Class Polymorphism / Polimorfismo de clases - Coding Basics #12 [EN/ES]
class newClass(Part2)
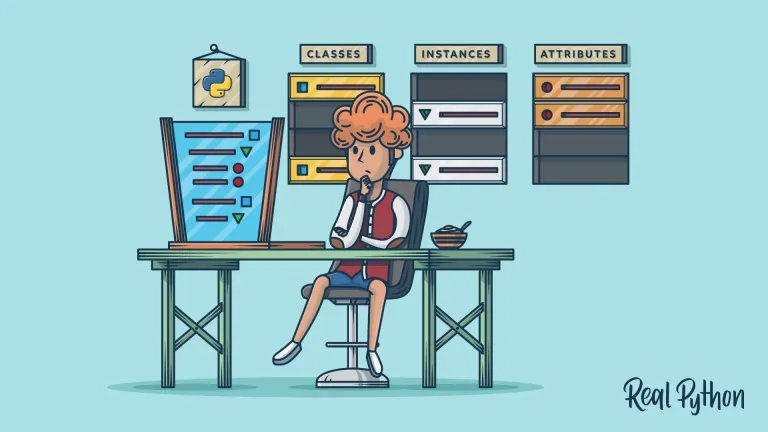
Shoutout to Real Python
In this article you'll find:
- Introduction
- What is polymorphism?
- What does class polymorphism look like in code?
- Polymorphism on inherited classes
- A little example:
Another of the key concepts that we must take into account in object-oriented programming is that of Polymorphism.
You see, without knowing, you may have been doing polymorphism all this time. When you create different functions that have operators with the same name, when you create methods with the same name in different classes and many other ways.
This is why, I will make you aware of the use of Polymorphism, this so that you master another pillar of object-oriented programming and can use it to make your life as a programmer easier. That being said:
What is polymorphism?
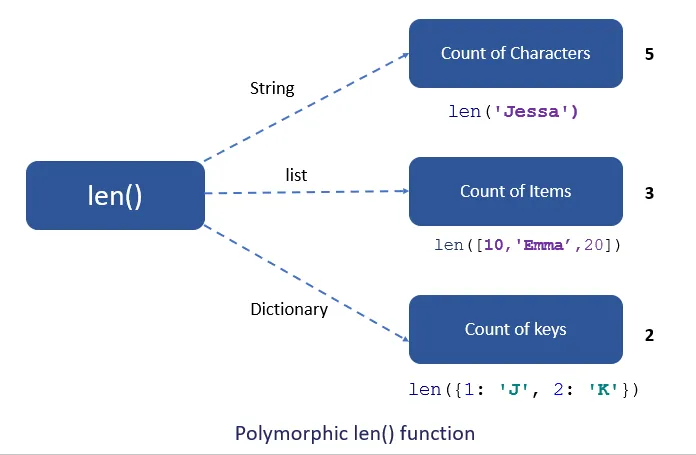
Shoutout to PYnative
To understand polymorphism, we must take the root of this word. Poly has a Latin root for large quantities, as in "much", while morph is a Greek word for form. If we put these two together:
Polymorph = Many forms.
This is precisely what polymorphism does, where we are referring to those functions, methods or operators that can be used in different objects and classes, meaning that they can take many forms.
If we get into functions for different types of objects, we can take a look at the in-built len function, which, depending on the DataType, will act differently to achieve what we want: The number of elements.
If we use len() for a string value:
string1 = 'Hello'
print(len(string1))
>>> 5
Now, if we use it with a list:
list1 = [1,2,3,3,4,5]
print(len(string1))
>>> 5
One tells us the number of characters in a string and the other the number of elements in a list. Although it acts differently depending on the type of value, it is still called len.
For classes, polymorphism applies to methods, where methods of different classes can have the same name. With this, we will not have problems since each class will act only with its own instances, so there will be no collisions in the definitions.
Now what does class polymorphism look like in code?
What does class polymorphism look like in code?
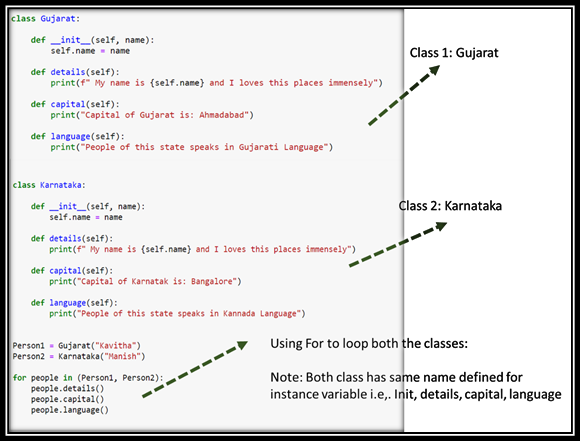
Shoutout to Trepend |Simplifying Business| from Medium
One of the quickest ways to determine polymorphism in classes is to create 3 distinct classes. With the knowledge of this article:
class Class1:
def __init__(self, prop1, prop2):
self.prop1 = prop1
self.prop2 = prop2
def methodX(self):
print(self.prop1 + self.prop2)
class Class2:
def __init__(self, prop1, prop2):
self.prop1 = prop1
self.prop2 = prop2
def methodX(self):
print(self.prop1 - self.prop2)
class Class3:
def __init__(self, prop1, prop2):
self.prop1 = prop1
self.prop2 = prop2
def methodX(self):
print(self.prop1 * self.prop2)
And if we create objects for each class, executing the method, we will have:
obj1 = Class1(2,4)
obj2 = Class2(5,8)
obj3 = Class3(2,6)
obj1.methodX()
obj2.methodX()
obj3.methodX()
>>>
6
-3
12
Thus, we can easily see that it is only necessary to place the same method name in different classes, and according to the class, the method defined within it will be executed.
But what if a child class tries to create a function with the same name as its parent class?
Polymorphism on inherited classes
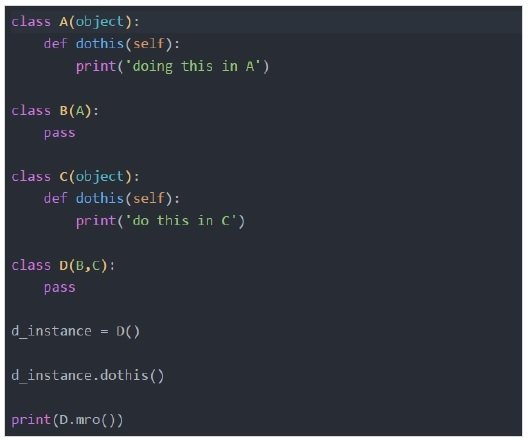
Shoutout to TutorialsPoint
In the previous post about class inheritance, we explained that if we create a class and make it inherit the attributes and methods of another class, we will have a child class of a parent class (Our original class).
However, what if we want to create a method in the child class that has the same name as a method in the parent class? Take the following code as an example:
class FootballTeam():
def __init__(self, team, players):
self.team = team
self.players = players
def Announce(self):
print('The soccer team is: ', self.team)
class TeamNFL(FootballTeam):
def Announce(self):
print('The American Football team is: ', self.team)
If we create an object that is an instance of the TeamNFL class, we will have the Announce function of the FootballTeam class overwritten and the Announce we defined in TeamNFL will be executed instead.
ManUtd = FootballTeam('Manchester United FC', 11)
Raptors = TeamNFL('Raptors', 11)
ManUtd.Announce()
Raptors.Announce()
>>>
The soccer team is: Manchester United FC
The American Football team is: Raptors
A little example:
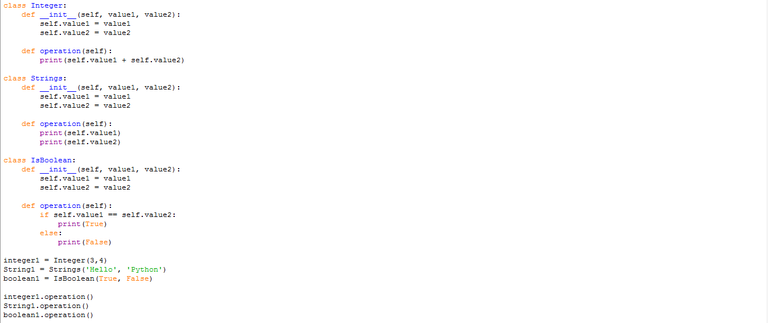
To carry out this example we will rely on the len() function, which according to the DataType performs a different thing. Here, we will do the same, only by means of different classes.
