JS Dom & Tricks By albro
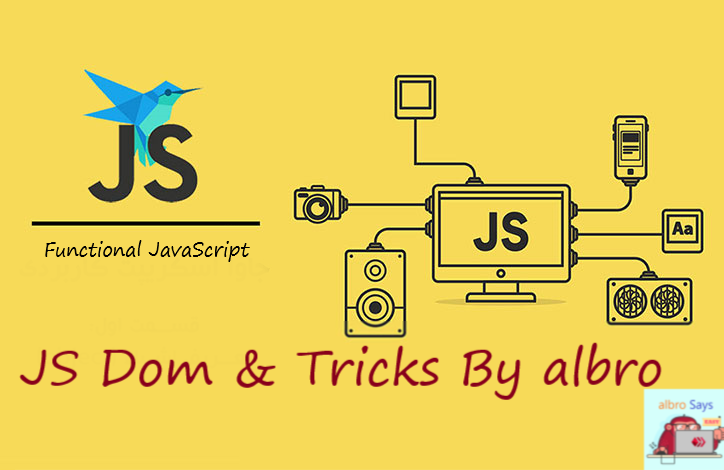
In this post, I'm talking about DOM, the most important and practical topic in JavaScript.
What is DOM?
DOM stands for Document Object Model. Using the DOM you can modify, delete or create HTML document elements!
In fact, DOM is one of the World Wide Web Consortium (W3C) standards that defines a standard for document access:
The W3C Document Object Model (DOM) is a platform and language-neutral interface that allows programs and scripts to dynamically access and update the content, structure, and style of a document.
This W3C standard is divided into three parts:
- Core DOM: Standard model for all types of documents
- XML DOM: Standard model for XML documents
- HTML DOM: The standard model for HTML documents
When your web page loads, the browser creates an object-oriented model of the page. In the case of HTML language, this model is made as a tree of different objects. Do not look at the image below:
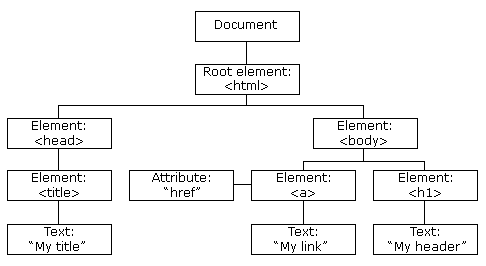
Based on this tree, JavaScript can access a variety of HTML elements:
- JavaScript can modify all HTML elements on a page
- JavaScript can change all attributes of HTML elements
- JavaScript can change all CSS directives on the page
- JavaScript can remove all attributes and various HTML elements
- JavaScript can create new HTML elements on the page
- JavaScript can react to page events and do various things
- JavaScript can create various events on the page
These are functions of DOM and JavaScript!
Working with the DOM
When I talk about DOM properties, I mean values that can be set (such as changing the content of HTML elements), and when I talk about DOM methods, I mean actions that I can perform (such as removing or adding elements).
In the example below, I want to change the content (which is innerHTML
) of a <p>
tag that has "id="demo"
:
<!DOCTYPE html>
<html>
<body>
<h2>My First Page</h2>
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML = "Hello World!";
</script>
</body>
</html>
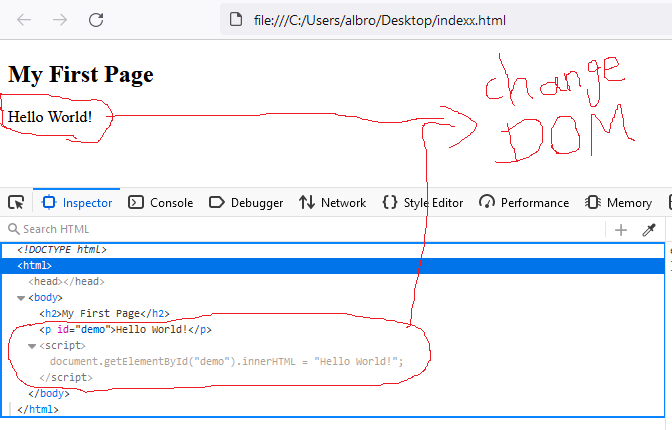
In the example above, getElementById
is a method, but innerHTML
is a property; The getElementById
method, as the name suggests, receives an HTML element and passes it to JavaScript, then innerHTML
displays its content. We can change this content to change the content of the tag as well.
There are many ways to access HTML elements, and accessing via id
is one of the most common ways, as you saw in the example above. You should also know that innerHTML
can access the content of all HTML elements, even <html>
and <body>
document object
The document object represents your web page and is the owner of all other objects in the HTML document. So if you want to access an element in HTML, you have to start with the document
object.
To find HTML elements:
Method | Description |
---|---|
document.getElementById(id) | Find element using id |
document.getElementsByTagName(name) | Find element using tag name |
document.getElementsByClassName(name) | Find element using class name |
To modify HTML elements:
property | Description |
---|---|
element.innerHTML = new html content | Modify the innerHTML (content) of an element |
element.attribute = new value | Changing the attribute value of an element |
element.style.property = new style | Change the style (CSS) of an element |
Method | Description |
---|---|
element.setAttribute(attribute, value) | Changing the attribute value of an element |
To add or remove HTML elements:
Method | Description |
---|---|
document.createElement(element) | Constructing an HTML element |
document.removeChild(element) | Remove an HTML element |
document.appendChild(element) | Add an HTML element |
document.replaceChild(new, old) | Replace an HTML element |
document.write(text) | Write to the output of an HTML element |
To add events:
Method | Description |
---|---|
document.getElementById(id).onclick = function(){code} | Add event handler to onclick event |
Accessing HTML objects
When HTML DOM Level 1 was introduced in 1998, only 11 HTML and ... objects were introduced, which are still valid in HTML5, but many more were added later in HTML DOM Level 3. I have provided some of these items for you in the following tables:
property | Description |
---|---|
document.anchors |
Returns all <a> s that have an attribute named name
|
document.baseURI | Returns base URI of the document |
document.body |
Returns us the <body> element
|
document.cookie | It returns all the cookies in the document |
document.doctype | Returns the doctype |
document.documentElement |
Returns the <html> element
|
document.documentMode | Returns the mode used by the browser |
document.documentURI | Returns the URI of the current document |
document.domain | Returns the domain name of the document server |
document.embeds |
Returns all <embed> elements
|
document.forms |
Returns all <form> elements
|
document.head |
Returns the <head> element
|
document.images |
Returns all <img> elements
|
document.implementation | Returns all DOM implementations |
document.inputEncoding | Returns the encoding (character set) of the document. |
document.lastModified | Returns the date and time when the document was last updated |
document.links |
Returns all <area> and <a> that have href
|
document.readyState | Returns the loading position of the document |
document.referrer | Returns the URI of the linked document (referrer) |
The main use of the JavaScript language (at least in the web context) is to change HTML elements and their styles, and it does not do much by itself (in the frontend); That is, we try to learn different JavaScript statements so that we can use them later in changing the shape of HTML elements. Of course, the JavaScript language is not limited to the frontend and is used in many cases such as the backend.
Access to HTML elements
Using JavaScript, we can access all kinds of HTML tags, but we need to learn how to do this. There are different ways to access these elements, and each way has its own statements and usage; These access ways are:
-
Accessing HTML elements by
id
(likeid="first"
) -
Accessing HTML elements through the tag itself (such as
<p>
) -
Accessing HTML elements by class name (eg
class="first"
) -
Accessing HTML elements through CSS selectors (such as
.first
) - Accessing HTML elements through collections of HTML objects (such as forms)
Access through id
The easiest way to access HTML tags is through id
; For this, you need to use the getElementById()
statement:
<!DOCTYPE html>
<html>
<body>
<h2>Finding HTML Elements by Id</h2>
<p id="intro">Hello World!</p>
<p>This example demonstrates the <b>getElementsById</b> method.</p>
<p id="demo"></p>
<script>
var myElement = document.getElementById("intro");
document.getElementById("demo").innerHTML =
"The text from the intro paragraph is " + myElement.innerHTML;
</script>
</body>
</html>
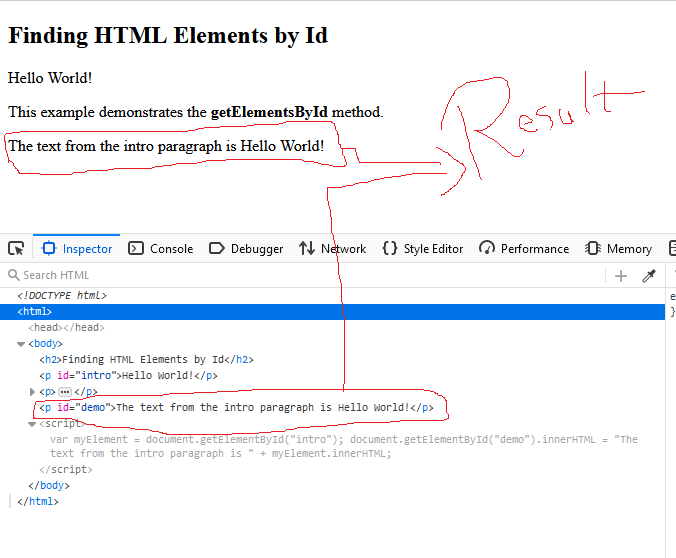
If the HTML tag is found by our JavaScript statement, it will be returned as an object, but if nothing is found, it will return null
.
Access through the tag itself
One of the other ways to access HTML elements is through the name of the tag itself; For this, you need to use the getElementsByTagName()
statement. In the following example, I want to find all <p>
elements:
<!DOCTYPE html>
<html>
<body>
<h2>Finding HTML Elements by Tag Name</h2>
<p>Hello World!</p>
<p>This example demonstrates the <b>getElementsByTagName</b> method.</p>
<p id="demo"></p>
<script>
var x = document.getElementsByTagName("p");
document.getElementById("demo").innerHTML =
'The text in first paragraph (index 0) is: ' + x[0].innerHTML;
</script>
</body>
</html>
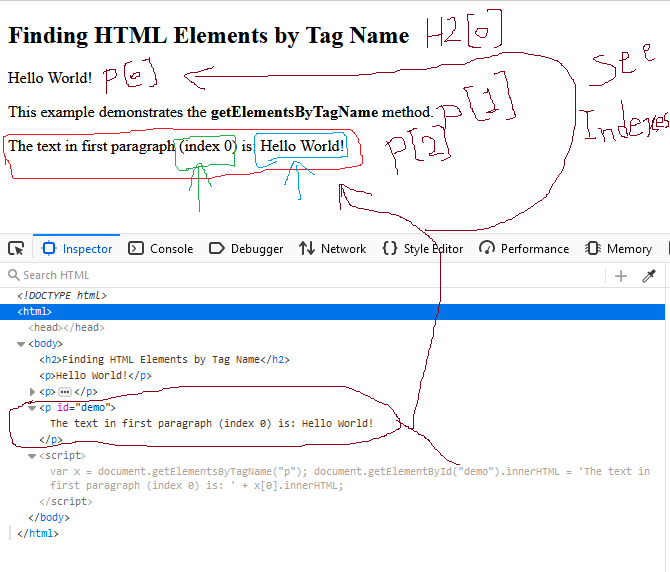
If you pay attention, in this example, all p
tags are returned to us like an array/object, and we deal with them in the same way (using index 0
).
These examples can be made more advanced; The following code first finds elements that have id="main"
and then finds elements that have <p>
tags and are inside main:
<!DOCTYPE html>
<html>
<body>
<h2>Finding HTML Elements by Tag Name</h2>
<div id="main">
<p>The DOM is very useful.</p>
<p>This example demonstrates the <b>getElementsByTagName</b> method.</p>
</div>
<p id="demo"></p>
<script>
var x = document.getElementById("main");
var y = x.getElementsByTagName("p");
document.getElementById("demo").innerHTML =
'The first paragraph (index 0) inside "main" is: ' + y[0].innerHTML;
</script>
</body>
</html>
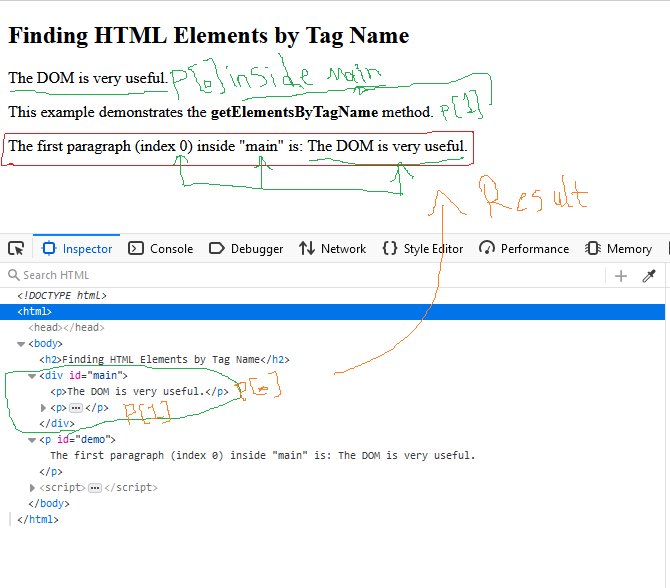
Access by class
If you want to find all the HTML elements that belong to the same class, you should use the getElementsByClassName()
statement. For example, the following code finds all elements that have class="intro"
:
<!DOCTYPE html>
<html>
<body>
<h2>Finding HTML Elements by Class Name</h2>
<p>Hello World!</p>
<p class="intro">The DOM is very useful.</p>
<p class="intro">This example demonstrates the <b>getElementsByClassName</b> method.</p>
<p id="demo"></p>
<script>
var x = document.getElementsByClassName("intro");
document.getElementById("demo").innerHTML =
'The first paragraph (index 0) with class="intro": ' + x[0].innerHTML;
</script>
</body>
</html>
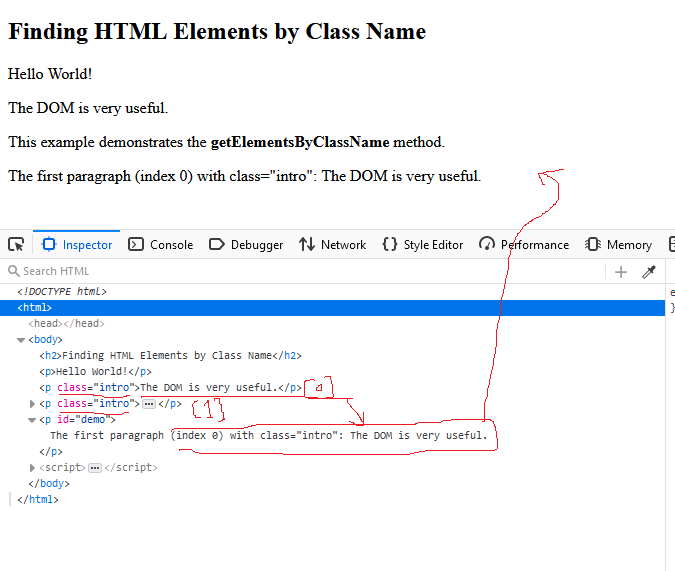
Note: This statement does not work in Internet Explorer 8 and earlier versions.
Access through CSS selectors
If you want to find all HTML elements that match a specific CSS selector, you can use the querySelectorAll()
statement. CSS selectors are things like id
, class
, attributes
, attribute value
, etc.
