JS Dom: Change HTML & CSS By albro
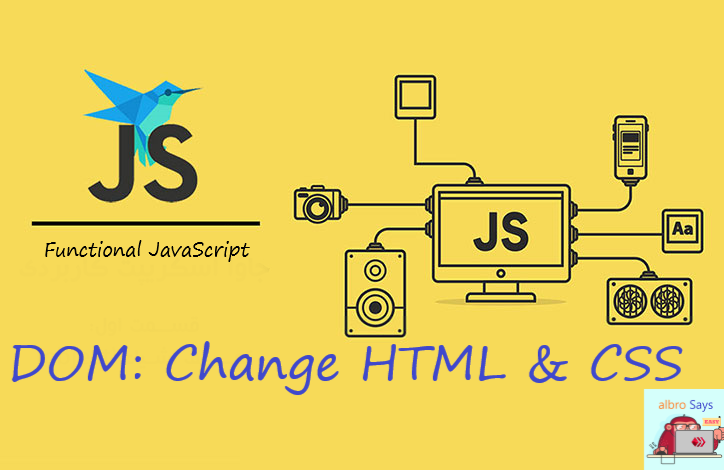
We can change the HTML content of a page using JavaScript and DOM. To create something that doesn't exist in HTML, the document.write()
statement is usually used. This statement directly inserts what we want into the HTML document. For example:
<!DOCTYPE html>
<html>
<body>
<script>
document.write(Date());
</script>
</body>
</html>
Warning
: Never use document.write()
after the page is fully loaded! If the page is loaded, document.write()
overwrites the entire page; This means that all your content will be deleted and only the new content you gave to document.write()
will be displayed!
Although this statement is powerful, it's rarely used because it sometimes causes problems. Most of the time our intention is to change the existing content. The easiest way to do this is to use innerHTML
.
To change the content of HTML elements by innerHTML
, you must follow the following structure:
document.getElementById(id).innerHTML = new HTML
In the following example I want to change the <p>
element:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript can Change HTML</h2>
<p id="p1">Hello World!</p>
<script>
document.getElementById("p1").innerHTML = "This paragraph has been modified by JavaScript codes!";
</script>
<p>The original text of this paragraph has been modified by JavaScript codes!!!!</p>
</body>
</html>
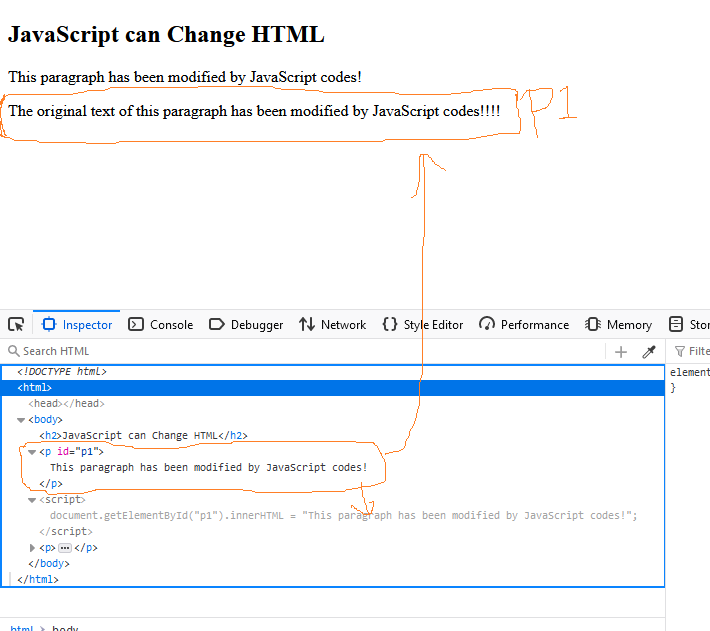
If you pay attention to the code of the example above, you will notice that the content of the paragraph was originally Hello World!
, but nothing like that is displayed for us anymore. The reason is to use JavaScript code to change this content. I used id="p1"
to get the element in DOM. Then I changed its content using innerHTML
.
Note
: Many times when working with the DOM (like the example above), the HTML source code does not change and the change is visible in the output, so if you encounter such cases, don't assume that your program has a problem.
What if you want to change the value of an attribute? There is a simple structure for this as well:
document.getElementById(id).attribute = new value
For example, in the following code I want to change the src
for <img>
:
<!DOCTYPE html>
<html>
<body>
<img id="image" src="https://ecency.com/static/media/logo-circle.2df6f251.svg" width="160" height="120">
<script>
document.getElementById("image").src = "https://peakd.com/static/img/peakd_logo_white.0091ccd4.svg";
</script>
<p>The original image that you see in the source code was the ecency logo, but in the new image, the Peakd logo is placed.</p>
</body>
</html>
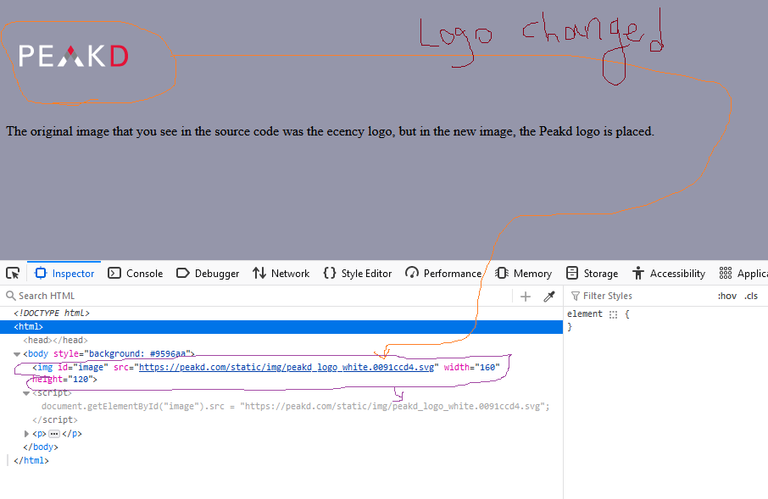
First, I got image
, which was the id of our element, through the getElementById
statement. Then I have changed the value of the attribute called src
to another image.
Modifying CSS content
When we say that CSS content is modified by JavaScript, we mean a CSS type:
- CSS that is written inline.
Therefore, JavaScript can modify the CSS codes that are inside the HTML document.
To create new styles, we can use this structure:
document.getElementById(id).style.property = new style
For example, in the following code I want to style the <p>
element:
<!DOCTYPE html>
<html>
<body>
<p id="p1">Hello World!</p>
<p id="p2">Hello World!</p>
<script>
document.getElementById("p2").style.color = "blue";
document.getElementById("p2").style.fontFamily = "Arial";
document.getElementById("p2").style.fontSize = "larger";
</script>
<p>The paragraph above was changed by a script.</p>
</body>
</html>
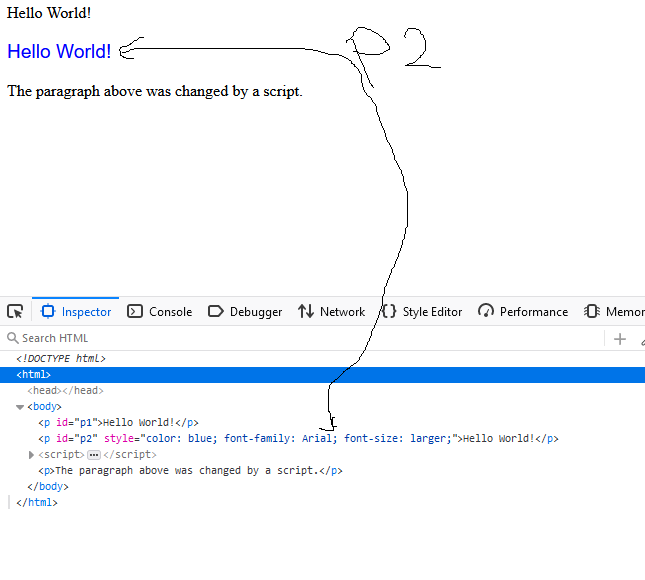
JavaScript can define events. Events are events that are executed when a certain event occurs. For example, clicking on a certain part of the page, loading the page, changing a file, moving the user's mouse over a certain element, etc. All of these are an event. Now we can use events in the context of CSS:
<!DOCTYPE html>
<html>
<body>
<h1 id="id1">My Heading 1</h1>
<button type="button"
onclick="document.getElementById('id1').style.color = 'red'">
Click Me!</button>
</body>
</html>
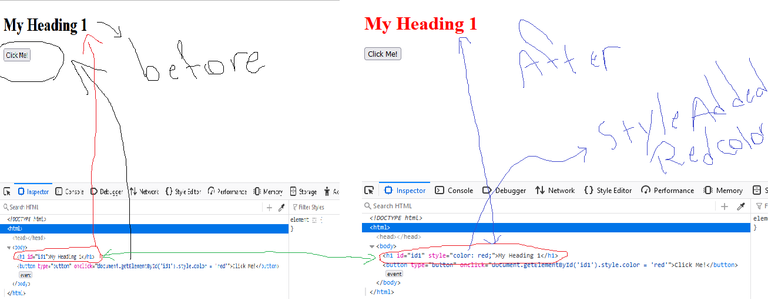
In the code above, I received an element with the ID id1
(getElementById
statement) and then I said that if the user clicks on a certain button (onclick
), change the color of our text in h1
! You can go to the above code output and see this change by clicking this button
.
A more advanced example is to show and hide text:
<!DOCTYPE html>
<html>
<body>
<p id="p1">
This is a text.<br />
This is a text.<br />
This is a text.<br />
This is a text.<br />
</p>
<input type="button" value="Hide Text" onclick="document.getElementById('p1').style.visibility='hidden'">
<input type="button" value="Display Text" onclick="document.getElementById('p1').style.visibility='visible'">
</body>
</html>
Working with DOM is very easy and with a little practice you can master it. I hope you enjoyed this post.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
I love your profession darling
Congratulations @albro! You received a personal badge!
You can view your badges on your board and compare yourself to others in the Ranking
Check out our last posts: