Smart Contract for Arbitrage Opportunities on DEXs
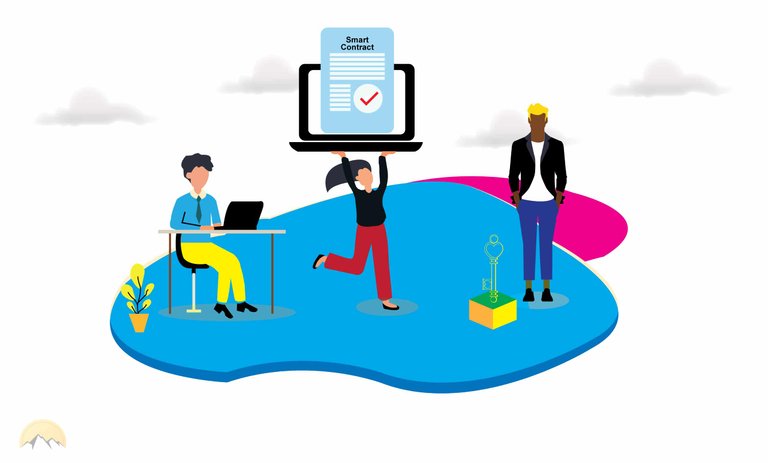
Below is a simple Solidity smart contract that checks for arbitrage opportunities on decentralized exchanges (DEXs) and executes the arbitrage if found. I'll also explain each part of the code line by line.
Smart Contract Code
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@uniswap/v2-periphery/contracts/interfaces/IUniswapV2Router02.sol";
import "@uniswap/v2-core/contracts/interfaces/IUniswapV2Pair.sol";
import "@uniswap/v2-core/contracts/interfaces/IERC20.sol";
contract Arbitrage {
address public owner;
IUniswapV2Router02 public uniswapRouter;
IUniswapV2Router02 public sushiswapRouter;
constructor(address _uniswapRouter, address _sushiswapRouter) {
owner = msg.sender;
uniswapRouter = IUniswapV2Router02(_uniswapRouter);
sushiswapRouter = IUniswapV2Router02(_sushiswapRouter);
}
modifier onlyOwner() {
require(msg.sender == owner, "Not the contract owner");
_;
}
function executeArbitrage(
address token0,
address token1,
uint amount
) external onlyOwner {
uint startBalance = IERC20(token0).balanceOf(address(this));
// Buy token1 on Uniswap
IERC20(token0).approve(address(uniswapRouter), amount);
address[] memory path = new address[](2);
path[0] = token0;
path[1] = token1;
uniswapRouter.swapExactTokensForTokens(
amount,
1,
path,
address(this),
block.timestamp
);
uint token1Amount = IERC20(token1).balanceOf(address(this));
// Sell token1 on Sushiswap
IERC20(token1).approve(address(sushiswapRouter), token1Amount);
path[0] = token1;
path[1] = token0;
sushiswapRouter.swapExactTokensForTokens(
token1Amount,
1,
path,
address(this),
block.timestamp
);
uint endBalance = IERC20(token0).balanceOf(address(this));
require(endBalance > startBalance, "Arbitrage not profitable");
}
}
Explanation
License and Pragma:
// SPDX-License-Identifier: MIT pragma solidity ^0.8.0;
- Specifies the license type and the Solidity compiler version.
Imports:
import "@uniswap/v2-periphery/contracts/interfaces/IUniswapV2Router02.sol"; import "@uniswap/v2-core/contracts/interfaces/IUniswapV2Pair.sol"; import "@uniswap/v2-core/contracts/interfaces/IERC20.sol";
- Imports necessary interfaces for interacting with Uniswap and ERC20 tokens.
Contract Definition:
contract Arbitrage { address public owner; IUniswapV2Router02 public uniswapRouter; IUniswapV2Router02 public sushiswapRouter;
- Defines the
Arbitrage
contract with state variables for the owner and the routers for Uniswap and Sushiswap.
- Defines the
Constructor:
constructor(address _uniswapRouter, address _sushiswapRouter) { owner = msg.sender; uniswapRouter = IUniswapV2Router02(_uniswapRouter); sushiswapRouter = IUniswapV2Router02(_sushiswapRouter); }
- Initializes the contract with the addresses of the Uniswap and Sushiswap routers and sets the contract owner.
Modifier:
modifier onlyOwner() { require(msg.sender == owner, "Not the contract owner"); _; }
- Restricts access to certain functions to only the contract owner.
Arbitrage Execution Function:
function executeArbitrage( address token0, address token1, uint amount ) external onlyOwner { uint startBalance = IERC20(token0).balanceOf(address(this));
- Defines the
executeArbitrage
function which takes two token addresses and an amount to trade. It checks the initial balance oftoken0
.
- Defines the
Buying on Uniswap:
IERC20(token0).approve(address(uniswapRouter), amount); address[] memory path = new address[](2); path[0] = token0; path[1] = token1; uniswapRouter.swapExactTokensForTokens( amount, 1, path, address(this), block.timestamp );
- Approves the Uniswap router to spend
token0
, sets up the swap path, and executes the swap fromtoken0
totoken1
.
- Approves the Uniswap router to spend
Selling on Sushiswap:
uint token1Amount = IERC20(token1).balanceOf(address(this)); IERC20(token1).approve(address(sushiswapRouter), token1Amount); path[0] = token1; path[1] = token0; sushiswapRouter.swapExactTokensForTokens( token1Amount, 1, path, address(this), block.timestamp );
- Approves the Sushiswap router to spend
token1
, sets up the swap path, and executes the swap fromtoken1
back totoken0
.
- Approves the Sushiswap router to spend
Profit Check:
uint endBalance = IERC20(token0).balanceOf(address(this)); require(endBalance > startBalance, "Arbitrage not profitable");
- Checks the final balance of
token0
to ensure the arbitrage was profitable.
- Checks the final balance of
0
0
0.000
Hello.
We would appreciate it if you could avoid copying and pasting or cross-posting content from external sources (full or partial texts, video links, art, etc.).
Thank you.
If you believe this comment is in error, please contact us in #appeals in Discord.
Congratulations @alfredo24! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 50 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Check out our last posts: