Just Another Day")
Note: Remember that range creates a range of values to run from the first value until the condition that we are in a position less than or equal to the second parameter is met. This is why we use 32 and not 31.
Note: We use the replace method because with this method we can create a new object based on the previous x. If we simply try to use x.day = i, we will get the following error:
AttributeError: attribute 'day' of 'datetime.datetime' objects is not writable
Thus, when executing the program:
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
It's Christmas
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
It's the day before New Year
The datetime module is a very practical tool when you want to work with specific times, allowing you to create timers, calendars or reminders.
Once you have read this article, you will understand and be able to use this module very easily. In the next chapter we will increase the degree of complexity by using a more advanced module. Until then:
¿Puedes decirme la hora?
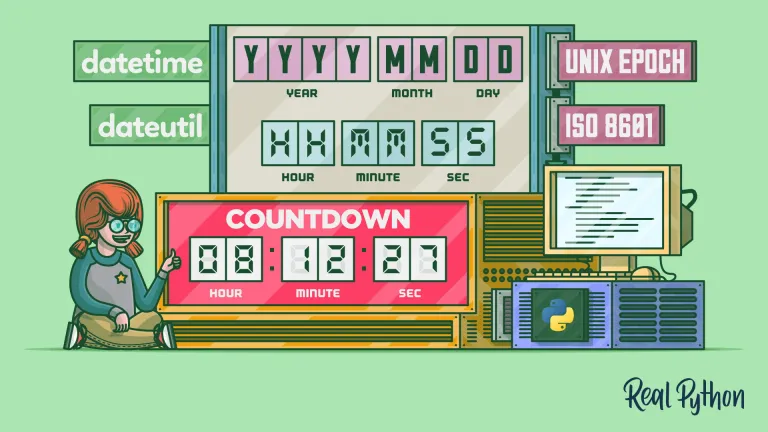
Shoutout to Real Python
En este artículo encontrarás:
- Introducción
- ¿Que es el módulo Date?
- ¿Cómo se ve datetime en código?
- Representando fechas como strings
- Creando objetos de la clase Date
- Un pequeño ejemplo
Ahora que conocemos los módulos, podemos empezar a conocer la verdadera cara de Python y el sin fin de módulos built-in dentro de este lenguaje de programación.
El prmero de estos es uno relativamente sencillo pero de gran importancia cuando queremos representar los valores de nuestras variables en forma de fechas: Dates.
Así, si quieres ver como este módulo funciona y como puedes aplicarlo para darle un detalle más técnico a tus proyectos, sigue leyendo
¿Qué es el módulo Date?
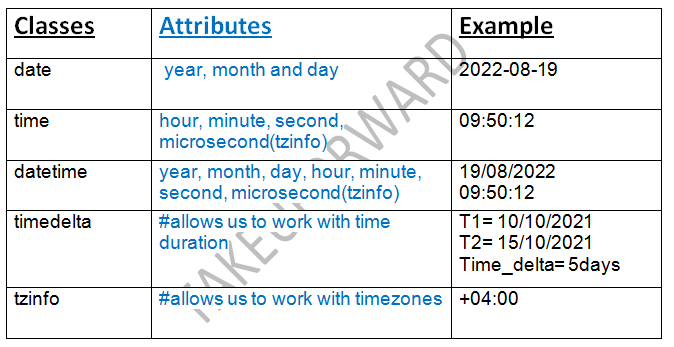
Shoutout to takeUforward
Date es un módulo interno dentro de Python que se usa cuando se quieren representar valores en términos de fecha. Si quisieramos representar el valor de fechas en Python sin importar alguna librería, no podemos usar un DataType específico.
Para esto tendríamos que aplicar un proceso manual y tedioso, que incluso puede complicarse si se quieren observar distintas fechas.
Es por eso, que en orden evitarnos este problema, usamos el módulo Datetime, que nos permite trabajar con objetos de la clase datetime para ver cualquier fecha en un formato adecuado.
Ahora que sabemos esto, ¿Cómo se ve el uso de Datetime en código?
¿Como se ve datetime en código?
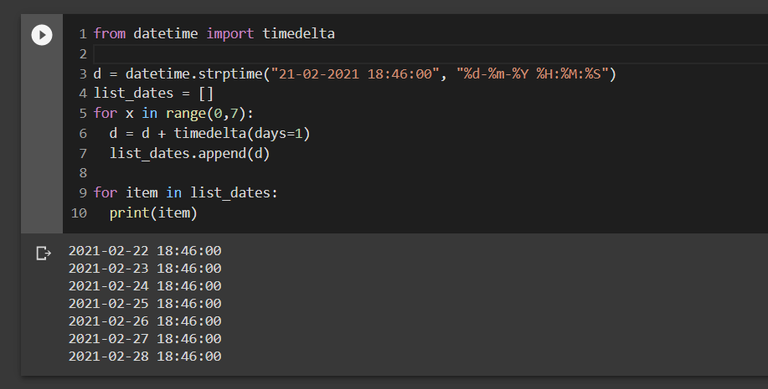
Shoutout to freeCodeCamp
Al ser un módulo, la primera acción será insertar la instrucción import:
import datetime
Ahora, todo dependerá del método que queramos ejecutar. Si por ejemplo, queremos ver la fecha actual, solo tenemos que usar now:
x = datetime.datetime.now()
print(x)
>>>
2023-08-29 08:13:38.666779
No necesariamente tenemos que ver la totalidad de la fecha, si queremos, podemos usar otros métodos propios del módulo datetime para ver partes específicas de esta. Por ejemplo, si queremos ver solo el año, usaremos year:
print(x.year)
>>> 2023
Si queremos ver el mes o el día, usamos month y day.
print(x.month)
print(x.day)
>>> 8
>>> 29
Representando Fechas como Strings
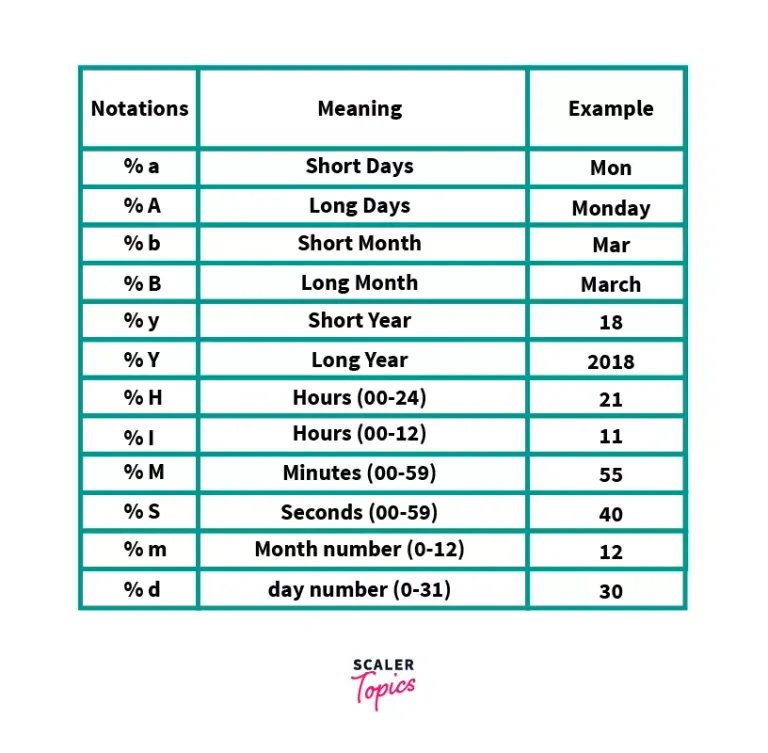
Shoutout to Scaler
Hasta ahora, el uso de Dates parece perfecto, pero ¿Qué pasa si queremos ver la fecha en términos de días de la semana o ver el nombre del mes?
