Android App Development | Beginner Course | Lecture#15 | Hive Learners
๐๐ป๐ฎ๐ฎ๐ฝ๐ฒ๐ท๐ฐ๐ผ
Hi everyone, Welcome to the 15th Lecture on Android App Development. Today we will arrange our calculator button and also add a decimal point button. It is so important to implement in the calculator. I hope you will learn something new in this lecture. Stay tuned
GitHub Link
Use this GitHub project to clone into your directory. It will always get updated in the next lecture. So that you will never miss the latest code. Happy Coding!.
What Should I Learn
- How to arrange buttons in horizontal
- How to code the decimal point button
- How to set simple if condition
Assignment
- Arrange your layout buttons
- Write code for your decimal point button and test it.
Procedure
First, we need to align the 3 buttons in the horizontal layout. We create a Linearlayout with horizontal orientation and also set the gravity to center. Then we add a three-button in the linear layout tag and then repeat these steps for other buttons.
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
Here I align all the buttons. activity_main.xml
complete code
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/screen1_tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="24sp" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<Button
android:id="@+id/num1_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="1" />
<Button
android:id="@+id/num2_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="2" />
<Button
android:id="@+id/num3_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="3" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<Button
android:id="@+id/num4_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="4" />
<Button
android:id="@+id/num5_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="5" />
<Button
android:id="@+id/num6_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="6" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<Button
android:id="@+id/num7_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="7" />
<Button
android:id="@+id/num8_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="8" />
<Button
android:id="@+id/num9_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="9" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<Button
android:id="@+id/num0_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="0" />
</LinearLayout>
</LinearLayout>
Now add a new button decimal_btn. This button will add decimal points when a user clicks on it. There will be only one decimal point in a number we will tackle it later in coding.
<Button
android:id="@+id/decimal_btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="." />
Now we need to go to the MainActivity.java
file and declare, initialize and see on click for the decimal_btn.
Now we need to create a logic that will prevent the duplication of the decimal point on button click. We will use the if condition to check if the decimal point is already in the number or not. I use a letter ! Exclamation mark. IT indicates not in JAVA. It negates the condition written against it,
if (!screen1_tv.getText().toString().contains(".")) {
}
This condition checks if screen1_tv does not contains a decimal point then write the decimal like this.
if (!screen1_tv.getText().toString().contains(".")) {
screen1_tv.setText(screen1_tv.getText().toString().concat(decimal_btn.getText().toString()));
}
Now, let's run the app and check if this condition prevents duplication or not.

Thank You
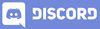
Great lesson!
!1UP
You have received a 1UP from @luizeba!
@stem-curator
And they will bring !PIZZA ๐, !PGM ๐ฎ and !LOLZ ๐คฃ
Learn more about our delegation service to earn daily rewards. Join the Cartel on Discord.