Javascript Function Call And Tricks By albro
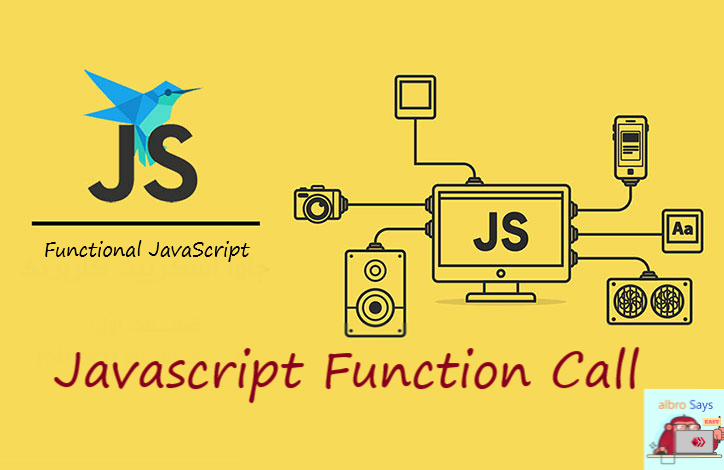
In this post I want to review the types of calls in JavaScript functions.
Call functions
The code inside a JavaScript function is not executed until the function is called. "Calling" is equivalent to call a function and invoke a function, they are not different from each other and you can use any one you like.
The following example is a simple example of calling JavaScript functions:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>The global function (myFunction) returns the product of the arguments (a ,b):</p>
<p id="demo"></p>
<script>
function myFunction(a, b) {
return a * b;
}
document.getElementById("demo").innerHTML = myFunction(10, 2);
</script>
</body>
</html>
Note: As it is clear, the above function does not belong to any specific object, but this is the appearance of the case. In JavaScript, there is always a default global object. In HTML documents, the global object is the HTML page, so the function I wrote above belongs to the HTML page object. But when this HTML document is placed in a browser, the main object is the window
object, so the above code can be written as follows:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>Global functions automatically become window methods. Invoking myFunction() is the same as invoking window.myFunction().</p>
<p id="demo"></p>
<script>
function myFunction(a, b) {
return a * b;
}
document.getElementById("demo").innerHTML = window.myFunction(10, 2);
</script>
</body>
</html>
And we see that myFunction
is exactly the same as window.myFunction
.
Although this method is a popular and simple method of calling and creating functions, it is not the best way, because in some cases global variables, methods or functions can be identical to the names that already exist in the global object, causing creation bugs in your program.
The this
keyword refers to the object that owns the current code. So if we use this keyword in a function, this
returns to the object that owns this function.
If our code is without a specific object, what does the this
keyword indicate? If your function or code is not inside a specific object, the this keyword points to the global object. For example, we can write the following code to specify the main object:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>In HTML the value of <b>this</b>, in a global function, is the window object.</p>
<p id="demo"></p>
<script>
var x = myFunction();
function myFunction() {
return this;
}
document.getElementById("demo").innerHTML = x;
</script>
</body>
</html>
In the output, we can see that [object Window]
is returned to us.
Warning: Using [object Window]
as a variable will cause your program to crash and stop completely. As a general rule, I will tell you never to touch functions, objects and generally build-in JavaScript code.
Call functions as methods
I can define JavaScript functions as methods of different objects. In this case, I have to define the function inside the desired object:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>myObject.fullName() will return John Doe:</p>
<p id="demo"></p>
<script>
var myObject = {
firstName:"Albro",
lastName: "Hive Blog",
fullName: function() {
return this.firstName + " " + this.lastName;
}
}
document.getElementById("demo").innerHTML = myObject.fullName();
</script>
</body>
</html>
In this case, the this
keyword refers to the object that owns the code, myObject
. If I change the above object and replace it with the following code:
var myObject = {
firstName:"Albro",
lastName: "Hive Blog",
fullName: function () {
return this;
}
The output will be [object Object]
, which indicates that the owner of this method is myObject
.
Calling the function through the Function Constructor
In simple words: if a function is called with the new
keyword, it was called through the constructor. It looks like you're creating another function, but since JavaScript functions are objects, you're creating a new object:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>In this example, myFunction is a function constructor:</p>
<p id="demo"></p>
<script>
function myFunction(arg1, arg2) {
this.firstName = arg1;
this.lastName = arg2;
}
var x = new myFunction("Albro","Hive Blog")
document.getElementById("demo").innerHTML = x.firstName;
</script>
</body>
</html>
In this case, the this
keyword in a constructor does not have a value, but the value of this
is the new object that is created when the function is called.
Using the call()
function
One of the things that I should talk about is the call()
method. Using this method, you can have methods that run on other objects.
In JavaScript, all functions are special object methods. In such a way that if a function does not have its own object, it will be part of the JavaScript global object.
Now what the call()
method does for us is that it receives the desired object as a parameter and executes a certain method on it.
What does this feature do for us?
It seems useless by itself, but if you look carefully, you will notice that an object can use a method that belongs to another object through call()
! So you can avoid a lot of overwriting and at the same time save your time. Imagine implementing the methods of an object on any object you want! This function is one of the most useful JavaScript functions.
For example, I define three objects and name them person
, person1
, and person2
, and define a method named fullName
in the person object. Now I want to make the person1 object able to use it without having the fullName
method:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>This example calls the fullName method of person, using it on person1:
</p>
<p id="demo"></p>
<script>
var person = {
fullName: function() {
return this.firstName + " " + this.lastName;
}
}
var person1 = {
firstName:"Albro",
lastName: "Hive Blog"
}
var person2 = {
firstName:"OCDB",
lastName: "Hive Witness"
}
var x = person.fullName.call(person1);
document.getElementById("demo").innerHTML = x;
</script>
</body>
</html>
I can also run it on the person2
object by changing the call parameter to the following code:
person.fullName.call(person2);
So you can call a method on another object with the call()
statement!
[Hive: @albro]
https://inleo.io/threads/albro/re-leothreads-3cpkrcn7f
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Very nice post, I just joined HIVE but am started some python tips. I use JS a lot too, so hope to follow your posts. THanKS!
welcome!😘 I look forward to your posts, comments and ideas. Good luck👍
Thanks albro, and me as well1
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.