In the following example, we want to find all <p>
s that contain class="intro"
:
<!DOCTYPE html>
<html>
<body>
<h2>Finding HTML Elements by Query Selector</h2>
<p>Hello World!</p>
<p class="intro">The DOM is very useful.</p>
<p class="intro">This example demonstrates the <b>querySelectorAll</b> method.</p>
<p id="demo"></p>
<script>
var x = document.querySelectorAll("p.intro");
document.getElementById("demo").innerHTML =
'The first paragraph (index 0) with class="intro": ' + x[0].innerHTML;
</script>
</body>
</html>
Access through the HTML objects
The best way to learn this type of access is by example. In the following example, I want to find the form with id="frm1"
among the HTML forms and show its different values:
<!DOCTYPE html>
<html>
<body>
<h2>Finding HTML Elements Using document.forms</h2>
<form id="frm1" action="">
First name: <input type="text" name="fname" value="Donald"><br>
Last name: <input type="text" name="lname" value="Duck"><br><br>
<input type="submit" value="Submit">
</form>
<p>Click "Try it" to display the value of each element in the form.</p>
<button onclick="myFunction()">Try it</button>
<p id="demo"></p>
<script>
function myFunction() {
var x = document.forms["frm1"];
var text = "";
var i;
for (i = 0; i < x.length ;i++) {
text += x.elements[i].value + "<br>";
}
document.getElementById("demo").innerHTML = text;
}
</script>
</body>
</html>
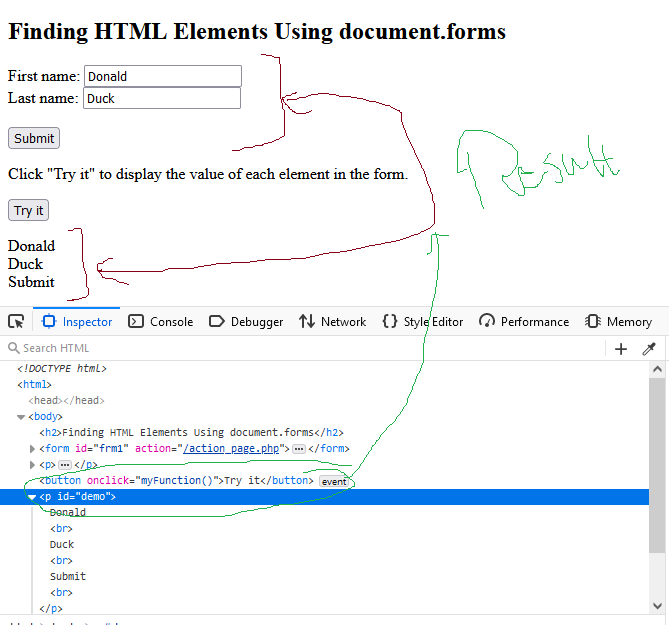
If you go to the output of the above code and then click on the try it
button, you will see that the different values of the form (First name
, Last name
and submit
) will be displayed for you. Now enter your name and family in the form and click on the try it
button again. This time your name and family will be displayed!
https://inleo.io/threads/albro/re-leothreads-2rq3p5grs
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Is there a reason you use var to declare variables? It's generally considered bad practice to do that, it's better you use const and let when declaring variables. Good job by the way
You are absolutely right!😮💨 This is a bad habit!!!!😪
That is a good class, when I started to learn front end programming this knowledge was essential and it isn't easy for those used with back end
Want to Know more about Hivepakistan?
Ping Us On Hive Pakistan Discord server
To support HivePakistan, delegate Hive Power to hivepakistan and earn 90% curation reward :)
Here are some handy links for delegation
A delegation of 500 or more HP makes you earn Hivepakistan supporter badge.
You can use var when it comes to declaring global variables, and yet the extra features they have compared to let and const are nominal and barely useful.
Congrats!
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team