JavaScript Events & Tricks By albro
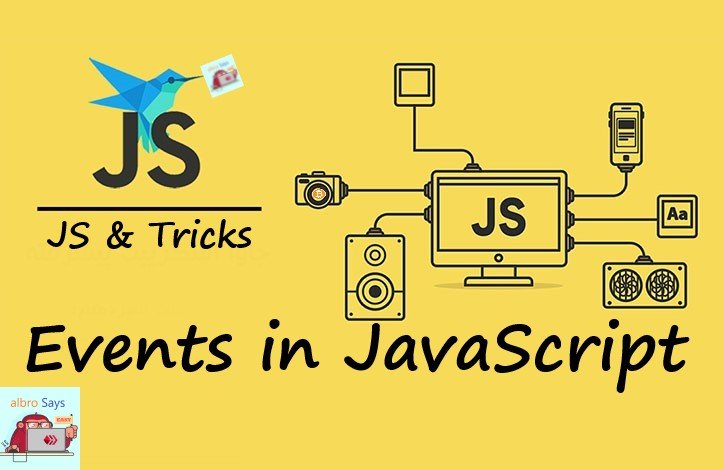
Events in JavaScript give us a lot of power to make pages dynamic. The way events work is that we can write JavaScript codes and set them so that if a certain event (ie an event) is done on the page, those codes will also be executed.
One of these events can be clicking on an HTML element. If we want to write code for such a situation, we say:
onclick=JavaScript
And instead of JavaScript, we put our JavaScript codes.
Let me tell you some examples of events:
- Clicking with the mouse on a specific object
- When the page is finished loading
- When an image is loaded
- When we move the mouse (without clicking) on a specific element
- When the input data (forms field) changes
- When the HTML form is registered and submitted
- When a key is pressed on the keyboard
- And ....
For example, in the following code, the <h1>
element changes after the user clicks:
<!DOCTYPE html>
<html>
<body>
<h1 onclick="this.innerHTML='Ooops!'">Click on this text!</h1>
</body>
</html>
Of course, we can write this code in another way and instead of adding the code directly to onclick
, give it to a function:
<!DOCTYPE html>
<html>
<body>
<h1 onclick="changeText(this)">Click on this text!</h1>
<script>
function changeText(id) {
id.innerHTML = "Ooops!";
}
</script>
</body>
</html>
How to use events
There are several ways to use an event. The first method is to add an attribute specific to that event to the element. See the following example:
<!DOCTYPE html>
<html>
<body>
<p>Click the button to display the date.</p>
<button onclick="displayDate()">The time is?</button>
<script>
function displayDate() {
document.getElementById("demo").innerHTML = Date();
}
</script>
<p id="demo"></p>
</body>
</html>
In this example, we have a function named displayDate
that will be executed when the button is clicked.
Another way to add events is to use JavaScript. Example:
<!DOCTYPE html>
<html>
<body>
<p>Click "Try it" to execute the displayDate() function.</p>
<button id="myBtn">Try it</button>
<p id="demo"></p>
<script>
document.getElementById("myBtn").onclick = displayDate;
function displayDate() {
document.getElementById("demo").innerHTML = Date();
}
</script>
</body>
</html>
First, we have received the element with the getElementById
function and then defined an event for it with the onclick
method. Now, whenever it is clicked, the displayDate
function will be executed.
Other events
As I said, the onclick
event is not the only JavaScript event, and the onload
(meaning "after loading") and onunload
(meaning after leaving the page) events are also considered among the events. These events are executed when the user enters or exits the page. For example, when the user enters our site, we can use onload
to provide the best version of the site based on the type and version of the user's browser. Also, as another use, we can use them to work with cookies. For example, as soon as you enter the page, the following code determines whether your browser cookies are enabled or not:
<!DOCTYPE html>
<html>
<body onload="checkCookies()">
<p id="demo"></p>
<script>
function checkCookies() {
var text = "";
if (navigator.cookieEnabled == true) {
text = "Cookies are enabled.";
} else {
text = "Cookies are not enabled.";
}
document.getElementById("demo").innerHTML = text;
}
</script>
</body>
</html>
Another famous event in JavaScript is the onchange
event. This event is mostly used to validate forms. I have given you one of its simple examples; When the user changes the content of the input field, the upperCase()
function is called:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction() {
var x = document.getElementById("fname");
x.value = x.value.toUpperCase();
}
</script>
</head>
<body>
Enter your name: <input type="text" id="fname" onchange="myFunction()">
<p>When you write your name inside the form and then click somewhere outside the form, a function is called that converts the typed letters into uppercase letters.</p>
</body>
</html>
Another famous HTML event is the onmouseover
(when the mouse moves over it) and onmouseout
(when the mouse moves away from the element) events.
Consider the following interesting example:
<!DOCTYPE html>
<html>
<body>
<div onmouseover="mOver(this)" onmouseout="mOut(this)"
style="background-color:#D94A38;width:120px;height:20px;padding:40px;">
Move the mouse over me
</div>
<script>
function mOver(obj) {
obj.innerHTML = "Thank you for listening to me"
}
function mOut(obj) {
obj.innerHTML = "Move the mouse over me"
}
</script>
</body>
</html>
Three other very useful events are:
-
onmousedown
means "while holding the mouse click" -
onmouseup
means "after releasing the mouse click" -
onclick
means "after one click"
How these three work is as follows: each click consists of two parts; When we press the mouse button and when we release the mouse button. onmousedown
is executed when we press the mouse button, onmouseup
is executed when we release the mouse button, and when these two operations are finished, a click is complete, so now onclick
is executed.
Pay attention to the following example:
<!DOCTYPE html>
<html>
<body>
<div onmousedown="mDown(this)" onmouseup="mUp(this)"
style="background-color:#D94A38;width:90px;height:20px;padding:40px;">
click on me
</div>
<script>
function mDown(obj) {
obj.style.backgroundColor = "#1ec5e5";
obj.innerHTML = "let me go";
}
function mUp(obj) {
obj.style.backgroundColor="#D94A38";
obj.innerHTML="Thanks";
}
</script>
</body>
</html>
We can give more creative examples. For example, in the code below, we use the famous image of our lamp:
<!DOCTYPE html>
<html>
<head>
<script>
function lighton() {
document.getElementById('myimage').src = "https://files.peakd.com/file/peakd-hive/albro/245HyrB7yNcBwbYAQ3nyTDhu8yamfsnLZHCMKQ97MiZK18i47XNvUH4aGiJaScDUX3PjB.gif";
}
function lightoff() {
document.getElementById('myimage').src = "https://files.peakd.com/file/peakd-hive/albro/23zS7bZFpAECgiDRkg2fihquz5LZDo6kxUcyeBy7cj3M1kPQDPMrjSGzBhWv5YZmWAMng.gif";
}
</script>
</head>
<body>
<img id="myimage" onmousedown="lighton()" onmouseup="lightoff()" src="https://files.peakd.com/file/peakd-hive/albro/23zS7bZFpAECgiDRkg2fihquz5LZDo6kxUcyeBy7cj3M1kPQDPMrjSGzBhWv5YZmWAMng.gif" width="100" height="180" />
<p>Click mouse and hold down!</p>
</body>
</html>
In the example above, when you click on the light bulb and hold the click, the image of the light bulb will change to the image of another light bulb, so you can turn on the light bulb with one click! First, use the DOM and getElementById
function to find the element It is the same image of the lamp (img
tag), we take it. Then we set the onmousedown
and onmouseup
events for it and tell which image to display when each is executed.
I hope you enjoyed this episode and realized the magical power of JavaScript and events.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🏅 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team