Unlocking the Power of Swift: An Introduction to Programming Structure, Constants, and Variables
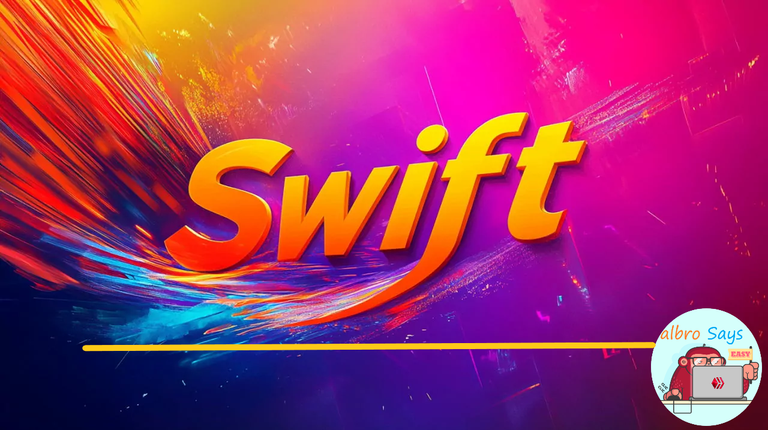
Swift is a general-purpose programming language designed specifically for building Apple products. All this while the first priority is given to the speed, stability and security of the programs. The Swift programming language is behind the success of many iPhone applications. Another advantage of Swift is its simple learning process. This language works on platforms like iOS, OS X, tvOS, and watchOS. Swift has the best parts of C and Objective-C languages. In addition to using all the advantages of the C language, this programming language has also removed its limitations.
What is Swift programming language?
Swift programming language is a multi-purpose, object-oriented, functional and block-structured language. Swift is the result of the latest research on programming languages and was developed by Apple. This language focuses on security and follows the latest software design patterns. The Swift language is designed to design and build software for Apple operating systems such as iOS, OS X, tvOS, and watchOS.
The process of learning this language is simple. Simple implementation, high security, high speed and effectiveness are important features of this programming language. The software development process by Swift has also become more attractive since it started supporting other platforms and software.
Swift language works with Cocoa and Cocoa Touch frameworks. It can also use Objective-C code for all Apple products. This language is designed to handle possible mistakes during coding better than Objective-C. As a result, it is more secure than the Objective-C programming language. In addition to the above, the readability of this programming language is also high. Swift uses the LLVM compiler framework, which includes Xcode version 6 and higher.
Since the Swift programming language is open source to the public, it can also be ported to the Internet. Some web frameworks such as Kitura, Perfect and Vapor have already been developed by IBM. On the other hand, Apple is responsible for the development of server-side APIs, and Swift language programmers are one of the most important contributors to this project.
The software company RemObjects Software has provided another free version of the Swift language that works with platforms such as Cocoa and the .NET framework from Microsoft, Java and Android. This version is fully compatible with all these platforms.
In addition to the mentioned case, with the help of the combination of LLVM and Macintosh Programmer's Workshop tools, it is possible to run a small part of the Swift language on Mac OS 9.
Basic coding tips with Swift programming language
Unlike many object-oriented programming languages that are based on older procedural languages - for example, C++ and Objective-C were developed with C - Swift was designed from the ground up as a new, modern, object-oriented language that makes it faster and easier. This language helps programmers write clearer code that is less error-prone than most languages.
Although the Swift language is not based on an older language, according to the head of its architecture team, Chris Lattner, Swift has been inspired by many programming languages such as Ruby, Python, #C, CLU, and many others.
Like most modern programming languages, Swift derives most of its basic syntax from the C programming language. If you already have experience working with other languages derived from C, most aspects of the Swift language syntax will be familiar to you.
- Programs are made up of statements that are executed sequentially.
-
In each line, we can code more than one expression. provided that they are separated from each other with a
;
. - Units of work in Swift are modularized and organized in different ways using functions.
- Functions defined in Swift accept one or more different parameters and return a value in the output.
- Single or multiple comments follow the syntax used in C++ and Java.
- Swift has the concept of named variables that are mutable and named constants that are immutable.
- Like C++ and C#, Swift supports both the concepts of structs and classes.
Apart from the above, there are other syntax tips in C-inspired languages that you may have come across while working with these languages. In some of these points, the Swift language includes differences and improvements over those languages. I have listed the most important points of dispute in the list below.
-
It is not necessary to use a
;
at the end of the sentences. Except for the cases where several command statements are written in one line of code and a space should be placed between them. -
The Swift programming language does not need to use the
main()
method to indicate the starting point of the program when the application is loaded by the operating system. Swift programs start from the first line of code in the code source file. Most interpreted programming languages start their work like this. - In Swift functions, the type of the return value is written on the right side of the function definition instead of on the left side.
- The syntax for defining function parameters in Swift language is inspired by Objective-C language. This is completely different from languages like Java, C# and C++. As a result, developers of these languages may be a little confused at the beginning of working with Swift.
- The difference between a structure and a class in Swift is the same as in C#. The syntax of both languages is the same except that the members of the structures are public by default.
Swift program structure
In this section, I want to show the basic structure of Swift language programs. For this purpose, I have implemented a simple program to display the string Hello, World
in the console.
let message = "Hello, World"
print(message)
After executing the above code, the output is created as below and displayed in the console.
Hello, World
So far, we have written the first executable program using the Swift programming language.
It is safe to say that most programming languages can store different values in memory by assigning names to specific locations for each value in memory. These names are chosen by the programmers. Variables help programmers to perform various operations on data. Therefore, the value inside the variables can be changed during the execution time of the program.
To define a variable in Swift, we use the syntax shown below.
var <variable name>: <type> = <value>
For example, based on the above syntax, the variable pi
should be defined as follows.
var pi: Double = 3.14159
In the above line of code, we instruct the compiler to create a variable named pi
. This variable stores data of type Double
. Then assign an initial value of 3.14159
to it.
We may want to store a named value so that it is immutable during program execution. In this section, we will see how to make sure that after defining and initializing the variables, they will not be changed randomly by another part of the code. The solution is to define "Constant".
In the Hello, World
program I wrote above, I defined the message
variable using the let
keyword instead of var
. Therefore, message
is a type of constant variables.
Since message
is defined as a constant, if we add a line of code to the end of the program that wants to change the constant value of message
, we will encounter the message "Compile-Time Error
". Because changing the constant defined with let
is not allowed. I have displayed the text of this compile time error message below.
[Out:]error: cannot assign to value: ‘message’ is a ‘let’ constant
In general, whenever we define a named variable that will never change during program execution, we should use the let
keyword to make it static. When a variable has been created and not changed during program execution, the Swift programming language compiler announces this recommendation—using the let
keyword instead of var
—by displaying a Compile-Time Warning.
The only difference between variables and constants in the Swift language is that after defining and initializing the constants, it is no longer possible to change their value. Apart from this rule, variables and constants are used in the same way in Swift language.
In the Hello, World
example, I created a constant called message
without specifying its data type. In this code, I have used one of the features of the Swift compiler called "Type Inference".
When we assign a certain value to a variable or constant at the same time, the Swift programming language compiler analyzes the data on the right side of the assignment sign - the same as equals. Detects the corresponding data type of that value and assigns it to the variable or constant defined on the left. For example, in the following variable definition, the compiler defines a variable named name
with the type String
without directly referring to the type.
var name = "Mostafa"
Swift, as a type-safe language, whenever a specific type is assigned to a variable or constant by the compiler, it preserves that type until the lifetime of the variable or constant in question expires. Attempting to assign a non-string value to the name
variable defined above will result in a compile-time error.
name = 3.14159
After executing the above code, the following output will be displayed in the Swift console.
[Out:]error: “cannot assign value of type ‘Double’ to type ‘String’
Although Swift is a Type-Safe programming language. The type of the variable value is explicitly specified and does not change during runtime.By using the Any data type in Swift, we can write program code to behave like dynamically typed languages.
Rules for naming variables in Swift
Like most C-inspired programming languages, variables and constants in Swift follow the same naming conventions.
- No names should start with numbers.
- After the first character, we are allowed to use numbers.
-
Names can be started with an underscore (
_
). Using this character in the middle of names is also allowed. - All names are case sensitive.
- Reserved keywords in Swift can be used as variable names as long as they are enclosed in backticks.
When choosing names for variables or constants in Swift, the generally accepted rule is to use the camelCase technique. Of course, the first character of the name must be written with a small English letter. By following generally accepted naming conventions, others can read and understand the code more easily.
In Swift language, unlike other programming languages, we are not limited to using English alphabet characters for naming variables and constants. Therefore, we can use other characters defined in Unicode to name the variables.
Unicode includes character encoding, display and processing of text in most languages of the world. Unicode has more than 65,535 characters - 16 bits. Encodings can describe more characters; But the first 65,535 bits cover most of the existing languages.
Note: Just because we can use all Unicode characters and reserved keywords in Swift with quotes as names for variables and constants doesn't mean we have to. Always consider other developers who may want to read and make changes to your code in the future. The priority of variable naming is always to increase the readability, understandability and maintainability of the codes.
In Swift language, we can print the value of a variable or a constant to the console using the print()
function. Below, I have implemented an example about the method of creating a constant called name
and a variable called address
.
let name = "albro Blog" // constant
var address = "Hive Blockchain" // variable
print("\(name) lives at \(address)") // Print statement
After executing the above code, the following output will be displayed in the console.
[Out:]albro Blog lives at Hive Blockchain
Both name
and address
are stored as string text in programming. By enclosing the variable or constant name in a pair of open and closed parentheses and adding the backslash character (\
) as a prefix to them, we can print the values stored inside them in the expression related to the print()
function. This work is called "String Interpolation".
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.