In this case, we want that if its values are of type integer, the object is created as a member of the integer class and that when using our function, the values are added.
If the attributes are of type string, then it will be a member of the Strings class, where when executing the same function, both values will be shown.
Finally if they are boolean, then we want them to be part of the IsBoolean class, where we will execute the function and according to if the value of both booleans is the same, we will return True, and if it is the opposite, False.
Thus, defining each class:
class Integer:
def __init__(self, value1, value2):
self.value1 = value1
self.value2 = value2
def operation(self):
print(self.value1 + self.value2)
class Strings:
def __init__(self, value1, value2):
self.value1 = value1
self.value2 = value2
def operation(self):
print(self.value1)
print(self.value2)
class IsBoolean:
def __init__(self, value1, value2):
self.value1 = value1
self.value2 = value2
def operation(self):
if self.value1 == self.value2:
print(True)
else:
print(False)
Now, if we create an object for each class and execute the function:
integer1 = Integer(3,4)
String1 = Strings('Hello', 'Python')
boolean1 = IsBoolean(True, False)
integer1.operation()
String1.operation()
boolean1.operation()
>>>
7
Hello
Python
False
Polymorphism shows us that there is nothing difficult in object-oriented programming, where if we understand the most important concepts, we will master the rest without any problem.
After this, you will be able to understand that despite having the same name, if we use methods with the same name, they will still perform different functions according to what we assign. Thus, your path to Python mastery becomes shorter.
class newClass(Part2)
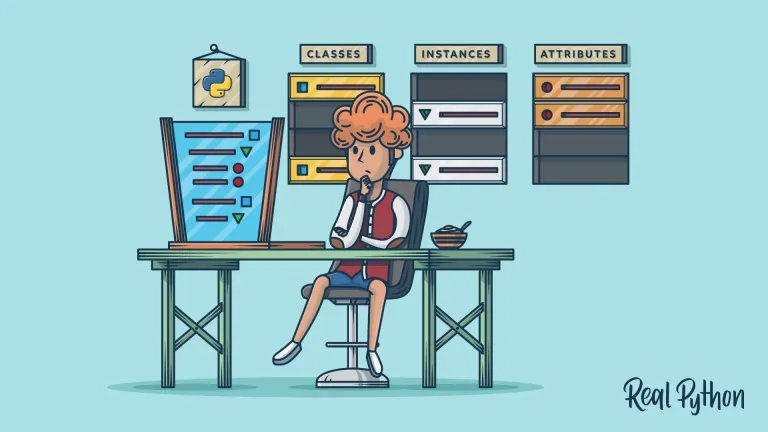
Shoutout to Real Python
En este artículo encontrarás:
- Introducción
- ¿Qué es el polimorfismo?
- ¿Cómo luce el polimorfismo en código?
- Polimorfismo en clases heredadas
- Un pequeño ejemplo
Otro de los conceptos clave que debemos tener en cuenta en la programación orientada a objetos es el de Polimorfismo.
Verás, sin saberlo, puede que hayas estado haciendo polimorfismo todo este tiempo. Cuando creas diferentes funciones que tienen operadores con el mismo nombre, cuando creas métodos con el mismo nombre en diferentes clases y de muchas otras maneras.
Es por esto, que te haré consciente del uso del Polimorfismo, esto para que domines otro pilar de la programación orientada a objetos y puedas utilizarlo para hacer más fácil tu vida como programador. Dicho esto:
¿Qué es el polimorfismo?
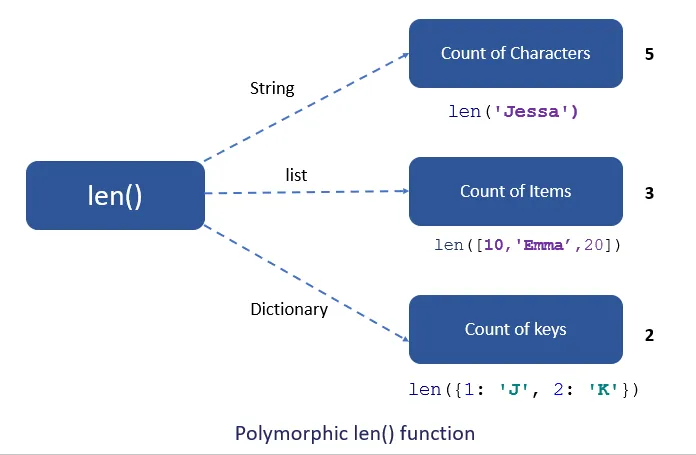
Shoutout to PYnative
Para entender el polimorfismo, debemos partir de la raíz de esta palabra. Poly tiene una raíz latina que significa grandes cantidades, como en "mucho", mientras que morph es una palabra griega que significa forma. Si juntamos estas dos palabras
Polimorfo = Muchas formas.
Esto es precisamente lo que hace el polimorfismo, donde nos referimos a aquellas funciones, métodos u operadores que pueden ser utilizados en diferentes objetos y clases, lo que significa que pueden tomar muchas formas.
Si nos metemos en funciones para diferentes tipos de objetos, podemos echar un vistazo a la función len incorporada, que dependiendo del DataType actuará de forma diferente para conseguir lo que queremos: El número de elementos.
Si usamos len() para un valor de cadena:
string1 = 'Hello'
print(len(string1))
>>> 5
Ahora, si lo usamos con una lista:
list1 = [1,2,3,3,4,5]
print(len(string1))
>>> 5
Uno nos indica el número de caracteres de una cadena y el otro el número de elementos de una lista. Aunque actúa de forma diferente según el tipo de valor, sigue llamándose len.
En el caso de las clases, el polimorfismo se aplica a los métodos, donde los métodos de diferentes clases pueden tener el mismo nombre. Con esto no tendremos problemas ya que cada clase actuará sólo con sus propias instancias, por lo que no habrá colisiones en las definiciones.
Ahora, ¿Cómo luce el polimorfismo de clases en código?
¿Cómo luce el polimorfismo en código?
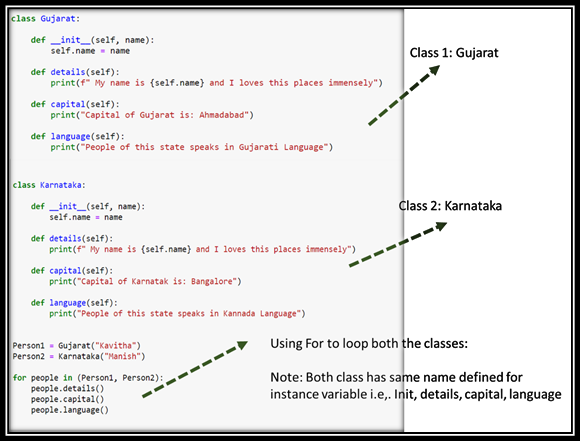
Shoutout to Trepend |Simplifying Business| from Medium
Una de las formas más rápidas de determinar el polimorfismo en las clases es crear 3 clases distintas. Con el conocimiento de este artículo:
class Class1:
def __init__(self, prop1, prop2):
self.prop1 = prop1
self.prop2 = prop2
def methodX(self):
print(self.prop1 + self.prop2)
class Class2:
def __init__(self, prop1, prop2):
self.prop1 = prop1
self.prop2 = prop2
def methodX(self):
print(self.prop1 - self.prop2)
class Class3:
def __init__(self, prop1, prop2):
self.prop1 = prop1
self.prop2 = prop2
def methodX(self):
print(self.prop1 * self.prop2)
Y si creamos objetos para cada clase, ejecutando el método, tendremos:
obj1 = Class1(2,4)
obj2 = Class2(5,8)
obj3 = Class3(2,6)
obj1.methodX()
obj2.methodX()
obj3.methodX()
>>>
6
-3
12
Así, podemos ver fácilmente que sólo es necesario colocar el mismo nombre de método en diferentes clases, y según la clase, se ejecutará el método definido en ella.