Para esto usamos otro método, este se llama strftime, el cual permitirá dar un formato específico a la fecha. Para esto, solo debemos introducir un parámetro, que de acuerdo al valor que tenga, nos permitirá ver las fechas en términos de:
- %A: Días de la semana
- %B: Nombre del mes
- %H: Horas de 0 a 23
- %I: Horas de 0 a 12
- %p: AM/PM
- %M: Minuto de 0 a 59
- %S: Secundo de 0 a 59
- %Z: Zona de tiempo.
Así, probando algunas de estas:
import datetime
x = datetime.datetime.now()
print(x.strftime("%B"))
>>> August
print(x.strftime("%H"))
>>> 8
print(x.strftime(%M))
>>> 32
Creando objetos de la clase Date
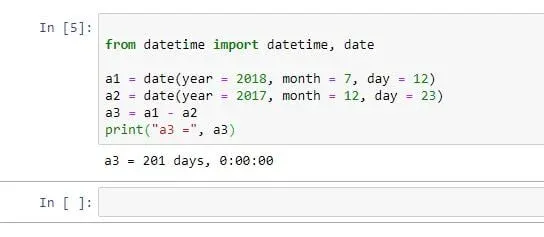
Shoutout to Technicalblog.in
Hasta ahora solo hemos trabajado con objetos que nos muestren la fecha presente. Sin embargo, si quisieramos usar una fecha que nosotros podamos escoger, solo tendremos que crear un objeto de la clase Date.
Para hacer esto, solo basta con usar el constructor de la clase datetime e introducir los datos de año, mes y día como parámetros. Observando un ejemplo:
import datetime
date = datetime.datetime(2023, 8, 23)
print(date)
>>> 2023-08-23 00:00:00
Con lo que tendremos un objeto con valor de fecha específicado por nosotros.
Un pequeño ejemplo
Para terminar de explicar las aplicaciones de datetime, podemos usar un ciclo para determinar las festividades dentro de un mes. En este caso, si queremos determinar las festividades en Diciembre.
Lo primero que tendríamos que hacer es crear el objeto que tendrá la fecha del primero de diciembre de 2023:
import datetime
x = datetime.datetime(2023,12,1)
Entonces, por medio del uso de un ciclo for y la instrucción range, recorremos los días del mes de diciembre para finalmente verificar que, si el mes es 12 y la fecha es la indicada (25 y 31), es un día festivo:
for i in range(1,32):
x = x.replace(day=i)
if x.month == 12 and x.day == 25:
print("It's Christmas")
elif x.month == 12 and x.day == 31:
print("It's the day before New Year")
else:
print("Just Another Day")
Nota: Recordamos que range crea un rango de valores a correr que van desde el primer valor hasta que se cumpla la condición de que nos encontremos en una posición menor o igual al segundo parámetro. Es por esto que usamos 32 y no 31.
Nota: Usamos el método replace ya que con este podemos crear un nuevo objeto basado en el x anterior. Si simplemente trataramos de usar x.day = i, nos saltará el siguiente error:
AttributeError: attribute 'day' of 'datetime.datetime' objects is not writable
Así, al ejecutar el programa:
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
It's Christmas
Just Another Day
Just Another Day
Just Another Day
Just Another Day
Just Another Day
It's the day before New Year
El módulo datetime es una herramienta muy práctica cuando queremos trabajar con horas específicas, permitiéndonos crear timers, calendarios o recordatorios.
Una vez leido este artículo, comprenderás y podrás usar con suma facilidad este módulo. En el siguiente capítulo aumentaremos el grado de complejidad al usar un módulo más avanzado. Hasta entonces:
Do people still make use of data module
As long as you need to set dates in your apps, datetime will be used. However, for programs that handle countdowns and timers, people tend to use the time module.
Thanks for reading!
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.