Pero, ¿qué ocurre si una clase hija intenta crear una función con el mismo nombre que su clase padre?
Polimorfismo en clases heredadas
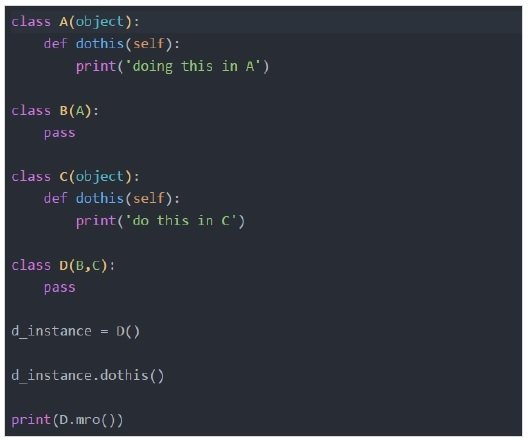
Shoutout to TutorialsPoint
En el post anterior sobre herencia de clases, explicamos que si creamos una clase y hacemos que herede los atributos y métodos de otra clase, tendremos una clase hija de una clase padre (Nuestra clase original).
Sin embargo, ¿qué pasa si queremos crear un método en la clase hija que tenga el mismo nombre que un método de la clase padre? Tomemos el siguiente código como ejemplo:
class FootballTeam():
def __init__(self, team, players):
self.team = team
self.players = players
def Announce(self):
print('The soccer team is: ', self.team)
class TeamNFL(FootballTeam):
def Announce(self):
print('The American Football team is: ', self.team)
Si creamos un objeto que sea una instancia de la clase TeamNFL, se sobrescribirá la función Announce de la clase FootballTeam y se ejecutará en su lugar la Announce que definimos en TeamNFL
ManUtd = FootballTeam('Manchester United FC', 11)
Raptors = TeamNFL('Raptors', 11)
ManUtd.Announce()
Raptors.Announce()
>>>
The soccer team is: Manchester United FC
The American Football team is: Raptors
Un pequeño ejemplo
Para llevar a cabo este ejemplo nos basaremos en la función len(), que de acuerdo al DataType lleva a cabo una cosa distinta. Aquí, haremos lo mismo, solo que por medio de distintas clases.
En este caso, queremos que si sus valores son de tipo integer, el objeto se cree como miembro de la clase integer y que al utilizar nuestra función, se sumen los valores.
Si los atributos son de tipo string, entonces será miembro de la clase Strings, donde al ejecutar la misma función, se mostrarán ambos valores.
Por último si son booleanos, entonces queremos que formen parte de la clase IsBoolean, donde ejecutaremos la función y según si el valor de ambos booleanos es el mismo, devolveremos True, y si es al contrario, False.
Así, definiendo cada clase:
class Integer:
def __init__(self, value1, value2):
self.value1 = value1
self.value2 = value2
def operation(self):
print(self.value1 + self.value2)
class Strings:
def __init__(self, value1, value2):
self.value1 = value1
self.value2 = value2
def operation(self):
print(self.value1)
print(self.value2)
class IsBoolean:
def __init__(self, value1, value2):
self.value1 = value1
self.value2 = value2
def operation(self):
if self.value1 == self.value2:
print(True)
else:
print(False)
Ahora, si creamos un objeto para cada clase y ejecutamos la función:
integer1 = Integer(3,4)
String1 = Strings('Hello', 'Python')
boolean1 = IsBoolean(True, False)
integer1.operation()
String1.operation()
boolean1.operation()
>>>
7
Hello
Python
False
El polimorfismo nos demuestra que no hay nada difícil en la programación orientada a objetos, donde si entendemos los conceptos más importantes, dominaremos el resto sin ningún problema.
Después de esto, serás capaz de entender que a pesar de tener el mismo nombre, si usamos métodos con el mismo nombre, estos realizarán diferentes funciones de acuerdo a lo que les asignemos. Así, tu camino hacia el dominio de Python se hace más corto.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.